java实验银行账户管理信息系统代码
时间: 2024-10-16 08:20:22 浏览: 38
在Java中设计一个简单的银行账户管理系统,我们通常会创建一个`Account`类作为基础类,然后通过继承和多态构建不同的账户类型,比如`SavingsAccount`和`CheckingAccount`。下面是一个基本的代码框架:
```java
// Account类(抽象类或接口)
public abstract class Account {
private String accountNumber;
private double balance;
// 构造函数、getter/setter等
// 抽象方法,如存款、取款操作
public abstract void deposit(double amount);
public abstract void withdraw(double amount);
}
// SavingsAccount类(子类)
public class SavingsAccount extends Account {
@Override
public void deposit(double amount) {
super.deposit(amount); // 调用父类方法先更新余额
// 具体储蓄相关的处理...
}
@Override
public void withdraw(double amount) {
if (amount <= balance) {
super.withdraw(amount); // 如果足够,更新余额
// 具体储蓄相关的处理...
} else {
System.out.println("Insufficient funds.");
}
}
}
// CheckingAccount类(另一个子类)
public class CheckingAccount extends Account {
// 检查账户特有的操作...
@Override
public void deposit(double amount) {
super.deposit(amount); // 更新余额
}
@Override
public void withdraw(double amount) {
// 检查转账限额等...
super.withdraw(amount);
}
}
// 主程序入口
public class BankManagementSystem {
public static void main(String[] args) {
Account savings = new SavingsAccount("123456", 1000);
Account checking = new CheckingAccount("789012", 500);
savings.deposit(500);
savings.withdraw(200);
checking.deposit(200);
checking.withdraw(100);
}
}
```
阅读全文
相关推荐
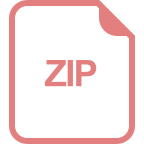
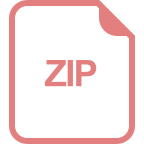
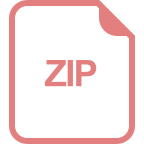
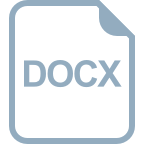
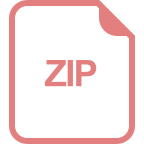
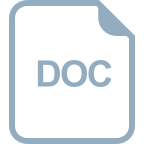
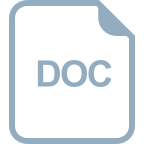
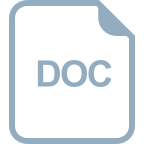
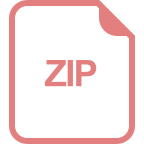
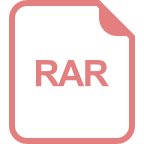
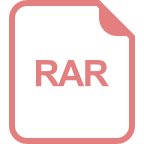
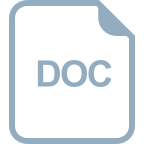
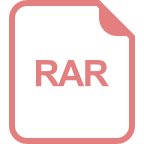
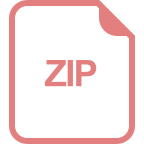
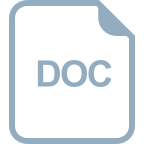
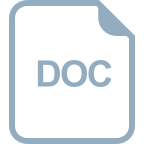
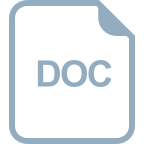

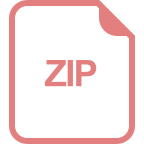