import requests from bs4 import BeautifulSoup import csv # 发送HTTP请求获取网页内容 url = "https://item.jd.com/100016034394.html" # 替换为目标商品评价页面的URL response = requests.get(url) html = response.text # 使用BeautifulSoup解析网页内容 soup = BeautifulSoup(html, "html.parser") # 获取所有评价信息 comments = soup.find_all("div", class_="comment-item") # 创建CSV文件并写入数据 filename = "商品评价.csv" with open(filename, "w", encoding="utf-8-sig", newline="") as csvfile: writer = csv.writer(csvfile) writer.writerow(["用户", "评分", "评价内容"]) # 写入表头 # 写入每条评价的数据 for comment in comments: user = comment.find("div", class_="user-info").find("span").text.strip() rating = comment.find("div", class_="comment-star").find("i")["class"][1].split("-")[1] content = comment.find("div", class_="comment-con").find("p").text.strip() writer.writerow([user, rating, content]) print(f"成功爬取并保存为 {filename}") HTTPSConnectionPool(host='www.jd.com', port=443): Max retries exceeded with url: /?from=pc_item_sd (Caused by ConnectTimeoutError(<urllib3.connection.HTTPSConnection object at 0x0000015FCC87E7C0>, 'Connection to www.jd.com timed out. (connect timeout=10)'))
时间: 2023-12-05 20:45:20 浏览: 470
这个错误是连接超时错误,可能是因为请求的网站响应时间过长或网络连接不稳定导致的。你可以尝试以下解决方案:
1. 增加请求超时时间:在发送请求时,你可以指定一个更长的超时时间来等待服务器响应。将`requests.get()`函数中的`timeout`参数设置为一个适当的值,例如`timeout=30`,表示等待30秒钟来建立连接和获取响应。
```python
response = requests.get(url, timeout=30)
```
2. 检查网络连接和目标网站的可访问性:确保你的网络连接正常,并且能够正常访问目标网站。你可以尝试在浏览器中打开目标网址以检查是否能够成功加载页面。
3. 使用代理服务器:如果你的网络环境有限制或目标网站对爬虫有限制,可以尝试使用代理服务器来发送请求。通过设置`requests.get()`函数中的`proxies`参数,指定代理服务器的地址和端口。
```python
proxies = {
'http': 'http://proxy.example.com:8080',
'https': 'http://proxy.example.com:8080',
}
response = requests.get(url, proxies=proxies)
```
4. 尝试其他网络环境:如果以上方法都无效,你可以尝试在其他网络环境下运行代码,例如使用不同的网络连接或切换到其他网络环境,以排除网络问题。
根据具体情况选择适合的解决方案,以确保能够成功建立连接并爬取目标网页的数据。
相关问题
import requests from bs4 import BeautifulSoup import csv # 发送HTTP请求获取网页内容 url = "https://item.jd.com/100016034394.html" # 替换为目标商品评价页面的URL response = requests.get(url) html = response.text # 使用BeautifulSoup解析网页内容 soup = BeautifulSoup(html, "html.parser") # 获取所有评价信息 comments = soup.find_all("div", class_="comment-item") # 创建CSV文件并写入数据 filename = "商品评价.csv" with open(filename, "w", encoding="utf-8-sig", newline="") as csvfile: writer = csv.writer(csvfile) writer.writerow(["用户", "评分", "评价内容"]) # 写入表头 # 写入每条评价的数据 for comment in comments: user = comment.find("div", class_="user-info").find("span").text.strip() rating = comment.find("div", class_="comment-star").find("i")["class"][1].split("-")[1] content = comment.find("div", class_="comment-con").find("p").text.strip() writer.writerow([user, rating, content]) print(f"成功爬取并保存为 {filename}") 上述代码加入用户代理内容怎么加
你可以在发送HTTP请求时,通过设置headers参数来添加用户代理内容。可以使用`User-Agent`字段来设置用户代理。以下是修改后的代码示例:
```python
import requests
from bs4 import BeautifulSoup
import csv
