vue3 二维码生成新页面
时间: 2023-11-05 20:04:24 浏览: 55
关于在Vue3中生成二维码的新页面,你可以使用vue-qrcode库来实现。首先,你需要安装vue-qrcode库,可以通过以下命令进行安装:
```
npm install vue-qrcode --save
```
然后,在你的Vue组件中引入vue-qrcode库,并在模板中使用该组件来生成二维码。下面是一个示例代码:
```html
<template>
<div>
<vue-qrcode :text="url" :size="200"></vue-qrcode>
</div>
</template>
<script>
import { VueQRCode } from 'vue-qrcode'
export default {
components: {
VueQRCode
},
data() {
return {
url: 'https://example.com' // 需要转化成二维码的网址
}
}
}
</script>
```
在上面的代码中,你可以通过修改`url`属性来指定需要转化成二维码的网址。生成的二维码将会以200px的大小显示在页面上。
相关问题
vue 生成二维码并打印
要在Vue中生成二维码并打印,你可以使用qrcode.js和html2canvas库。下面是一个简单的实现示例:
1. 安装qrcode.js和html2canvas
```bash
npm install qrcodejs2 html2canvas --save
```
2. 在Vue组件中引入库
```javascript
import QRCode from 'qrcodejs2'
import html2canvas from 'html2canvas'
```
3. 在Vue组件中定义一个函数来生成二维码
```javascript
generateQRCode() {
const qrcode = new QRCode('qrcode', {
text: 'https://www.example.com', // 二维码内容
width: 256, // 二维码宽度
height: 256, // 二维码高度
colorDark : '#000000', // 二维码颜色
colorLight : '#ffffff', // 二维码背景色
correctLevel : QRCode.CorrectLevel.H // 二维码纠错级别
})
}
```
4. 在Vue组件中定义一个函数来打印页面
```javascript
print() {
html2canvas(document.body).then(canvas => {
const printWindow = window.open('', 'Print', 'height=600,width=800')
printWindow.document.write('<html><head><title>Print</title></head><body></body></html>')
printWindow.document.body.appendChild(canvas)
printWindow.document.close()
printWindow.print()
})
}
```
5. 在Vue组件中使用生成二维码和打印页面的函数
```vue
<template>
<div>
<div id="qrcode"></div>
<button @click="generateQRCode">生成二维码</button>
<button @click="print">打印页面</button>
</div>
</template>
<script>
import QRCode from 'qrcodejs2'
import html2canvas from 'html2canvas'
export default {
methods: {
generateQRCode() {
const qrcode = new QRCode('qrcode', {
text: 'https://www.example.com', // 二维码内容
width: 256, // 二维码宽度
height: 256, // 二维码高度
colorDark : '#000000', // 二维码颜色
colorLight : '#ffffff', // 二维码背景色
correctLevel : QRCode.CorrectLevel.H // 二维码纠错级别
})
},
print() {
html2canvas(document.body).then(canvas => {
const printWindow = window.open('', 'Print', 'height=600,width=800')
printWindow.document.write('<html><head><title>Print</title></head><body></body></html>')
printWindow.document.body.appendChild(canvas)
printWindow.document.close()
printWindow.print()
})
}
}
}
</script>
```
vue 点击分享随机生成二维码
Vue.js 是一款流行的 JavaScript 框架,它提供了丰富的组件和工具,用于开发交互性强的前端应用程序。要实现在Vue项目中点击分享随机生成二维码的功能,可以按以下步骤操作:
1. 在Vue项目中安装一个支持生成二维码的库,比如`qrcode`。可以使用npm进行安装,命令如下:`npm install qrcode`
2. 在需要生成二维码的组件中引入`qrcode`库,可以使用`import`语句进行引入:`import QRCode from 'qrcode'`
3. 在组件的`methods`中定义一个方法,用于生成二维码。方法中可以使用`qrcode`库的`toDataURL`方法生成二维码的base64格式数据。
4. 在模板中添加一个按钮或者其他交互元素,用于触发生成二维码的方法。可以使用`@click`指令绑定点击事件。
5. 在方法中使用`QRCode.toDataURL`方法生成二维码的base64格式数据,并将数据绑定到Vue实例的数据属性中。
6. 在模板中使用`<img>`标签显示生成的二维码。可以使用Vue的数据绑定将二维码的base64数据绑定到`src`属性。
例如,可以在Vue组件中按照以下方式实现生成二维码的功能:
```javascript
<template>
<div>
<button @click="generateQRCode">生成二维码</button>
<img :src="QRCodeData" alt="二维码">
</div>
</template>
<script>
import QRCode from 'qrcode';
export default {
data() {
return {
QRCodeData: '',
};
},
methods: {
generateQRCode() {
QRCode.toDataURL('https://example.com', (err, url) => {
if (err) {
console.error(err);
return;
}
this.QRCodeData = url;
});
},
},
};
</script>
```
上述代码中,点击按钮会调用`generateQRCode`方法,在方法中使用`QRCode.toDataURL`生成二维码的base64数据,并将数据绑定到`QRCodeData`属性上,在模板中使用`<img>`标签显示二维码。
这样,当点击分享按钮时,会随机生成一个二维码并显示在页面上。每次点击生成二维码的按钮,都会生成一个新的二维码。
相关推荐
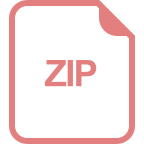











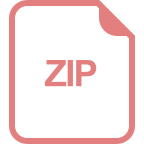