用java通过sftp在服务器之间传输文件
时间: 2024-10-16 09:15:35 浏览: 27
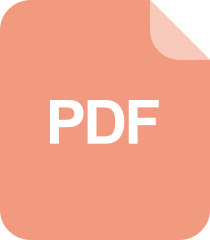
如何在java中使用SFTP协议安全的传输文件
在Java中,使用SFTP (Secure File Transfer Protocol) 进行服务器之间的文件传输通常会依赖于第三方库,如JSch (Java Secure Channel) 或者 Apache Commons VFS2。下面是使用JSch库的一个基本示例:
首先,你需要在项目中添加JSch的依赖。如果你的项目是Maven管理的,可以在pom.xml中添加:
```xml
<dependency>
<groupId>com.jcraft</groupId>
<artifactId>jsch</artifactId>
<version>0.1.55</version>
</dependency>
```
然后,你可以创建一个简单的SFTP操作类:
```java
import com.jcraft.jsch.ChannelSftp;
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.Session;
public class SftpFileTransfer {
private static final String USER = "your_username";
private static final String PASS = "your_password";
private static final String HOST = "remote_host";
private static final int PORT = 22; // default for SSH
public void transferFile(String localFilePath, String remoteFilePath) throws Exception {
JSch jsch = new JSch();
Session session = jsch.getSession(USER, HOST, PORT);
session.setPassword(PASS);
session.connect(); // Establish the connection
try (ChannelSftp channel = (ChannelSftp) session.openChannel("sftp")) {
channel.connect();
if (!channel.ls(remoteFilePath).isEmpty()) {
System.out.println("Remote file already exists");
} else {
channel.put(localFilePath, remoteFilePath); // Upload from local to remote
System.out.println("File uploaded successfully");
}
channel.disconnect(); // Close the sftp connection after transfer
}
session.disconnect(); // Disconnect from the server
}
}
```
在这个例子中,`transferFile`方法接收本地文件路径和远程文件路径作为参数,尝试连接到远程服务器、建立SFTP通道,并将文件从本地上传到指定的位置。
阅读全文
相关推荐
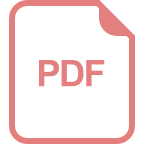
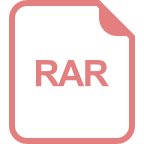


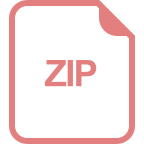
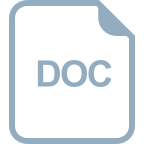
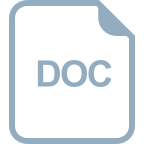
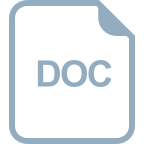
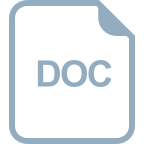
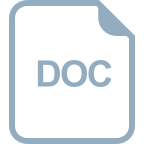


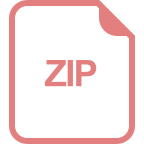
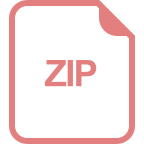
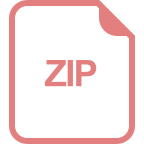
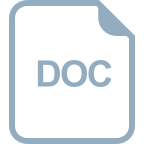
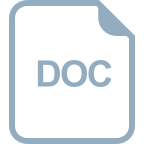
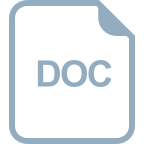
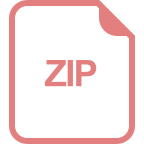