C++ winpcap网络抓包代码实现
时间: 2024-12-17 12:12:44 浏览: 3
在C++中,WinPcap是一个流行的网络捕获库,用于实时网络数据包的获取和分析。以下是使用WinPcap编写基础网络抓包代码的一个简单示例:
```cpp
#include <pcap.h>
#include <iostream>
// 函数声明
void packet_handler(u_char* user, const struct pcap_pkthdr* pkthdr, const u_char* packet);
int main() {
char errbuf[PCAP_ERRBUF_SIZE];
pcap_t* handle = pcap_open_live("eth0", BUFSIZ, 1, 1000, errbuf); // eth0为目标网卡,缓冲区大小,是否过滤,超时时间
if (handle == NULL) {
std::cerr << "Error opening capture device: " << errbuf << std::endl;
return -1;
}
// 设置处理数据包的函数
pcap_setfilter(handle, "ip and tcp");
// 注册回调函数并开始抓包
pcap_loop(handle, -1, packet_handler, NULL); // -1表示无限循环,直到手动停止
pcap_close(handle);
return 0;
}
// 数据包处理函数
void packet_handler(u_char* user, const struct pcap_pkthdr* pkthdr, const u_char* packet) {
struct ether_header* eth_hdr = (struct ether_header*)packet;
struct iphdr* ip_hdr = (struct iphdr*)(packet + sizeof(struct ether_header));
printf("Source IP: %d.%d.%d.%d\n", ip_hdr->ihl * 4 + ip_hdr->saddr >> 24,
(ip_hdr->ihl * 4 + ip_hdr->saddr) & 0xFF,
(ip_hdr->ihl * 4 + ip_hdr->saddr) >> 8 & 0xFF,
ip_hdr->ihl * 4 + ip_hdr->saddr & 0xFF);
// 更深入的数据解析可以在这里添加...
}
```
这个代码片段首先打开指定的网络设备(如"eth0"),然后设置过滤条件(例如只抓取IP和TCP协议的数据包)。`packet_handler`函数会在每次接收到符合条件的数据包时被调用,你可以在这个函数里根据需要解析网络数据。
阅读全文
相关推荐
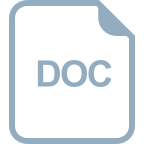
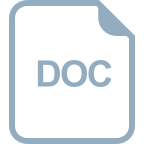
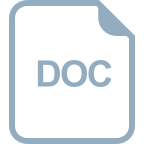

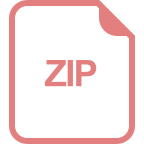
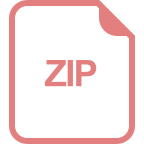
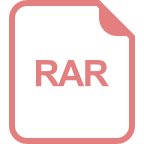
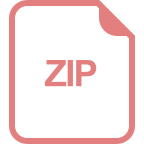
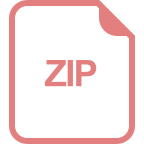
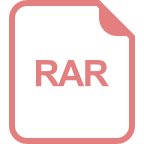
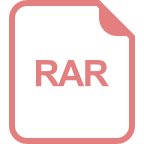
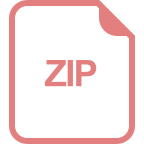
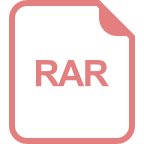
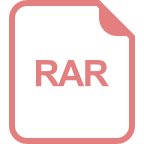
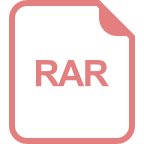
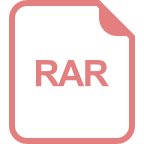
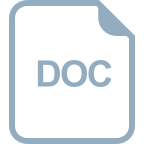