#include <iostream> #include <fstream> #include <string> #include <cstring> using namespace std; void run_length_coding(char* src, char* dst) { /**********Program**********/ int count = 1; char prevChar = src[0]; int index = 0; for (int i = 1; i < strlen(src); i++) { if (src[i] == prevChar) { count++; } else { dst[index++] = count + '0'; dst[index++] = prevChar; count = 1; prevChar = src[i]; } } dst[index++] = count + '0'; dst[index++] = prevChar; dst[index] = '\0'; /********** End **********/ } int main() { char s[1000], t[1000]; cin >> s; int k = strlen(s); run_length_coding(s, t); cout << t << endl; return 0; } 做每一行代码的详细注释
时间: 2023-08-09 11:12:35 浏览: 174
这段代码实现了一个简单的行程长度编码(run-length coding)算法。下面是对每一行代码的详细注释:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <cstring>
using namespace std;
void run_length_coding(char* src, char* dst)
{
// 定义变量
int count = 1; // 用于记录字符重复的次数
char prevChar = src[0]; // 用于记录前一个字符
int index = 0; // 用于记录目标字符串的索引位置
// 遍历源字符串
for (int i = 1; i < strlen(src); i++) {
// 如果当前字符与前一个字符相同,增加重复次数
if (src[i] == prevChar) {
count++;
}
else {
// 当前字符与前一个字符不同,将重复次数和前一个字符存入目标字符串
dst[index++] = count + '0';
dst[index++] = prevChar;
// 重置重复次数和前一个字符
count = 1;
prevChar = src[i];
}
}
// 存入最后一个字符的重复次数和字符本身
dst[index++] = count + '0';
dst[index++] = prevChar;
// 添加字符串结尾标志
dst[index] = '\0';
}
int main()
{
char s[1000], t[1000];
cin >> s; // 输入源字符串
int k = strlen(s); // 获取源字符串的长度
run_length_coding(s, t); // 调用行程长度编码函数
cout << t << endl; // 输出编码后的字符串
return 0;
}
```
这段代码中的 `run_length_coding` 函数接收两个参数:源字符串 `src` 和目标字符串 `dst`。它通过遍历源字符串,计算每个字符的重复次数,并将结果存入目标字符串中。最后,在 `main` 函数中,我们接收用户输入的源字符串,调用 `run_length_coding` 函数进行编码,并输出结果。
阅读全文
相关推荐












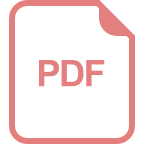




