读取文件夹中txt文件
时间: 2024-12-26 15:17:08 浏览: 5
### 如何用编程语言读取指定文件夹下所有 txt 文件的内容
#### Python 实现方法
Python 提供了简单而强大的库用于操作文件系统。`os` 和 `glob` 是两个常用的模块,可以用来获取目录中的文件列表并过滤特定类型的文件。
```python
import os
import glob
def read_txt_files_from_directory(directory_path):
# 获取指定目录下的所有 .txt 文件路径
file_paths = glob.glob(os.path.join(directory_path, "*.txt"))
all_contents = {}
for path in file_paths:
with open(path, 'r', encoding='utf-8') as f:
content = f.read()
all_contents[path] = content
return all_contents
```
这段代码定义了一个函数 `read_txt_files_from_directory()` ,它接受一个参数作为目标文件夹的位置,并返回字典形式的结果集,其中键为各个 `.txt` 文件的绝对路径,值则对应于这些文件内的文本内容[^4]。
对于设置编码的部分:
```python
#!/usr/bin/python
#encoding=utf-8
```
这两行声明位于脚本顶部,指定了源码采用 UTF-8 编码保存以及解释器应使用的执行环境配置。这有助于确保程序能正确解析含有特殊字符的数据。
#### C++ 实现方案
在 Windows 平台上开发时,可以通过调用 WinAPI 来枚举给定路径下的子项。下面是一个简单的例子展示怎样利用 `_findfirst`, `_findnext` 函数来查找匹配模式(如 *.txt)的所有条目:
```cpp
#include <iostream>
#include <io.h> // _finddata_t struct and related functions
#include <string>
using namespace std;
void ReadTxtFilesInDirectory(const string& dirPath){
long hFile;
struct _finddata_t fileInfo;
string pName = dirPath + "\\*.txt";
if((hFile=_findfirst(pName.c_str(), &fileInfo)) != -1L){
do{
cout << "Found text file: " << fileInfo.name << endl;
ifstream infile(dirPath+"\\"+fileInfo.name);
stringstream buffer;
buffer<<infile.rdbuf();
cout<<"Content of "<<fileInfo.name<<":\n"<<buffer.str()<<endl;
}while(_findnext(hFile,&fileInfo)==0);
_findclose(hFile);
}
}
```
此段代码展示了如何通过迭代找到每一个符合条件 (即扩展名为`.txt`) 的项目,并打印其名称连同内部存储的信息到控制台窗口中去[^2]。
#### Java 实现方式
Java 中同样存在多种途径完成相同任务;这里给出一种基于 Files.walk() 流式处理的方式来进行递归扫描整个树形结构直至最底层节点为止。
```java
import java.io.IOException;
import java.nio.file.*;
import java.util.stream.Collectors;
public class TxtReader {
public static void main(String[] args) throws IOException {
Path startDir = Paths.get("your/path/here");
try (Stream<Path> stream = Files.find(startDir,
Integer.MAX_VALUE,
(path, attr) -> String.valueOf(path).endsWith(".txt"))) {
List<String> contents = stream.map(path -> {
try {
return new String(Files.readAllBytes(path));
} catch (IOException e) {
throw new RuntimeException(e);
}
}).collect(Collectors.toList());
System.out.println(contents);
}
}
}
```
上述片段实现了从起始位置向下逐层深入寻找满足条件 (`".txt"` 结尾) 的对象集合,并最终将其转换成字符串数组输出至标准输出流上显示出来[^5]。
阅读全文
相关推荐
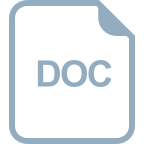
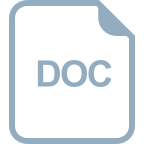
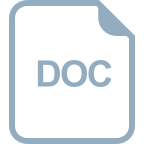















