使用标准i/o库设计一个程序,将文件a的内容复制到b中去使得b的内容与a中内容相同。将a中的内容追加到c文件中。 注意:需要考虑到a、 b、c不存在,b中有原始数据等
时间: 2024-10-13 18:14:51 浏览: 23
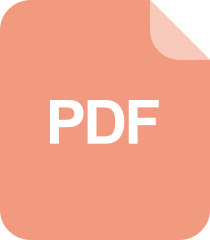
小班附加内容1
为了完成这个任务,你可以使用Python的标准`os`和`shutil`库来操作文件,以及`open()`函数读取和写入文本。这里是一个简单的示例程序:
```python
import os
# 检查文件是否存在
def check_file(file_path):
return os.path.exists(file_path)
# 将文件a的内容复制到b中
def copy_to_b(a_path, b_path):
if not check_file(b_path) or not check_file(a_path): # 如果b不存在或者为空
with open(a_path, 'r') as src_file, open(b_path, 'w') as dest_file:
dest_file.write(src_file.read())
print(f"已将 {a_path} 的内容复制到 {b_path}")
# 将a的内容追加到c文件中
def append_to_c(a_path, c_path):
if check_file(c_path): # 如果c存在,追加模式打开
with open(c_path, 'a') as dest_file:
with open(a_path, 'r') as src_file:
dest_file.write(src_file.read())
print(f"{a_path} 的内容已追加到 {c_path}")
else: # 如果c不存在,创建并写入
with open(c_path, 'w') as dest_file:
with open(a_path, 'r') as src_file:
dest_file.write(src_file.read())
print(f"{a_path} 的内容已直接写入 {c_path}")
# 调用函数
a_path = "input.txt"
b_path = "output.txt"
c_path = "append.txt"
copy_to_b(a_path, b_path)
append_to_c(a_path, c_path)
```
在这个程序中,我们首先检查每个文件是否存在。如果`b_path`不存在或者是一个空文件,我们就把`a_path`的内容完全写入`b_path`。然后,无论`c_path`是否存在,我们都将其内容追加到`c_path`。
阅读全文
相关推荐
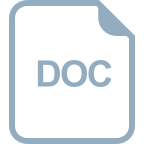
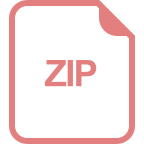

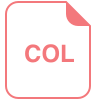
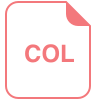
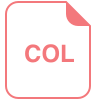
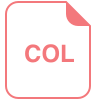
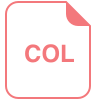
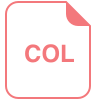
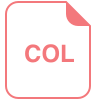
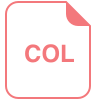
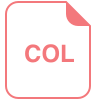
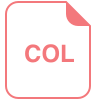
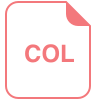
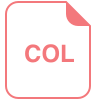
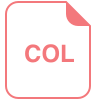
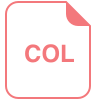
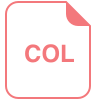