python 遗传算法示例
时间: 2024-05-28 09:07:31 浏览: 147
Python 是一门非常流行的编程语言,在遗传算法方面也有很多优秀的库和示例。遗传算法是一种基于生物进化理论的优化算法,可以用于解决许多优化问题,如函数优化、组合优化、路径规划等。
下面是一个 Python 实现的简单遗传算法示例:
```python
import random
# 目标函数
def fitness(x):
return x * x
# 初始化种群
def init_population(pop_size, gene_length):
population = []
for i in range(pop_size):
gene = []
for j in range(gene_length):
gene.append(random.randint(0, 1))
population.append(gene)
return population
# 计算适应度
def calc_fitness(population):
fitness_values = []
for gene in population:
x = int(''.join(map(str, gene)), 2)
fitness_values.append(fitness(x))
return fitness_values
# 选择
def selection(population, fitness_values):
total_fitness = sum(fitness_values)
p = [f / total_fitness for f in fitness_values]
p_sum = [sum(p[:i+1]) for i in range(len(p))]
selected_population = []
for i in range(len(population)):
r = random.random()
for j in range(len(p_sum)):
if r <= p_sum[j]:
selected_population.append(population[j])
break
return selected_population
# 交叉
def crossover(parent1, parent2):
pos = random.randint(0, len(parent1) - 1)
child1 = parent1[:pos] + parent2[pos:]
child2 = parent2[:pos] + parent1[pos:]
return child1, child2
# 变异
def mutation(gene, mutation_rate):
for i in range(len(gene)):
if random.random() < mutation_rate:
gene[i] = 1 - gene[i]
return gene
# 遗传算法主程序
def genetic_algorithm(pop_size, gene_length, num_generation, crossover_rate, mutation_rate):
population = init_population(pop_size, gene_length)
for i in range(num_generation):
fitness_values = calc_fitness(population)
selected_population = selection(population, fitness_values)
next_population = []
for j in range(int(len(selected_population) / 2)):
parent1 = selected_population[j * 2]
parent2 = selected_population[j * 2 + 1]
if random.random() < crossover_rate:
child1, child2 = crossover(parent1, parent2)
else:
child1, child2 = parent1, parent2
child1 = mutation(child1, mutation_rate)
child2 = mutation(child2, mutation_rate)
next_population.append(child1)
next_population.append(child2)
population = next_population
fitness_values = calc_fitness(population)
best_gene = population[fitness_values.index(max(fitness_values))]
x = int(''.join(map(str, best_gene)), 2)
print("最优解:x =", x, "f(x) =", fitness(x))
# 示例运行
genetic_algorithm(pop_size=50, gene_length=6, num_generation=100, crossover_rate=0.8, mutation_rate=0.01)
```
这个示例是一个简单的二进制遗传算法,目标函数为 f(x) = x²,求在 x∈[0, 63] 范围内的最大值。代码中包括了初始化种群、计算适应度、选择、交叉和变异等基本操作。
阅读全文
相关推荐
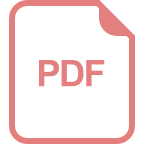

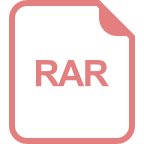
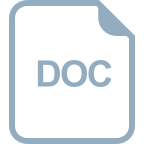