# 发送HTTP请求获取网页内容
url = "https://item.jd.com/100016034394.html" # 替换为目标商品评价页面的URL
# 设置用户代理
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/94.0.4606.81 Safari/537.36"
}
response = requests.get(url, headers=headers)
html = response.text
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(html, "html.parser")
# 获取所有评价信息
comments = soup.find_all("div", class_="comment-item")
# 创建CSV文件并写入数据
filename = "商品评价.csv"
with open(filename, "w", encoding="utf-8-sig", newline="") as csvfile:
writer = csv.writer(csvfile)
writer.writerow(["用户", "评分", "评价内容"]) # 写入表头
# 写入每条评价的数据
for comment in comments:
user = comment.find("div", class_="user-info").find("span").text.strip()
rating = comment.find("div", class_="comment-star").find("i")["class"][1].split("-")[1]
content = comment.find("div", class_="comment-con").find("p").text.strip()
writer.writerow([user, rating, content])
print(f"成功爬取并保存为 {filename}")
```
在上述代码中,我们在发送请求前设置了`headers`参数,并且将用户代理信息添加到其中。你可以根据需要修改`User-Agent`字段的值。
import requests from bs4 import BeautifulSoup import csv # 发送HTTP请求获取网页内容 url = "https://item.jd.com/100016034394.html" #设置用户代理 headers={'cookie':'over18=1', 'User-Agent':'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36 Edg/114.0.1823.82' } response = requests.get(url,headers=headers) html = response.text # 使用BeautifulSoup解析网页内容 soup = BeautifulSoup(html, "html.parser") # 获取所有评价信息 comments = soup.find_all("div", class_="comment-item") # 创建CSV文件并写入数据 filename = "商品评价.csv" with open(filename, "w", encoding="utf-8-sig", newline="") as csvfile: writer = csv.writer(csvfile) writer.writerow(["用户", "评分", "评价内容"]) # 写入表头 # 写入每条评价的数据 for comment in comments: user = comment.find("div", class_="user-info").find("span").text.strip() rating = comment.find("div", class_="comment-star").find("i")["class"][1].split("-")[1] content = comment.find("div", class_="comment-con").find("p").text.strip() writer.writerow([user, rating, content]) print(f"成功爬取并保存为 {filename}") 怎么改html,html格式是什么
要修改HTML内容,你可以使用BeautifulSoup提供的方法来操作解析后的HTML对象。以下是一些常用的操作:
1. 修改标签的文本内容:可以使用`tag.string`属性或`tag.string.replace_with(new_text)`方法来修改标签的文本内容。例如,`tag.string = "新的内容"`会将标签的文本内容替换为新的内容。
2. 修改标签的属性:可以使用`tag["属性名"] = "新的属性值"`来修改标签的属性值。例如,`tag["class"] = "new-class"`会将标签的class属性值修改为"new-class"。
3. 添加新的标签:可以使用`new_tag = soup.new_tag("标签名")`来创建一个新的标签对象,然后使用`tag.append(new_tag)`或`tag.insert(index, new_tag)`将新标签添加到指定位置。
4. 删除标签:可以使用`tag.decompose()`方法来删除一个标签及其内容。或者使用`tag.extract()`方法将标签从解析树中提取出来,但保留其内容。
关于HTML格式,HTML是一种标记语言,用于结构化表示网页内容。它由一系列标签和属性组成,用于描述网页的结构和样式。在爬取网页数据时,我们使用HTML解析库(如BeautifulSoup)将HTML文档解析成树状结构,然后可以通过操作解析后的HTML对象来提取和修改其中的数据。
在爬虫过程中,你可以通过查看网页源代码来了解具体的HTML格式,并根据需要来提取和修改特定的标签和属性。你可以使用浏览器的开发者工具(如Chrome的开发者工具)来查看网页源代码和调试HTML的修改效果。
相关推荐
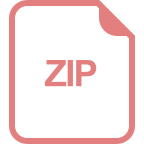
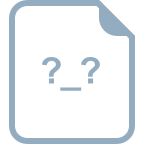










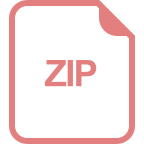
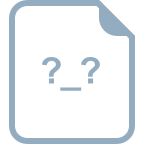