设以不带头结点的循环链表表示队列,并且只设一个指针指向队尾结点,但不设头指针。编写相应的入队和出队程序。
时间: 2024-11-15 21:32:29 浏览: 22
要在不使用头节点的情况下,仅使用一个指针 `tail` 指向队尾来实现循环链表表示的队列,我们需要定义两个操作:
1. **入队(enqueue)**:当有新元素需要加入到队列时,我们将在当前队尾之后插入新的结点,并更新 `tail` 指针。
2. **出队(dequeue)**:如果队列非空,即 `tail` 不为 NULL,则我们将 `tail` 的下一个结点作为结果并更新 `tail` 为下一个结点,确保队列依然保持循环。
以下是一个简单的 C++ 实现:
```cpp
// 链表节点结构体
struct Node {
int data;
Node* next;
};
class QueueWithoutHead {
private:
Node* tail; // 只有一个指针指向队尾
public:
// 构造函数
QueueWithoutHead() : tail(nullptr) {}
// 入队操作
void enqueue(int value) {
if (tail == nullptr) { // 如果队列为空
Node* newNode = new Node();
newNode->data = value;
newNode->next = newNode; // 创建环形链表
tail = newNode;
} else {
Node* newNode = new Node();
newNode->data = value;
newNode->next = tail->next;
tail->next = newNode;
tail = newNode;
}
}
// 出队操作(假定队列非空)
int dequeue() {
if (tail == nullptr) return -1; // 返回错误值,表示队列已空
int result = tail->data; // 获取并存储数据
Node* temp = tail->next;
delete tail; // 删除旧结点
tail = temp; // 更新尾指针
return result;
}
};
```
在这个实现中,需要注意的是:
1. 当队列第一次入队时(即 `tail` 为 NULL),新创建的结点形成一个闭合的环形链表。
2. 出队操作前需要检查 `tail` 是否为空,因为不能从空队列删除元素。
3. 出队后要记得释放被移除的结点内存。
阅读全文
相关推荐
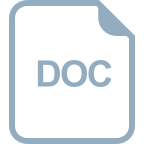
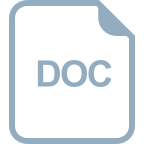
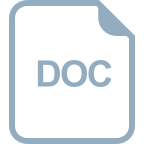









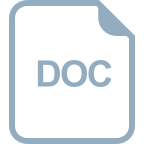
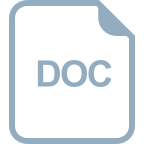
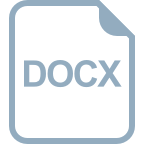
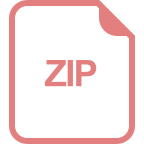