qt tftp 下载
时间: 2023-10-04 10:07:45 浏览: 76
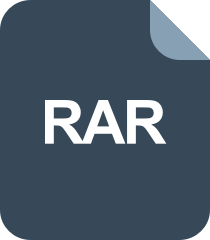
QT FTP下载

您好!对于在Qt中进行TFTP下载,您可以使用Qt的网络模块来实现。下面是一个简单的示例代码,可以用来下载文件:
```cpp
#include <QFile>
#include <QFileInfo>
#include <QNetworkAccessManager>
#include <QNetworkReply>
#include <QUrl>
class TftpDownloader : public QObject
{
Q_OBJECT
public:
TftpDownloader(QObject *parent = nullptr) : QObject(parent)
{
connect(&m_manager, &QNetworkAccessManager::finished, this, &TftpDownloader::onReplyFinished);
}
void downloadFile(const QUrl &url, const QString &savePath)
{
QNetworkRequest request(url);
m_reply = m_manager.get(request);
m_savePath = savePath;
QFile file(m_savePath);
file.open(QIODevice::WriteOnly);
connect(m_reply, &QIODevice::readyRead, this, &TftpDownloader::onReadyRead);
connect(m_reply, &QNetworkReply::downloadProgress, this, &TftpDownloader::onDownloadProgress);
}
private slots:
void onReplyFinished(QNetworkReply *reply)
{
if (reply->error() == QNetworkReply::NoError) {
QFile file(m_savePath);
file.close();
qDebug() << "File saved:" << m_savePath;
} else {
qDebug() << "Error occurred:" << reply->errorString();
}
reply->deleteLater();
}
void onReadyRead()
{
QFile file(m_savePath);
if (file.isOpen() && file.isWritable()) {
file.write(m_reply->readAll());
}
}
void onDownloadProgress(qint64 bytesReceived, qint64 bytesTotal)
{
qDebug() << "Downloaded" << bytesReceived << "bytes out of" << bytesTotal;
}
private:
QNetworkAccessManager m_manager;
QNetworkReply *m_reply = nullptr;
QString m_savePath;
};
```
您可以通过以下方式使用上述代码:
```cpp
TftpDownloader downloader;
QUrl url("tftp://example.com/path/to/file.txt");
QString savePath = "save/path/file.txt";
downloader.downloadFile(url, savePath);
```
请注意,这仅是一个简单的示例,并且不包含错误处理。您可能需要根据您的具体需求进行适当的修改。
希望能帮到您!如果有任何进一步的问题,请随时提问。
阅读全文
相关推荐
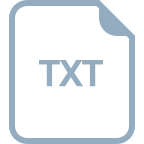

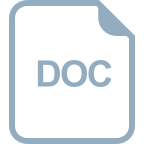
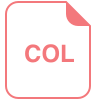





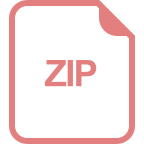
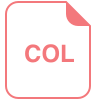
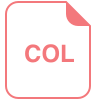
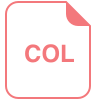
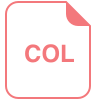
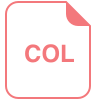
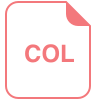
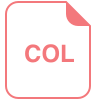