opencv全景视频拼接
时间: 2023-11-09 21:03:35 浏览: 172
要实现opencv全景视频拼接,可以使用以下步骤:
1. 读取视频帧并提取特征点
2. 匹配特征点并计算变换矩阵
3. 将变换矩阵应用于图像并进行拼接
4. 重复步骤2和3直到所有帧都被拼接
以下是一个简单的示例代码:
```python
import cv2
import numpy as np
# 读取视频
cap = cv2.VideoCapture('video.mp4')
# 创建ORB特征检测器
orb = cv2.ORB_create()
# 读取第一帧并提取特征点
ret, prev_frame = cap.read()
prev_gray = cv2.cvtColor(prev_frame, cv2.COLOR_BGR2GRAY)
prev_keypoints, prev_descriptors = orb.detectAndCompute(prev_gray, None)
# 创建FLANN匹配器
matcher = cv2.DescriptorMatcher_create(cv2.DESCRIPTOR_MATCHER_BRUTEFORCE_HAMMING)
# 创建拼接器
stitcher = cv2.createStitcher() if cv2.__version__.startswith('3') else cv2.Stitcher_create()
# 循环读取视频帧并拼接
while True:
# 读取下一帧
ret, next_frame = cap.read()
if not ret:
break
next_gray = cv2.cvtColor(next_frame, cv2.COLOR_BGR2GRAY)
# 提取特征点并匹配
next_keypoints, next_descriptors = orb.detectAndCompute(next_gray, None)
matches = matcher.match(prev_descriptors, next_descriptors)
# 计算变换矩阵
src_pts = np.float32([prev_keypoints[m.queryIdx].pt for m in matches]).reshape(-1, 1, 2)
dst_pts = np.float32([next_keypoints[m.trainIdx].pt for m in matches]).reshape(-1, 1, 2)
M, _ = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 应用变换矩阵并拼接图像
result = cv2.warpPerspective(next_frame, M, (prev_frame.shape[1] + next_frame.shape[1], prev_frame.shape[0]))
result[0:prev_frame.shape[0], 0:prev_frame.shape[1]] = prev_frame
# 更新上一帧和特征点
prev_frame = result
prev_gray = cv2.cvtColor(prev_frame, cv2.COLOR_BGR2GRAY)
prev_keypoints, prev_descriptors = orb.detectAndCompute(prev_gray, None)
# 显示结果
cv2.imshow('Result', result)
cv2.waitKey()
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
阅读全文
相关推荐
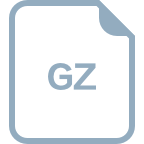













