pygame用类实现贪吃蛇
时间: 2023-11-30 09:41:57 浏览: 98
以下是使用pygame和类实现贪吃蛇的示例代码:
```python
import pygame
import random
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义方向常量
UP = 1
DOWN = 2
LEFT = 3
RIGHT = 4
# 定义方块大小和速度
BLOCK_SIZE = 20
SPEED = 20
# 定义游戏窗口大小
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
# 初始化pygame
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('贪吃蛇')
# 定义蛇类
class Snake:
def __init__(self):
self.body = [(WINDOW_WIDTH / 2, WINDOW_HEIGHT / 2)]
self.direction = random.choice([UP, DOWN, LEFT, RIGHT])
# 获取蛇头位置
def get_head(self):
return self.body[0]
# 获取蛇尾位置
def get_tail(self):
return self.body[-1]
# 移动蛇
def move(self):
head = self.get_head()
if self.direction == UP:
new_head = (head[0], head[1] - BLOCK_SIZE)
elif self.direction == DOWN:
new_head = (head[0], head[1] + BLOCK_SIZE)
elif self.direction == LEFT:
new_head = (head[0] - BLOCK_SIZE, head[1])
elif self.direction == RIGHT:
new_head = (head[0] + BLOCK_SIZE, head[1])
self.body.insert(0, new_head)
self.body.pop()
# 改变方向
def change_direction(self, direction):
if direction == UP and self.direction != DOWN:
self.direction = UP
elif direction == DOWN and self.direction != UP:
self.direction = DOWN
elif direction == LEFT and self.direction != RIGHT:
self.direction = LEFT
elif direction == RIGHT and self.direction != LEFT:
self.direction = RIGHT
# 增加长度
def add_length(self):
tail = self.get_tail()
if self.direction == UP:
new_tail = (tail[0], tail[1] + BLOCK_SIZE)
elif self.direction == DOWN:
new_tail = (tail[0], tail[1] - BLOCK_SIZE)
elif self.direction == LEFT:
new_tail = (tail[0] + BLOCK_SIZE, tail[1])
elif self.direction == RIGHT:
new_tail = (tail[0] - BLOCK_SIZE, tail[1])
self.body.append(new_tail)
# 检查是否撞墙
def check_wall_collision(self):
head = self.get_head()
if head[0] < 0 or head[0] >= WINDOW_WIDTH or head[1] < 0 or head[1] >= WINDOW_HEIGHT:
return True
return False
# 检查是否撞到自己
def check_self_collision(self):
head = self.get_head()
for i in range(1, len(self.body)):
if head == self.body[i]:
return True
return False
# 定义食物类
class Food:
def __init__(self):
self.position = self.generate_position()
# 生成随机位置
def generate_position(self):
x = random.randint(0, WINDOW_WIDTH // BLOCK_SIZE - 1) * BLOCK_SIZE
y = random.randint(0, WINDOW_HEIGHT // BLOCK_SIZE - 1) * BLOCK_SIZE
return (x, y)
# 绘制食物
def draw(self):
pygame.draw.rect(screen, RED, (self.position[0], self.position[1], BLOCK_SIZE, BLOCK_SIZE))
# 定义游戏类
class Game:
def __init__(self):
self.snake = Snake()
self.food = Food()
self.score = 0
# 处理事件
def handle_events(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP or event.key == pygame.K_w:
self.snake.change_direction(UP)
elif event.key == pygame.K_DOWN or event.key == pygame.K_s:
self.snake.change_direction(DOWN)
elif event.key == pygame.K_LEFT or event.key == pygame.K_a:
self.snake.change_direction(LEFT)
elif event.key == pygame.K_RIGHT or event.key == pygame.K_d:
self.snake.change_direction(RIGHT)
# 更新游戏
def update(self):
self.snake.move()
if self.snake.check_wall_collision() or self.snake.check_self_collision():
self.game_over()
if self.snake.get_head() == self.food.position:
self.snake.add_length()
self.food = Food()
self.score += 10
# 绘制游戏
def draw(self):
screen.fill(BLACK)
self.snake.draw()
self.food.draw()
self.draw_score()
pygame.display.update()
# 绘制分数
def draw_score(self):
font = pygame.font.SysFont(None, 25)
text = font.render('Score: ' + str(self.score), True, WHITE)
screen.blit(text, (10, 10))
# 游戏结束
def game_over(self):
font = pygame.font.SysFont(None, 50)
text = font.render('Game Over', True, WHITE)
screen.blit(text, (WINDOW_WIDTH / 2 - text.get_width() / 2, WINDOW_HEIGHT / 2 - text.get_height() / 2))
pygame.display.update()
pygame.time.wait(2000)
self.__init__()
# 创建游戏对象
game = Game()
# 游戏循环
while True:
game.handle_events()
game.update()
game.draw()
pygame.time.wait(SPEED)
```
阅读全文
相关推荐

















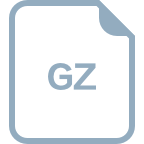