python不让用正则表达式批量替换
时间: 2024-06-14 22:02:46 浏览: 22
在Python中,如果你想要批量替换文本而不使用正则表达式,你可以使用`str.replace()`方法或者`re.sub()`函数配合列表推导式来进行替换操作。`str.replace()`方法适用于简单的字符串替换,而`re.sub()`则提供了更强大的正则表达式匹配功能,但如果你明确不希望使用正则,可以避免它。
这里是一个简单的例子,假设你想替换一个列表中的所有元素在字符串中的某个子串:
```python
# 假设你有一个字符串和一个替换列表
text = "Hello, World! This is a test string."
to_replace = ["World", "test"]
# 使用replace()方法逐个替换
for old in to_replace:
text = text.replace(old, "NewValue")
print(text) # 输出: "Hello, NewValue! This is a NewValue string."
```
如果你想替换特定位置的字符,可以直接用切片或字符串的`[]`运算符:
```python
# 例如替换每个单词的第一个字母为大写
words = text.split()
new_text = ' '.join(word.upper() + word[1:] for word in words)
print(new_text) # 输出: "Hello, NewValue! This is a NewValue string."
```
如果你有多个替换规则,并且不想硬编码它们,你可以创建一个字典来关联搜索值和替换值,然后遍历这个字典:
```python
replacement_dict = {"World": "Universe", "test": "trial"}
for old, new in replacement_dict.items():
text = text.replace(old, new)
print(text)
```
相关推荐
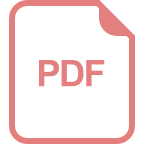
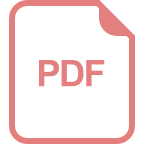
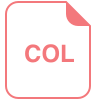
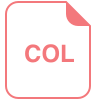
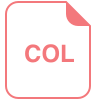
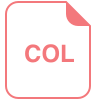
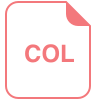









