while (<$file_a>) { s/ABCDEF/ABCD123DEF/g; # g标志表示全局替换 print $file_b $_; }
时间: 2024-10-22 07:21:14 浏览: 22
在Perl脚本中,`while (<$file_a>)` 用于逐行读取文件 `$file_a` 的内容。当遇到每一行时,`s/ABCDEF/ABCD123DEF/g` 这一行执行正则表达式替换操作。这里 `s` 是 substitute(替换)的缩写,`/ABCDEF` 是查找模式,`/ABCD123DEF` 是替换字符串,`g` 表示全局匹配(即在整个行中查找并替换所有匹配),而不是仅限第一个。
具体过程如下:
1. 打开文件 `$file_a` 并进入循环。
2. 对当前行(存储在 $_ 变量中)应用正则表达式,将所有出现的 "ABCDEF" 替换为 "ABCD123DEF"。
3. 替换后的行被打印到另一个文件 `$file_b` 中。
完整代码示例:
```perl
open(my $file_a, '<', 'input.txt') or die "Could not open file '$file_a' $!";
open(my $file_b, '>', 'output.txt') or die "Could not open file '$file_b' $!";
while (<$file_a>) {
s/ABCDEF/ABCD123DEF/g;
print $file_b $_;
}
close($file_a);
close($file_b);
```
这段代码会读取`input.txt`,将所有"ABCDEF"替换为"ABCD123DEF",并将结果写入`output.txt`。
相关问题
<!DOCTYPE html><html lang="en"> <head> <meta charset="UTF-8"> <title>My Interactive Web Page</title> <style> body { background-color: #f2f2f2; font-family: Arial, sans-serif; } h1 { text-align: center; color: #333; } p { font-size: 18px; line-height: 1.5; margin-bottom: 20px; } .container { max-width: 800px; margin: 0 auto; padding: 20px; } .button { display: inline-block; padding: 10px 20px; background-color: #333; color: #fff; text-align: center; text-decoration: none; font-size: 16px; border-radius: 5px; transition: background-color 0.3s ease; cursor: pointer; } .button:hover { background-color: #666; } </style> </head> <body> <div class="container"> <h1>欢迎来到我的网站</h1> <p>这是一个简单的网页,演示了使用HTML、CSS和JavaScript来创建的体验。.</p> <p>单击下面的按钮更改页面的背景色:</p> <button class="button" onclick="changeBackgroundColor()">改变颜色</button> <p>这里是跟多的内容:</p> <ul> <li><a href="http://www.baidu.com" >百度一下,你就知道</a></li> <li><a href="https://www.taobao.com" >淘宝网</a></li> <li><a href="https://weibo.com" >微博</a></li> </ul> </div> <script> function changeBackgroundColor() { var body = document.querySelector('body'); body.style.backgroundColor = getRandomColor(); } function getRandomColor() { var letters = '0123456789ABCDEF'; var color = '#'; for (var i = 0; i < 6; i++) { color += letters[Math.floor(Math.random() * 16)]; } return color; } </script> </body> </html>,请在此代码中加入更多内容,使网页显示跟丰富
的信息。
好的,为您添加一些内容,如下所示:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My Interactive Web Page</title>
<style>
body {
background-color: #f2f2f2;
font-family: Arial, sans-serif;
}
h1 {
text-align: center;
color: #333;
}
p {
font-size: 18px;
line-height: 1.5;
margin-bottom: 20px;
}
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
}
.button {
display: inline-block;
padding: 10px 20px;
background-color: #333;
color: #fff;
text-align: center;
text-decoration: none;
font-size: 16px;
border-radius: 5px;
transition: background-color 0.3s ease;
cursor: pointer;
}
.button:hover {
background-color: #666;
}
.image {
text-align: center;
margin-top: 20px;
}
.image img {
max-width: 100%;
height: auto;
}
.quote {
font-style: italic;
text-align: center;
margin-top: 20px;
margin-bottom: 20px;
}
.list {
margin-top: 20px;
}
.list h2 {
margin-bottom: 10px;
}
.list ul {
list-style: none;
margin: 0;
padding: 0;
}
.list li {
margin-bottom: 10px;
}
.list li a {
color: #333;
text-decoration: none;
font-weight: bold;
}
.list li a:hover {
text-decoration: underline;
