遗传算法python程序
时间: 2023-10-15 13:07:52 浏览: 59
遗传算法是一种经典的优化算法,可以用于解决各种问题。以下是一个简单的遗传算法的Python程序示例:
```python
import random
# 遗传算法的参数设定
population_size = 100 # 种群大小
chromosome_length = 10 # 染色体长度
crossover_rate = 0.8 # 交叉概率
mutation_rate = 0.01 # 变异概率
generations = 100 # 迭代次数
# 初始化种群
def init_population():
population = []
for _ in range(population_size):
chromosome = [random.randint(0, 1) for _ in range(chromosome_length)]
population.append(chromosome)
return population
# 计算适应度(这里以染色体中1的数量作为适应度)
def calculate_fitness(chromosome):
return sum(chromosome)
# 选择过程(这里使用轮盘赌选择算法)
def selection(population):
fitness_values = [calculate_fitness(chromosome) for chromosome in population]
total_fitness = sum(fitness_values)
probabilities = [fitness / total_fitness for fitness in fitness_values]
selected_population = random.choices(population, probabilities, k=population_size)
return selected_population
# 交叉过程(这里使用单点交叉)
def crossover(parent1, parent2):
if random.random() < crossover_rate:
crossover_point = random.randint(1, chromosome_length - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
else:
return parent1, parent2
# 变异过程(这里使用位翻转变异)
def mutation(chromosome):
mutated_chromosome = chromosome.copy()
for i in range(chromosome_length):
if random.random() < mutation_rate:
mutated_chromosome[i] = 1 - mutated_chromosome[i]
return mutated_chromosome
# 遗传算法主流程
def genetic_algorithm():
population = init_population()
for _ in range(generations):
population = selection(population)
next_generation = []
for i in range(0, population_size, 2):
parent1 = population[i]
parent2 = population[i+1]
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1)
child2 = mutation(child2)
next_generation.extend([child1, child2])
population = next_generation
best_chromosome = max(population, key=calculate_fitness)
best_fitness = calculate_fitness(best_chromosome)
return best_chromosome, best_fitness
# 运行遗传算法
best_chromosome, best_fitness = genetic_algorithm()
print("Best chromosome:", best_chromosome)
print("Best fitness:", best_fitness)
```
这个程序演示了一个简单的二进制编码遗传算法,其中染色体表示为由0和1组成的列表。通过选择、交叉和变异等操作,逐步优化种群中的染色体,最终得到具有最优适应度的染色体。在这个例子中,适应度被定义为染色体中1的数量。你可以根据自己的需求和问题进行相应的修改和扩展。
相关推荐
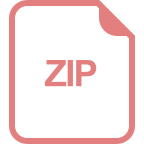
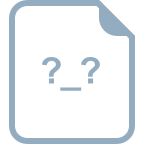
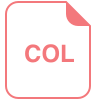
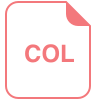
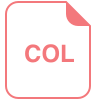
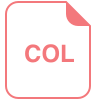
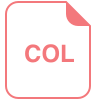









