一款游戏中,具有多种植物角色角色可供用户选择,豌豆射手、坚果墙、向日葵。。。 要求使用简单工厂,为用户提供角色实例对象。写出代码。
时间: 2024-10-12 08:13:17 浏览: 27
在一款游戏中,我们可以使用简单工厂模式创建不同类型的植物角色。简单工厂模式是一种创建型设计模式,它提供了一种创建对象的最佳方式,而不必暴露其内部创建逻辑。下面是一个简单的Java代码示例:
```java
// 定义一个接口,表示植物角色
interface Plant {
void attack();
}
// 实现各种植物角色
class PeaShooter implements Plant {
public void attack() {
System.out.println("Pea Shooter is shooting peas!");
}
}
class NutWall implements Plant {
public void attack() {
System.out.println("Nut Wall is blocking with nuts!");
}
}
class SunFlower implements Plant {
public void attack() {
System.out.println("Sun Flower is regenerating energy.");
}
}
// 简单工厂类
class PlantFactory {
// 创建对应类型的植物角色
public static Plant createPlant(String type) {
switch (type) {
case "peashooter":
return new PeaShooter();
case "nutwall":
return new NutWall();
case "sunflower":
return new SunFlower();
default:
throw new IllegalArgumentException("Invalid plant type");
}
}
}
// 用户部分
public class GameUser {
public static void main(String[] args) {
Plant myPlant = PlantFactory.createPlant("peashooter"); // 使用工厂创建豌豆射手
myPlant.attack(); // 调用攻击方法
// 更改植物类型
myPlant = PlantFactory.createPlant("nutwall");
myPlant.attack();
}
}
```
在这个例子中,`PlantFactory`充当了简单工厂,根据传入的角色类型字符串动态创建并返回对应的植物角色实例。
阅读全文
相关推荐
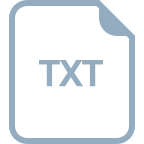
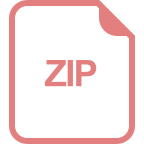
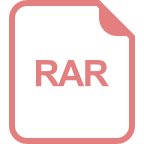
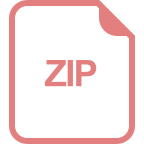
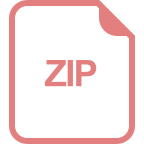
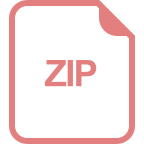
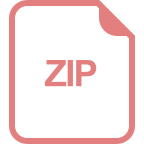
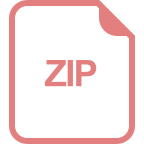
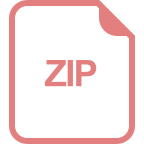
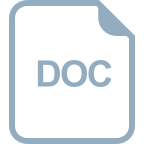
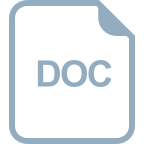
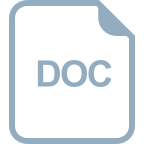
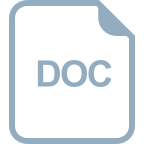
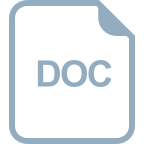
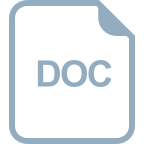
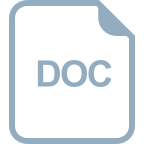
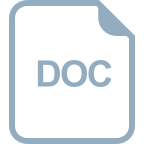


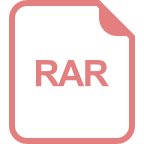