用c语言写给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出 和为目标值 target 的那 两个 整数,并返回它们的数组下标。 你可以假设每种输入只会对应一个答案,并且你不能使用两次相同的元素。 你可以按任意顺序返回答案。
时间: 2024-10-12 13:03:46 浏览: 32
这是一个典型的双指针问题,可以使用C语言解决。我们可以从数组的第一个元素开始,用一个指针i表示第一个元素,另一个指针j表示第二个元素。然后我们用一个循环来找到满足条件的两个数,即`nums[i] + nums[j] = target`。
以下是C语言的解决方案:
```c
#include <stdio.h>
#include <stdbool.h>
// 函数声明
bool findTwoSum(int* nums, int numsSize, int target);
int main() {
int nums[] = {2, 7, 11, 15};
int target = 9;
if (findTwoSum(nums, sizeof(nums) / sizeof(nums[0]), target)) {
int index1 = ...; // 第一个元素的索引
int index2 = ...; // 第二个元素的索引
printf("The two numbers are at indices %d and %d.\n", index1, index2);
} else {
printf("No such pair found.\n");
}
return 0;
}
// 查找并返回满足条件的两个数的下标
bool findTwoSum(int* nums, int numsSize, int target) {
for (int i = 0; i < numsSize - 1; i++) {
int complement = target - nums[i];
if (complement >= nums[i + 1] && complement >= 0) {
for (int j = i + 1; j < numsSize; j++) {
if (nums[j] == complement) {
return true;
}
}
}
}
return false;
}
阅读全文
相关推荐
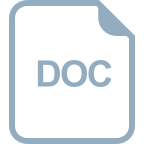
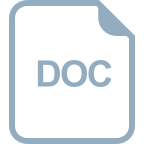
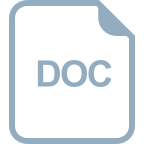















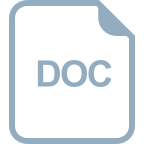