Spring AOP常用方法
时间: 2024-02-26 15:49:55 浏览: 85
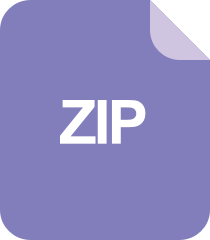
Spring AOP运用Spring AOP技术,要求包含前置通知、后置通知、环绕通知、返回通知、异常返回通知。
Spring AOP常用方法有以下几种:
1. 使用注解方式实现AOP:通过在目标方法上添加注解,然后在配置文件中配置切面和通知,实现对目标方法的增强。
```java
@Aspect
@Component
public class MyAspect {
@Before("execution(* com.example.service.UserService.addUser(..))")
public void beforeAddUser(JoinPoint joinPoint) {
// 在目标方法执行之前执行的逻辑
System.out.println("Before adding user...");
}
}
```
2. 使用XML配置方式实现AOP:通过在配置文件中配置切面和通知,实现对目标方法的增强。
```xml
<bean id="myAspect" class="com.example.aspect.MyAspect" />
<aop:config>
<aop:aspect ref="myAspect">
<aop:before method="beforeAddUser" pointcut="execution(* com.example.service.UserService.addUser(..))" />
</aop:aspect>
</aop:config>
```
3. 使用@Around注解实现环绕通知:通过在切面方法上添加@Around注解,可以在目标方法执行前后执行自定义逻辑。
```java
@Aspect
@Component
public class MyAspect {
@Around("execution(* com.example.service.UserService.addUser(..))")
public Object aroundAddUser(ProceedingJoinPoint joinPoint) throws Throwable {
// 在目标方法执行之前执行的逻辑
System.out.println("Before adding user...");
// 执行目标方法
Object result = joinPoint.proceed();
// 在目标方法执行之后执行的逻辑
System.out.println("After adding user...");
return result;
}
}
```
4. 使用@AfterReturning注解实现后置通知:通过在切面方法上添加@AfterReturning注解,可以在目标方法执行后执行自定义逻辑。
```java
@Aspect
@Component
public class MyAspect {
@AfterReturning(pointcut = "execution(* com.example.service.UserService.addUser(..))", returning = "result")
public void afterReturningAddUser(JoinPoint joinPoint, Object result) {
// 在目标方法执行后执行的逻辑
System.out.println("After adding user...");
}
}
```
5. 使用@AfterThrowing注解实现异常通知:通过在切面方法上添加@AfterThrowing注解,可以在目标方法抛出异常时执行自定义逻辑。
```java
@Aspect
@Component
public class MyAspect {
@AfterThrowing(pointcut = "execution(* com.example.service.UserService.addUser(..))", throwing = "ex")
public void afterThrowingAddUser(JoinPoint joinPoint, Exception ex) {
// 在目标方法抛出异常时执行的逻辑
System.out.println("Exception occurred while adding user: " + ex.getMessage());
}
}
```
阅读全文
相关推荐
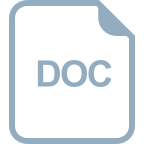
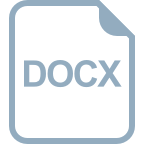
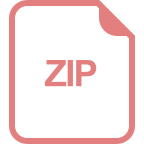
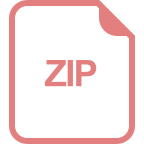
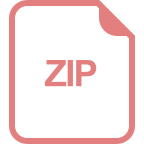
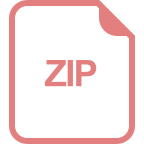
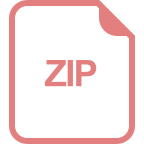
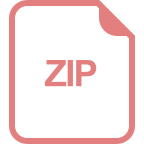
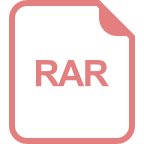
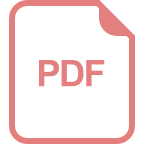
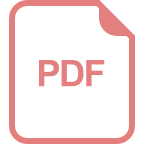
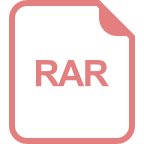
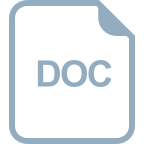
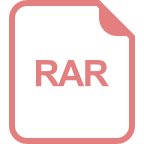
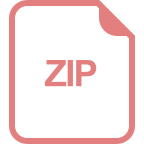
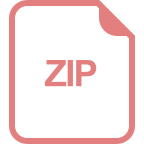
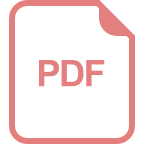