c++ 线程池 条件变量
时间: 2023-11-14 17:05:53 浏览: 101
c线程池中条件变量的作用是实现多个线程之间的同步操作。当条件不满足时,相关线程会被阻塞,直到某种条件成立,这些线程才会被唤醒。条件变量通常与互斥锁一起使用,互斥锁用于保护共享资源,而条件变量用于在某个条件满足时通知等待在该条件上的线程。
在c线程池中,当线程池中的任务队列为空时,所有的工作线程都会进入等待状态。当有任务添加到任务队列时,线程池会通过发送信号或广播的方式通知等待的线程,使之解除阻塞并开始执行任务。
相关问题
C++ 线程池
线程池是一种多线程处理方式,它可以通过预先创建一定数量的线程,来处理多个任务。这样可以避免过多的线程创建和销毁开销,提高程序性能和稳定性。以下是一个简单的 C++ 线程池实现示例:
```cpp
#include <iostream>
#include <thread>
#include <queue>
#include <mutex>
#include <condition_variable>
class ThreadPool {
public:
ThreadPool(int threads) : stop(false) {
for (int i = 0; i < threads; ++i) {
workers.emplace_back([this] {
for (;;) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(this->queue_mutex);
this->condition.wait(lock, [this] { return this->stop || !this->tasks.empty(); });
if (this->stop && this->tasks.empty())
return;
task = std::move(this->tasks.front());
this->tasks.pop();
}
task();
}
});
}
}
template<class F>
void enqueue(F &&f) {
{
std::unique_lock<std::mutex> lock(queue_mutex);
tasks.emplace(std::forward<F>(f));
}
condition.notify_one();
}
~ThreadPool() {
{
std::unique_lock<std::mutex> lock(queue_mutex);
stop = true;
}
condition.notify_all();
for (std::thread &worker: workers)
worker.join();
}
private:
std::vector<std::thread> workers;
std::queue<std::function<void()>> tasks;
std::mutex queue_mutex;
std::condition_variable condition;
bool stop;
};
```
在这个实现中,我们使用了一个 `std::queue` 来存储任务,使用了一个互斥锁 `std::mutex` 来保护队列,使用了一个条件变量 `std::condition_variable` 来实现线程等待和唤醒。通过 `enqueue` 方法向线程池中添加任务,通过 `stop` 标识来控制线程池的停止。
使用线程池的示例代码:
```cpp
ThreadPool pool(4);
std::vector<std::future<int>> results;
for (int i = 0; i < 8; ++i) {
results.emplace_back(
pool.enqueue([i] {
std::cout << "hello " << i << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
std::cout << "world " << i << std::endl;
return i * i;
})
);
}
for (std::future<int> &result: results)
std::cout << result.get() << ' ';
std::cout << std::endl;
```
在这个示例中,我们创建了一个线程池 `ThreadPool pool(4)`,然后向线程池中添加了 8 个任务。每个任务都是一个 lambda 表达式,其中包含了两个输出语句和一个返回值。通过 `std::future` 来获取每个任务的返回值。最后输出所有任务的返回值。
阅读全文
相关推荐
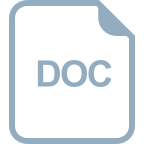
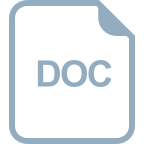
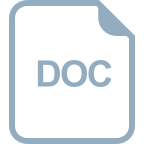
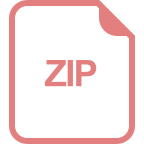
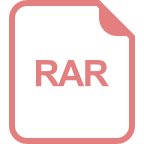
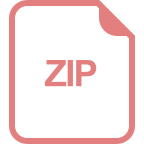
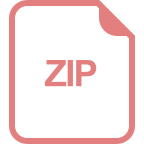
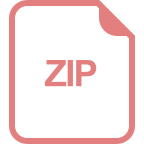
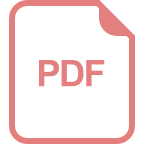
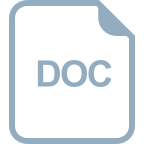
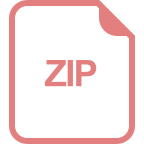
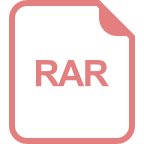
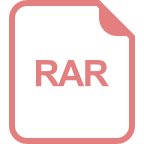
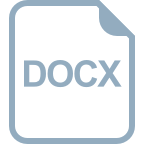
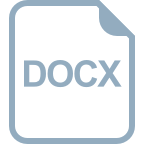
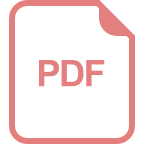
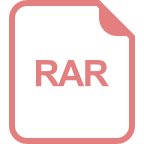
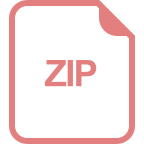