凯撒密码的破译c++
时间: 2024-09-23 13:08:46 浏览: 38
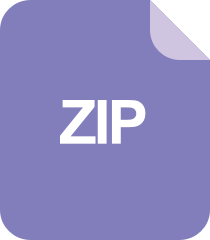
凯撒密码C++的实现
凯撒密码是一种简单的替换加密方法,它通过将字母表中的每个字符按照固定的位移(比如3位)进行循环移动来进行加密。在C++中,破译凯撒密码通常涉及以下几个步骤:
1. **接收密文**:首先需要获取用户输入的密文字符串。
2. **确定偏移量**:通常假设偏移量是一个已知值,可能是1到25之间的数字,对应字母表中的位置变化。如果是不知道偏移量的情况,可以尝试所有可能的偏移来解密。
3. **创建解密函数**:编写一个函数,接受密文、偏移量作为参数,遍历密文中的每一个字符。对于每个字母,检查其是否位于英文小写字母范围内(a-z),然后按字母顺序向左移动偏移量的位置,如果超出范围则回绕到开头。
4. **解码过程**:对密文中的每个字符应用上述解密函数,生成明文。
5. **显示结果**:将解密后的文本输出给用户。
以下是一个简单的C++代码示例:
```cpp
#include <iostream>
#include <string>
char caesarDecrypt(char c, int shift) {
if ('a' <= c && c <= 'z') {
return static_cast<char>((c - 'a' - shift + 26) % 26 + 'a');
} else if ('A' <= c && c <= 'Z') {
return static_cast<char>((c - 'A' - shift + 26) % 26 + 'A');
}
return c; // 对于非字母字符不做处理
}
std::string decryptCaesarCipher(const std::string& encrypted, int shift) {
std::string decrypted;
for (char c : encrypted) {
decrypted += caesarDecrypt(c, shift);
}
return decrypted;
}
int main() {
std::string encrypted = "Khoor Zruog"; // 示例密文
int shift = 3; // 假设偏移量为3
std::string decrypted = decryptCaesarCipher(encrypted, shift);
std::cout << "Decrypted message: " << decrypted << std::endl;
return 0;
}
```
阅读全文
相关推荐
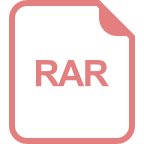
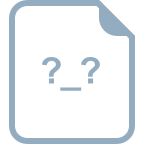



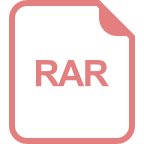
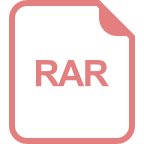



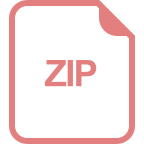
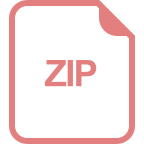
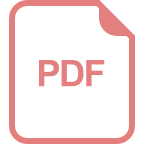
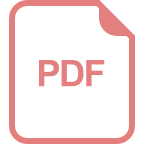
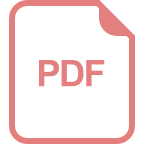