}
</style>
</head>
<body>
<div class="container">
<h1>欢迎来到我的网站</h1>
<p>这是一个简单的网页,演示了使用HTML、CSS和JavaScript来创建的体验。.</p>
<p>单击下面的按钮更改页面的背景色:</p>
<button class="button" onclick="changeBackgroundColor()">改变颜色</button>
<div class="image">
<img src="https://picsum.photos/800/400" alt="随机图片">
</div>
<div class="quote">
<p>"生命就像一盒巧克力,你永远不知道你会得到什么。"</p>
<p>-《阿甘正传》</p>
</div>
<div class="list">
<h2>一些有趣的链接</h2>
<ul>
<li><a href="http://www.baidu.com" >百度一下,你就知道</a></li>
<li><a href="https://www.taobao.com" >淘宝网</a></li>
<li><a href="https://weibo.com" >微博</a></li>
<li><a href="https://www.youtube.com" >YouTube</a></li>
<li><a href="https://www.instagram.com" >Instagram</a></li>
</ul>
</div>
</div>
<script>
function changeBackgroundColor() {
var body = document.querySelector('body');
body.style.backgroundColor = getRandomColor();
}
function getRandomColor() {
var letters = '0123456789ABCDEF';
var color = '#';
for (var i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
</script>
</body>
</html>
在上述代码中,我添加了以下内容:
- 一个随机图片,使用了 https://picsum.photos 提供的 API 来获取一张随机图片,并将其居中显示。
- 一条经典的电影名言,并将其以斜体显示,并居中在页面上方。
- 一个链接列表,其中包括了一些有趣的站点。将其放在页面底部,使用了一个标题和一个无序列表来组织这些链接。
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <title>My Interactive Web Page</title> <style> body { background-color: #f2f2f2; font-family: Arial, sans-serif; } h1 { text-align: center; color: #333; } p { font-size: 18px; line-height: 1.5; margin-bottom: 20px; } .container { max-width: 800px; margin: 0 auto; padding: 20px; } .button { display: inline-block; padding: 10px 20px; background-color: #333; color: #fff; text-align: center; text-decoration: none; font-size: 16px; border-radius: 5px; transition: background-color 0.3s ease; cursor: pointer; } .button:hover { background-color: #666; } </style></head><body> <div class="container"> <h1>Welcome to my interactive web page</h1> <p>This is a simple web page that demonstrates how to use HTML, CSS, and JavaScript to create an interactive user experience.</p> <p>Click the button below to change the background color of the page:</p> <button class="button" onclick="changeBackgroundColor()">Change Color</button> </div> <script> function changeBackgroundColor() { var body = document.querySelector('body'); body.style.backgroundColor = getRandomColor(); } function getRandomColor() { var letters = '0123456789ABCDEF'; var color = '#'; for (var i = 0; i < 6; i++) { color += letters[Math.floor(Math.random() * 16)]; } return color; } </script></body></html>根据以上代码插入适当的代码,显示更多的内容
可以在<div class="container">标签后面添加更多的HTML内容,例如:
<div class="container">
<h1>Welcome to my interactive web page</h1>
<p>This is a simple web page that demonstrates how to use HTML, CSS, and JavaScript to create an interactive user experience.</p>
<p>Click the button below to change the background color of the page:</p>
<button class="button" onclick="changeBackgroundColor()">Change Color</button>
<p>Here is some more content:</p>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<p>You can add as much content as you want here!</p>
</div>
阅读全文
相关推荐
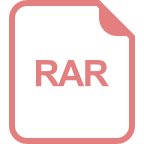
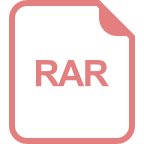
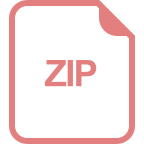














