梯形法求二重积分 python
时间: 2025-01-06 09:32:03 浏览: 7
### 使用Python实现梯形法求解二重积分
为了利用梯形法则计算二重积分,可以采用嵌套的方式分别沿两个维度应用该方法。下面展示了一个具体的例子来说明这一过程。
#### 准备工作
首先确保安装了必要的科学计算库`numpy`用于创建测试数据集以及执行基本运算操作:
```bash
pip install numpy
```
接着定义被积函数$f(x,y)$作为示例,在实际应用场景中这应当替换为目标物理模型对应的表达式。
```python
import numpy as np
def integrand(x, y):
"""Example function to integrate."""
return (np.cos(np.pi * x)) ** 2 + (np.sin(2 * np.pi * y)) ** 2
```
#### 构建离散网格并初始化参数
设定积分区间$[a,b]\times[c,d]$内的采样点数目$n_x,n_y$以控制精度;构建$x-y$平面内均匀分布的节点集合$\{(x_i,y_j)\}$构成矩形单元格网状结构。
```python
# Define integration limits and number of points per axis.
nx, ny = 50, 75 # Number of sample points along each dimension
xa, xb = -1., 1. # Integration bounds on the X-axis
ya, yb = 0., 2. # Integration bounds on the Y-axis
# Create meshgrid from defined intervals.
X, Y = np.meshgrid(
np.linspace(xa, xb, nx),
np.linspace(ya, yb, ny))
```
#### 应用复合梯形公式
对于每一个内部结点$(i,j)$处的贡献值按照权重累加得到最终结果。注意边缘位置需特殊处理以免重复计入端点项。
```python
dx = (xb - xa)/(nx-1) # Step size between adjacent samples over X-dimension
dy = (yb - ya)/(ny-1) # Step size between adjacent samples over Y-dimension
integral_value = 0.
for i in range(nx): # Iterate through all rows...
for j in range(ny): # ...and columns within grid cells
weight_factor = 1
if i==0 or i==(nx-1):
weight_factor *= .5
if j==0 or j==(ny-1):
weight_factor *= .5
integral_value += dx*dy*weight_factor*integrand(X[i][j],Y[i][j])
print(f"The estimated value using trapezoidal rule is {integral_value:.8f}.")[^1]
```
上述代码片段实现了基于梯形公式的简单版本来进行数值上的近似估计。然而当面对更复杂的几何形状或是更高维空间中的问题时,则可能需要借助更加高效的算法比如Gauss-Legendre quadrature等替代方案[^3]。
阅读全文
相关推荐






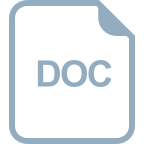












