参考word_predict中的代码,从其中调用RNN,GRU,LSTM的类 实现单层RNN,GRU,LSTM的嵌套架构
时间: 2024-10-05 07:04:06 浏览: 23
在Python的`word_predict`代码中,通常会利用深度学习库如TensorFlow或PyTorch来实现RNN(循环神经网络),GRU(门控循环单元)和LSTM(长短时记忆网络)。下面是一个简化的示例,展示如何分别创建单层的RNN、GRU和LSTM模块:
```python
import tensorflow as tf
# 单层RNN
class SimpleRNN(tf.keras.layers.Layer):
def __init__(self, units, input_shape):
super(SimpleRNN, self).__init__()
self.rnn = tf.keras.layers.SimpleRNN(units, return_sequences=True)
self.input_shape = input_shape
def call(self, inputs):
x = tf.reshape(inputs, (-1,) + self.input_shape) # Reshape input to match RNN's expectations
output = self.rnn(x)
return output
# 单层GRU
class SimpleGRU(tf.keras.layers.Layer):
def __init__(self, units, input_shape):
super(SimpleGRU, self).__init__()
self.gru = tf.keras.layers.GRU(units, return_sequences=True)
self.input_shape = input_shape
def call(self, inputs):
x = tf.reshape(inputs, (-1,) + self.input_shape) # Similar reshape for GRU
output = self.gru(x)
return output
# 单层LSTM
class SimpleLSTM(tf.keras.layers.Layer):
def __init__(self, units, input_shape):
super(SimpleLSTM, self).__init__()
self.lstm = tf.keras.layers.LSTM(units, return_sequences=True)
self.input_shape = input_shape
def call(self, inputs):
x = tf.reshape(inputs, (-1,) + self.input_shape) # LSTM also needs reshaping
output = self.lstm(x)
return output
```
在这段代码中,每个类都继承自`tf.keras.layers.Layer`,并设置了相应的RNN、GRU或LSTM层作为实例属性。`call`方法用于接收输入数据并通过这些层生成输出。
相关推荐
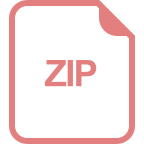
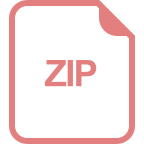
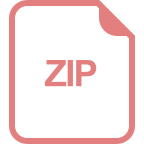














