按快速排序原理实现排序。要求运行后控制台输入待排序数据,并显示快速排序过程中第一趟划分结果以及排序结果。请用C++代码输出
时间: 2024-10-13 17:07:39 浏览: 28
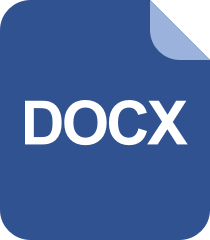
public class Aa.docx
以下是一个简单的C++程序,它实现了快速排序算法,并在每次分割后打印过程中的元素。注意,由于快速排序是递归的,所以这里仅展示了基本的分治思想,实际的完整代码会更复杂以处理边界情况和递归调用。
```cpp
#include <iostream>
#include <vector>
// 快速排序函数
void quickSort(std::vector<int>& arr, int low, int high) {
if (low < high) {
// 找到分区点
int pivotIndex = partition(arr, low, high);
// 对左右两个子数组进行递归排序
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
// 分区函数
int partition(std::vector<int>& arr, int low, int high) {
int pivot = arr[high]; // 选择最后一个元素作为基准
int i = low - 1;
for (int j = low; j < high; j++) {
// 如果当前元素小于或等于基准,交换并移动指针
if (arr[j] <= pivot) {
i++;
std::swap(arr[i], arr[j]);
}
}
// 将基准元素放到正确的位置(大于所有较小元素)
std::swap(arr[i + 1], arr[high]);
return i + 1;
}
// 主函数,接收用户输入并展示排序过程
int main() {
std::cout << "Enter a sequence of numbers to sort separated by space:\n";
std::string input;
std::getline(std::cin, input);
std::istringstream iss(input);
std::vector<int> nums;
int num;
while (iss >> num) {
nums.push_back(num);
}
quickSort(nums, 0, nums.size() - 1);
std::cout << "First pass after partitioning (pivot at end): ";
for (const auto& num : nums) {
std::cout << num << " ";
}
std::cout << "\nSorted array: ";
for (const auto& num : nums) {
std::cout << num << " ";
}
std::cout << "\n";
return 0;
}
```
阅读全文
相关推荐
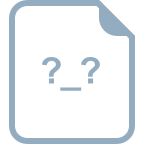
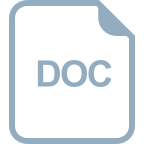





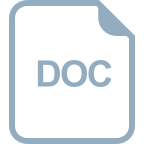
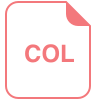
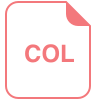
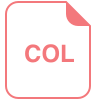
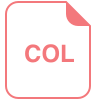
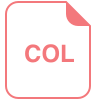
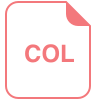
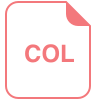
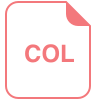
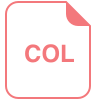
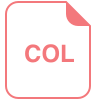