如何在MATLAB中编写代码来计算四元数矩阵的逆?
时间: 2024-12-08 17:16:29 浏览: 19
在MATLAB中,你可以使用quatinv函数来计算四元数矩阵的逆。以下是基本步骤:
```matlab
% 假设你有一个四元素向量表示的四元数q
q = [q0; q1; q2; q3];
% 将四元数转换成复数形式的四行四列矩阵
quaternion_matrix = [q(1); q(2)+q(3)*1i; q(4)+q(5)*1i; q(6)+q(7)*1i];
% 使用quatinv函数计算逆四元数矩阵
inverse_quaternion_matrix = quatinv(quaternion_matrix);
% 结果是一个复数四行四列矩阵,你需要将其拆分成四个元素向量
inverse_q = [inverse_quaternion_matrix(1,1), inverse_quaternion_matrix(2,1) - inverse_quaternion_matrix(3,1)*1i, ...
inverse_quaternion_matrix(4,1) - inverse_quaternion_matrix(5,1)*1i, ...
-inverse_quaternion_matrix(2,1)*1i + inverse_quaternion_matrix(3,1)];
```
请注意,这个过程假定输入的是一个单位四元数,如果不是,则需要先归一化再计算逆。
相关问题
使用matlab编写将一个三维方向余弦矩阵转换为四元数,并对四元数进行单位化
在MATLAB中,可以使用以下代码将一个3x3的方向余弦矩阵转换为四元数:
```matlab
function q = dcm2quat(dcm)
% DCM2QUAT Convert direction cosine matrix to quaternion
% q = DCM2QUAT(dcm) converts a 3x3 direction cosine matrix (DCM) to a 1x4
% quaternion. The DCM input represents the rotation from frame 2 to frame
% 1, where frame 1 is the global frame and frame 2 is the rotated frame.
%
% Reference: Eq. 2.65 and 2.67, "Quaternion Kinematics for the Error-State
% Kalman Filter", Joan Solà, 2017.
% Check input size
if any(size(dcm) ~= [3 3])
error('Input matrix must be 3x3.')
end
% Compute quaternion elements
q0 = 0.5 * sqrt(1 + dcm(1,1) + dcm(2,2) + dcm(3,3));
q1 = (dcm(3,2) - dcm(2,3)) / (4*q0);
q2 = (dcm(1,3) - dcm(3,1)) / (4*q0);
q3 = (dcm(2,1) - dcm(1,2)) / (4*q0);
% Return quaternion as a row vector
q = [q0 q1 q2 q3];
```
然后,可以使用以下代码将四元数单位化:
```matlab
function q_unit = quatnormalize(q)
% QUATNORMALIZE Normalize quaternion
% q_unit = QUATNORMALIZE(q) normalizes the quaternion q to have unit
% magnitude.
% Check input size
if any(size(q) ~= [1 4])
error('Input vector must be 1x4.')
end
% Compute quaternion magnitude
q_mag = norm(q);
% Normalize quaternion
q_unit = q / q_mag;
```
现在,您可以将这两个函数组合在一起,使用以下代码将方向余弦矩阵转换为单位四元数:
```matlab
% Example DCM
dcm = [0.7071 -0.7071 0; 0.7071 0.7071 0; 0 0 1];
% Convert DCM to quaternion
q = dcm2quat(dcm);
% Normalize quaternion
q_unit = quatnormalize(q);
```
使用matlab编写四元数的单位化
假设四元数为q,其单位化后的四元数为q_unit。
步骤如下:
1. 计算四元数的模长 q_norm = norm(q),其中 norm 表示向量或矩阵的模长。
2. 如果四元数的模长小于等于 0,则将单位化后的四元数 q_unit 设为原始四元数 q。
3. 如果四元数的模长大于 0,则将单位化后的四元数 q_unit 计算为 q_norm 的倒数乘以原始四元数 q,即:
q_unit = q / q_norm
4. 返回单位化后的四元数 q_unit。
以下是 MATLAB 代码实现:
function q_unit = quat_unit(q)
% q: 原始四元数
% q_unit: 单位化后的四元数
q_norm = norm(q);
if q_norm <= 0
q_unit = q;
else
q_unit = q / q_norm;
end
end
阅读全文
相关推荐
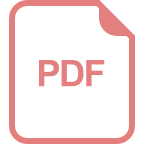
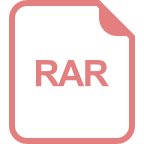
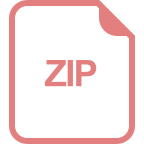
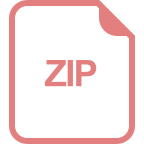
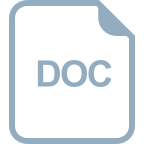
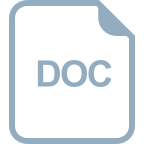
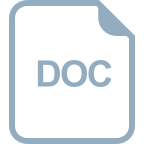
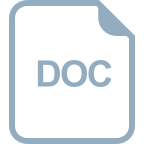
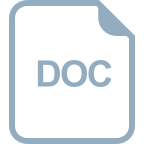
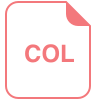
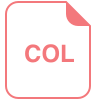
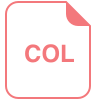
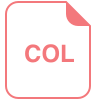
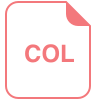
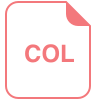
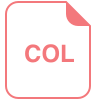
