在学生基本信息表中,包含了每个学生的学号、姓名和成绩等相关信息。请编写程序,输入每个学生的信息,并按照成绩从低到高的顺序输出每个学生的信息。使用c++与结构体 不使用函数
时间: 2024-09-26 07:16:47 浏览: 28
在C++中,你可以使用结构体(struct)来定义一个学生的基本信息,然后使用循环遍历并比较成绩。下面是一个简单的示例:
```cpp
#include <iostream>
#include <string>
// 定义学生信息结构体
struct Student {
int id; // 学号
std::string name; // 姓名
float score; // 成绩
// 按成绩排序的运算符重载,用于自定义排序
bool operator<(const Student& other) const {
return score < other.score;
}
};
int main() {
Student students[5]; // 假设最多有5个学生
int numStudents = 0;
// 输入学生信息
while (numStudents < 5) {
std::cout << "请输入第" << numStudents + 1 << "位学生的学号、姓名和成绩:\n";
std::cin >> students[numStudents].id >> students[numStudents].name >> students[numStudents].score;
numStudents++;
}
// 按照成绩从低到高排序
for (int i = 0; i < numStudents - 1; i++) {
for (int j = 0; j < numStudents - i - 1; j++) {
if (students[j] < students[j + 1]) {
// 交换记录
Student temp = students[j];
students[j] = students[j + 1];
students[j + 1] = temp;
}
}
}
// 输出排序后的学生信息
std::cout << "学生信息按成绩从低到高排序:\n";
for (Student student : students) {
std::cout << "学号:" << student.id << ", 姓名:" << student.name << ", 成绩:" << student.score << "\n";
}
return 0;
}
相关推荐
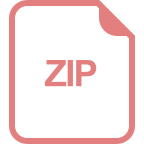
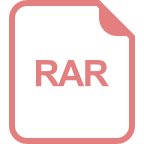
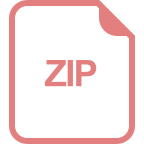








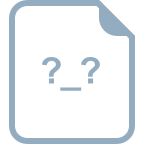
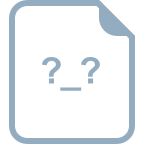
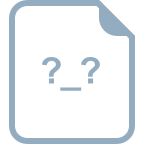
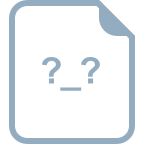
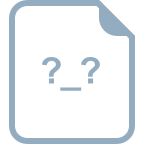