python实现实时监控一个文件夹中的内容,如果有新文件生成,将新文件上传到接口http://192.168.1.226:6060/dz接收
时间: 2024-01-28 07:05:15 浏览: 167
要实现实时监控一个文件夹中的内容,并在有新文件生成时将新文件上传到指定的接口,你可以使用 Python 的 watchdog 库来实现。下面是一个示例代码,演示了如何实现这个功能:
```python
import time
import requests
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
# 监控文件夹路径和上传接口
watch_folder = '/path/to/watch/folder'
upload_url = 'http://192.168.1.226:6060/dz'
class FileEventHandler(FileSystemEventHandler):
def on_created(self, event):
if not event.is_directory:
# 获取新创建的文件路径
file_path = event.src_path
# 发送文件到上传接口
with open(file_path, 'rb') as file:
files = {'file': file}
response = requests.post(upload_url, files=files)
# 打印上传结果
print(f"Uploaded {file_path}: {response.status_code}")
if __name__ == "__main__":
# 创建监控器和事件处理器
event_handler = FileEventHandler()
observer = Observer()
# 添加监控路径和事件处理器
observer.schedule(event_handler, watch_folder, recursive=True)
# 启动监控器
observer.start()
try:
while True:
time.sleep(1)
except KeyboardInterrupt:
observer.stop()
observer.join()
```
在代码中,你需要将 `watch_folder` 设置为要监控的文件夹的路径,并将 `upload_url` 设置为要上传文件的接口地址。
然后,我们创建一个 `FileEventHandler` 类,继承自 `FileSystemEventHandler`,并重写了 `on_created` 方法。当有新文件创建时,该方法会被触发,我们在其中将新文件上传到指定的接口。
在 `main` 函数中,我们创建了一个 `Observer` 对象和一个 `FileEventHandler` 对象,并将事件处理器添加到监控器中。然后,启动监控器并进入主循环,持续监控文件夹中的内容。
请注意,你需要安装 watchdog 和 requests 库,可以使用以下命令进行安装:
```
pip install watchdog requests
```
希望这个示例代码能满足你的需求!如果有任何问题,请随时提问。
阅读全文
相关推荐


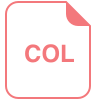
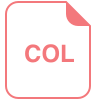
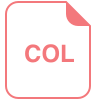
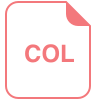
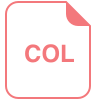
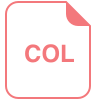
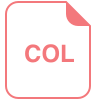
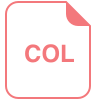
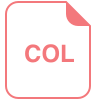
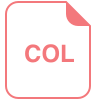
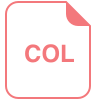