MyBatis配置正确的TypeHandler来处理将时间戳变为字符串
时间: 2024-09-10 12:21:05 浏览: 84
在MyBatis中,TypeHandler是用于定义Java类型与数据库类型之间如何相互转换的处理器。如果你想要在查询数据库时将时间戳转换为字符串格式,或者在插入数据时将字符串格式的时间戳转换为数据库能够接受的格式,你可以自定义一个TypeHandler来实现这一功能。
下面是一个简单的自定义TypeHandler的例子,用于将数据库中的时间戳转换为指定格式的字符串:
```java
import org.apache.ibatis.type.JdbcType;
import org.apache.ibatis.type.MappedJdbcTypes;
import org.apache.ibatis.type.MappedTypes;
import java.sql.CallableStatement;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.text.SimpleDateFormat;
import java.util.Date;
@MappedTypes(String.class)
@MappedJdbcTypes(JdbcType.TIMESTAMP)
public class TimestampTypeHandler extends BaseTypeHandler<String> {
private SimpleDateFormat dateFormat;
public TimestampTypeHandler() {
dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
}
@Override
public void setParameter(PreparedStatement ps, int i, String parameter, JdbcType jdbcType) throws SQLException {
Date date = dateFormat.parse(parameter);
if (date != null) {
ps.setTimestamp(i, new java.sql.Timestamp(date.getTime()));
} else {
ps.setNull(i, JdbcType.TIMESTAMP);
}
}
@Override
public String getResult(ResultSet rs, String columnName) throws SQLException {
Date date = rs.getTimestamp(columnName);
return date != null ? dateFormat.format(date) : null;
}
@Override
public String getResult(ResultSet rs, int columnIndex) throws SQLException {
Date date = rs.getTimestamp(columnIndex);
return date != null ? dateFormat.format(date) : null;
}
@Override
public String getResult(CallableStatement cs, int columnIndex) throws SQLException {
Date date = cs.getTimestamp(columnIndex);
return date != null ? dateFormat.format(date) : null;
}
}
```
使用自定义的TypeHandler需要在MyBatis的配置文件中注册它,例如在`mybatis-config.xml`中添加:
```xml
<typeHandlers>
<typeHandler handler="com.example.TimestampTypeHandler" />
</typeHandlers>
```
或者在Mapper接口映射文件中指定使用这个TypeHandler:
```xml
<resultMap id="exampleResultMap" type="com.example.YourEntity">
<result property="dateProperty" column="date_column" javaType="String" jdbcType="TIMESTAMP" typeHandler="com.example.TimestampTypeHandler"/>
</resultMap>
```
确保替换`com.example.TimestampTypeHandler`和`com.example.YourEntity`为实际的包名和类名。
在使用自定义的TypeHandler时,确保你的数据库中时间戳字段的类型为TIMESTAMP或者能够接受相应的数据类型。
阅读全文
相关推荐
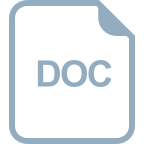
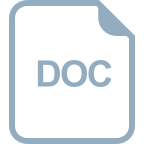
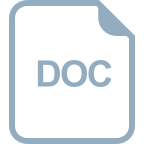
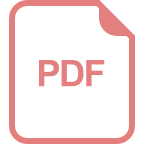
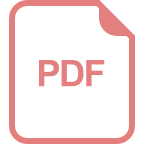
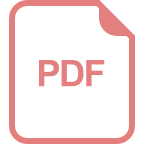
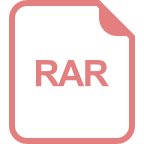
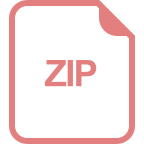
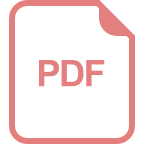
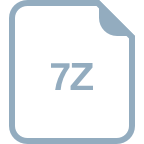
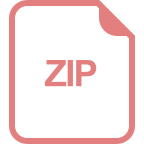
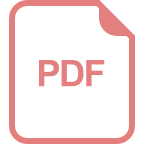
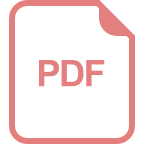
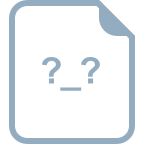
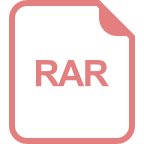
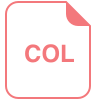
