java导出url文件
时间: 2024-09-18 18:03:49 浏览: 36
在Java中,如果你想要将数据(如文本、图片、PDF等)作为URL内容导出到本地文件,你可以使用`java.net.URL`类配合`java.io.InputStream`和`java.nio.file`包。下面是一个简单的示例,展示如何下载URL的内容并保存为文件:
```java
import java.io.*;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class URLToFile {
public static void downloadUrlAndSave(String urlString, String destinationPath) throws IOException {
try (InputStream in = new URL(urlString).openStream();
OutputStream out = new FileOutputStream(destinationPath)) {
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
System.out.println("Download complete! File saved at " + destinationPath);
} catch (MalformedURLException e) {
System.err.println("Invalid URL: " + urlString);
}
}
public static void main(String[] args) {
String url = "https://example.com/file-to-download";
String filePath = "/path/to/save/downloaded-file.txt"; // 替换为你需要保存的本地路径
try {
downloadUrlAndSave(url, filePath);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们首先创建一个`URL`对象,然后使用`openStream()`方法获取输入流,接着读取输入流的字节并写入到指定的输出文件。最后,处理可能出现的异常。
阅读全文
相关推荐
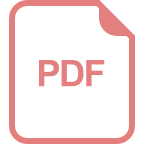
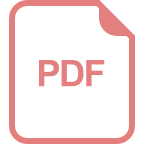
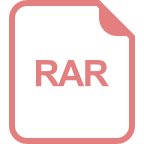
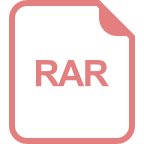
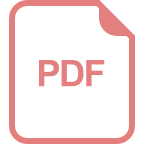
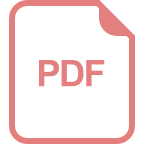
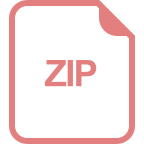
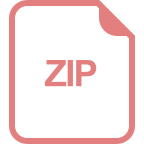
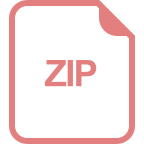
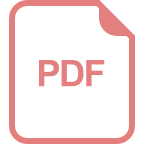
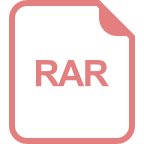
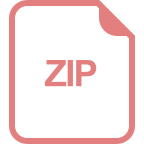
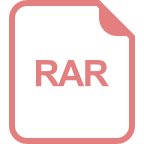
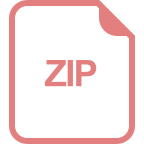
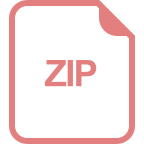
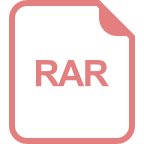
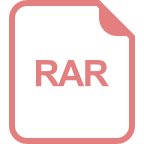

