python 欧拉角可视化
时间: 2023-11-16 18:00:16 浏览: 76
欧拉角可视化可以使用Python中的Matplotlib库来实现。以下是一个简单的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 定义欧拉角
yaw = np.pi/4
pitch = np.pi/4
roll = np.pi/4
# 计算旋转矩阵
R_yaw = np.array([[np.cos(yaw), -np.sin(yaw), 0],
[np.sin(yaw), np.cos(yaw), 0],
[0, 0, 1]])
R_pitch = np.array([[np.cos(pitch), 0, np.sin(pitch)],
[0, 1, 0],
[-np.sin(pitch), 0, np.cos(pitch)]])
R_roll = np.array([[1, 0, 0],
[0, np.cos(roll), -np.sin(roll)],
[0, np.sin(roll), np.cos(roll)]])
R = R_yaw.dot(R_pitch).dot(R_roll)
# 定义坐标轴
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.set_xlim([-1, 1])
ax.set_ylim([-1, 1])
ax.set_zlim([-1, 1])
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
# 定义坐标轴上的点
x = np.array([1, 0, 0])
y = np.array([0, 1, 0])
z = np.array([0, 0, 1])
# 绘制坐标轴
ax.quiver(0, 0, 0, x[0], x[1], x[2], color='r')
ax.quiver(0, 0, 0, y[0], y[1], y[2], color='g')
ax.quiver(0, 0, 0, z[0], z[1], z[2], color='b')
# 绘制旋转后的坐标轴
x_rot = R.dot(x)
y_rot = R.dot(y)
z_rot = R.dot(z)
ax.quiver(0, 0, 0, x_rot[0], x_rot[1], x_rot[2], color='r', linestyle='--')
ax.quiver(0, 0, 0, y_rot[0], y_rot[1], y_rot[2], color='g', linestyle='--')
ax.quiver(0, 0, 0, z_rot[0], z_rot[1], z_rot[2], color='b', linestyle='--')
plt.show()
```
这段代码可以绘制出一个坐标轴和旋转后的坐标轴,可以通过修改欧拉角来观察旋转后的效果。你也可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
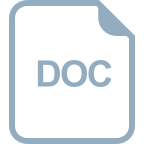
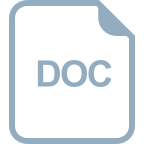
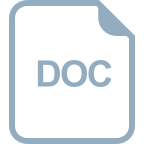
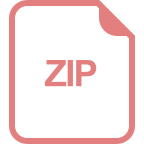
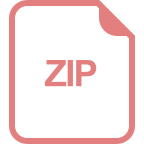
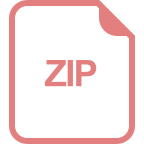
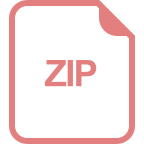
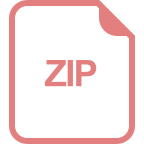
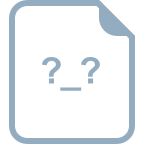
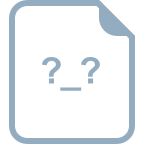
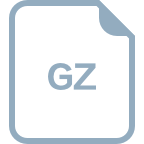
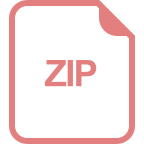
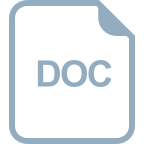
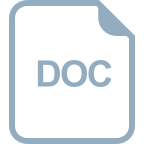
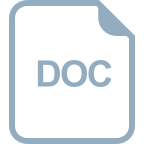
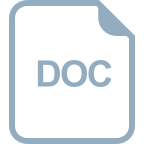
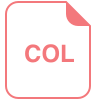
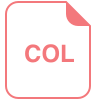
