二叉树的基本操作及代码
时间: 2024-11-15 15:25:27 浏览: 6
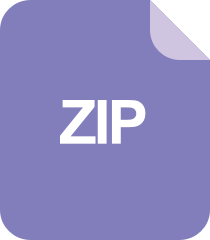
二叉树-基于C语言实现的二叉树基本操作.zip
二叉树的基本操作主要包括以下几个方面:
1. **创建和初始化**:
- 创建一个空的二叉树:`TreeNode root = null;`
- 插入节点:给定值创建一个新的节点并添加到适当的位置,如插入排序的二叉搜索树中 `root = insert(root, val)`。
2. **查找**:
- 遍历查找:前序、中序或后序遍历查找特定值,比如 `findValue(root, target)`.
- 递归查找:对于二叉搜索树,可以在 `isPresent(root, target)` 函数中使用递归来查找。
3. **删除**:
- 删除节点(复杂):涉及四种情况:未找到节点、只有一个子节点、有两个子节点(需要找到中序的后继或前驱)。具体实现视二叉树类型而定,例如 `deleteNode(root, target)`。
4. **遍历**:
- 前序遍历:`preOrderTraversal(root)`
- 中序遍历:`inOrderTraversal(root)`
- 后序遍历:`postOrderTraversal(root)`
- 层次遍历(广度优先搜索):`levelOrderTraversal(root)`
5. **其他**:
- 计算高度:`getHeight(root)`
- 检查是否平衡:判断二叉树左右子树高度差不超过1,如 `isBalanced(root)`
代码示例:
```java
public class TreeNode {
int val;
TreeNode left;
TreeNode right;
// constructor, getters and setters...
}
public TreeNode insert(TreeNode root, int val) {
if (root == null) {
return new TreeNode(val);
}
if (val < root.val) {
root.left = insert(root.left, val);
} else {
root.right = insert(root.right, val);
}
return root;
}
// 递归查找二叉搜索树中的值
public boolean isPresent(TreeNode root, int target) {
if (root == null || root.val == target) {
return root != null;
}
return root.val > target ? isPresent(root.left, target) : isPresent(root.right, target);
}
// 递归删除节点(仅简单示例)
public TreeNode deleteNode(TreeNode root, int key) {
if (root == null) return root;
if (key < root.val) root.left = deleteNode(root.left, key);
else if (key > root.val) root.right = deleteNode(root.right, key);
else { // key found
if (root.left == null && root.right == null) {
root = null;
} else if (root.left == null) {
TreeNode temp = root.right;
root = null;
return temp;
} else if (root.right == null) {
TreeNode temp = root.left;
root = null;
return temp;
}
TreeNode minNode = findMin(root.right); // find the minimum in right subtree
root.val = minNode.val;
root.right = deleteNode(root.right, minNode.val);
}
return root;
}
```
阅读全文
相关推荐
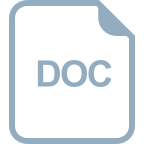
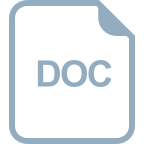
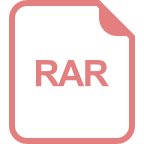
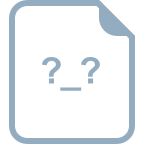
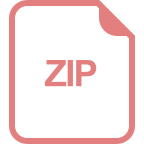
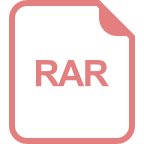
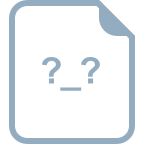
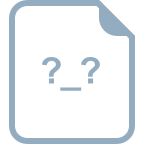
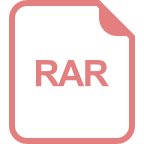
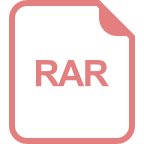
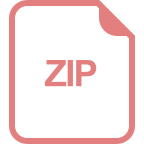
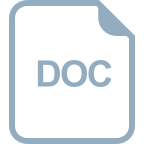
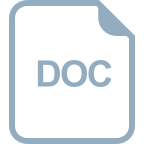
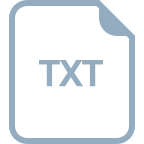
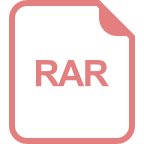
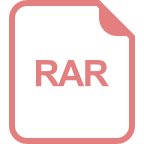