python 每秒读取一次配置文件,根据读取配置文件更新另一个值
时间: 2024-12-25 22:31:23 浏览: 0
在Python中,你可以通过`watchdog`库来实时监控文件变化,并配合`configparser`或`json`等模块来读取和更新配置文件。以下是一个简单的示例,假设我们有一个名为`config.ini`的配置文件,每秒检查其内容并更新另一个变量:
```python
import configparser
import time
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
class ConfigHandler(FileSystemEventHandler):
def __init__(self, config_path):
self.config_path = config_path
self.last_config_value = None
def on_modified(self, event):
if event.src_path == self.config_path:
with open(self.config_path, 'r') as f:
parser = configparser.ConfigParser()
parser.read(self.config_path)
new_value = parser.get('section', 'key') # 根据实际配置文件结构获取值
if new_value != self.last_config_value:
self.update_value(new_value)
self.last_config_value = new_value
def update_value(self, value):
# 这里可以根据新读取的value更新另一个地方的值,例如数据库、缓存等
print(f"Config updated to {value}")
def main(config_path):
handler = ConfigHandler(config_path)
observer = Observer()
observer.schedule(handler, path=config_path, recursive=False)
observer.start()
try:
while True:
time.sleep(1) # 每秒检查一次
except KeyboardInterrupt:
observer.stop()
observer.join()
if __name__ == "__main__":
main('path/to/config.ini')
```
在这个例子中,`ConfigHandler`类负责监听配置文件的变化,并在修改时重新加载配置。`update_value`方法是你处理新值的地方,可以根据需要进行操作,比如存储在一个全局变量、字典或者其他数据结构中。
阅读全文
相关推荐
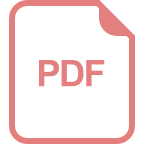
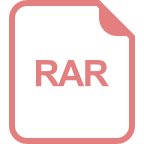
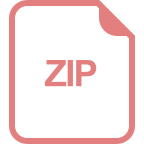
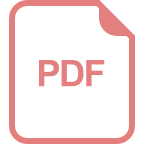
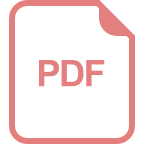
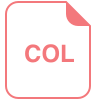
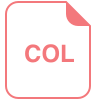
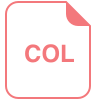
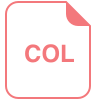
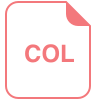
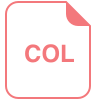
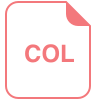
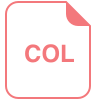
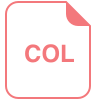
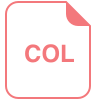



