C# 抓取其他程序中PICTUREBOX控件中的图片
时间: 2024-12-17 17:33:41 浏览: 3
在C#中抓取其他程序中PictureBox 控件里的图片通常涉及到屏幕截图或者是访问进程的内存。你可以使用Windows API函数如`GetDC`、`BitBlt`等来获取窗口的设备上下文(Device Context),然后通过复制像素数据到一个新的位图中,最后保存这个位图作为图片。
以下是一个简化的示例,展示了如何获取PictureBox的屏幕截图:
```csharp
using System;
using System.Drawing;
using System.Runtime.InteropServices;
public class ScreenCapture
{
[DllImport("user32.dll")]
private static extern IntPtr GetWindowDC(IntPtr hWnd);
[DllImport("gdi32.dll")]
private static extern int PrintWindow(IntPtr hWnd, IntPtr hdcBlt, uint nFlags);
[DllImport("gdi32.dll")]
private static extern int DeleteObject(IntPtr hObject);
public static Image CapturePictureBox(PictureBox pictureBox)
{
if (pictureBox == null) throw new ArgumentNullException(nameof(pictureBox));
// 获取PictureBox的窗口句柄
IntPtr hwnd = pictureBox.Handle;
// 获取PictureBox的设备上下文
IntPtr hdc = GetWindowDC(hwnd);
try
{
// 打开一个新位图来存储截图
IntPtr hBitmap = CreateCompatibleBitmap(hdc, pictureBox.Width, pictureBox.Height);
Graphics g = Graphics.FromHdc(hBitmap);
// 拷贝PictureBox的内容到新位图上
PrintWindow(hwnd, hBitmap, 0);
// 创建一个Image对象并加载截图
Bitmap bitmap = new Bitmap(hBitmap);
return bitmap;
}
finally
{
// 清理资源
ReleaseDC(hwnd, hdc);
DeleteObject(hBitmap);
}
}
[DllImport("gdi32.dll", SetLastError = true)]
private static extern bool ReleaseDC(IntPtr hWnd, IntPtr hDC);
[DllImport("gdi32.dll", SetLastError = true)]
private static extern IntPtr CreateCompatibleBitmap(IntPtr hDC, int width, int height);
}
```
使用此方法时,你需要传入你要抓取的PictureBox实例。请注意,这可能会受到权限限制,并且如果目标应用程序正在运行防屏拍策略,那么这种方法可能无法成功。
阅读全文
相关推荐
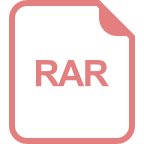
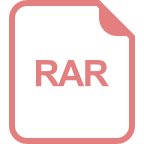
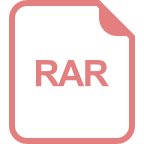

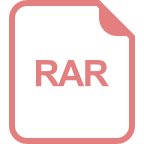
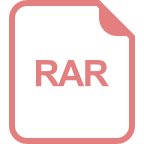
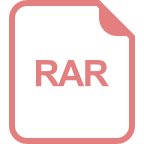
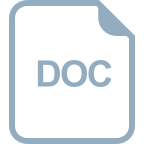

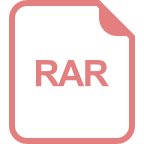
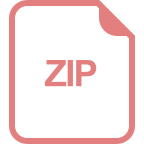
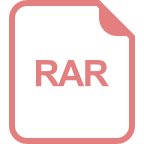
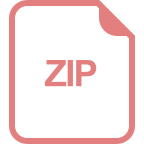
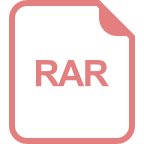
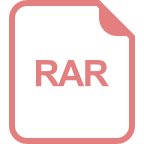
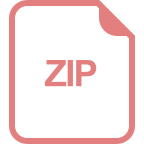
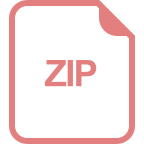
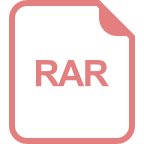
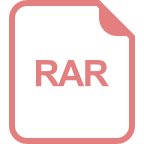