Python画环形图
时间: 2024-09-26 09:17:51 浏览: 31
在Python中,你可以使用matplotlib库中的`pie()`函数来创建环形图,也被称为饼图。环形图用于显示各部分占总体的比例,并通过颜色和标签清晰地展示出来。以下是一个简单的例子:
```python
import matplotlib.pyplot as plt
# 数据
labels = ['部分A', '部分B', '部分C', '部分D']
sizes = [40, 30, 25, 5] # 各部分所占百分比,总和应为100%
# 创建环形图
fig, ax = plt.subplots()
ax.pie(sizes, labels=labels, autopct='%1.1f%%', startangle=140, pctdistance=0.85) # 自动添加百分比标签
# 添加背景圆环
centre_circle = plt.Circle((0,0),0.70,fc='white')
fig = plt.gcf()
fig.gca().add_artist(centre_circle)
# 设置图形标题和标签
plt.title('环形图示例')
plt.axis('equal') # 确保饼图是正圆形的,而不是椭圆形
plt.show()
相关问题
python画环形图_用Python把图做的好看点:用Matplotlib画个环形图
好的,以下是用 Python 的 Matplotlib 库画环形图的示例代码:
```python
import matplotlib.pyplot as plt
# 数据
labels = ['A', 'B', 'C', 'D']
sizes = [15, 30, 45, 10]
# 颜色
colors = ['#ff9999','#66b3ff','#99ff99','#ffcc99']
# 环形图
fig1, ax1 = plt.subplots()
# 内环
ax1.pie([100], radius=0.7, colors=['w'])
# 外环
ax1.pie(sizes,
labels=labels,
colors=colors,
autopct='%1.1f%%',
startangle=90,
pctdistance=0.85,
labeldistance=1.1)
# 标题
ax1.set_title("环形图示例")
# 显示
plt.show()
```
运行以上代码,即可得到一个简单的环形图。
解释:
1. `labels` 和 `sizes` 分别为环形图的标签和大小。
2. `colors` 为环形图各部分的颜色。
3. `fig1, ax1 = plt.subplots()` 创建一个子图。
4. `ax1.pie([100], radius=0.7, colors=['w'])` 画内环。`[100]` 表示内环大小为 100,`radius=0.7` 表示内环半径为外环半径的 0.7,`colors=['w']` 表示内环颜色为白色。
5. `ax1.pie(sizes, labels=labels, colors=colors, autopct='%1.1f%%', startangle=90, pctdistance=0.85, labeldistance=1.1)` 画外环。`sizes` 为各部分的大小,`labels` 为各部分的标签,`colors` 为各部分的颜色。`autopct='%1.1f%%'` 表示显示各部分的百分比。`startangle=90` 表示从 90 度开始画图。`pctdistance=0.85` 表示百分比标签与圆心的距离,`labeldistance=1.1` 表示标签与圆心的距离。
6. `ax1.set_title("环形图示例")` 设置环形图标题。
7. `plt.show()` 显示图形。
python绘制多层环形图
要在Python中绘制多层环形图,你可以使用matplotlib库的pie函数,并设置不同的半径和参数。以下是一个简单的示例代码:
```
import matplotlib.pyplot as plt
# 根据需要设置数据和标签
sizes = [40, 30, 20, 10]
labels = ['A', 'B', 'C', 'D']
colors = ['#ff9999','#66b3ff','#99ff99','#ffcc99']
# 绘制第一层环形图
plt.pie(sizes, labels=labels, colors=colors, autopct='%1.1f%%', startangle=90)
# 设置第二层环形图的参数
radius = 0.5 # 内圈半径
colors2 = ['#ff6666','#66b3ff','#99ff99','#ffcc99']
# 绘制第二层环形图
plt.pie(sizes, labels=labels, colors=colors2, autopct='%1.1f%%', startangle=90, radius=radius)
# 添加中心圆以创建环形图效果
center_circle = plt.Circle((0,0),0.35,color='white', fc='white',linewidth=0)
# 将中心圆添加到图形中
fig = plt.gcf()
fig.gca().add_artist(center_circle)
# 设置图形的样式和标题
plt.axis('equal')
plt.title('Multi-layer Donut Chart')
# 显示图形
plt.show()
```
这段代码将绘制一个两层的环形图,其中第一层是完整的饼图,第二层是内部的环形图。你可以根据需要修改数据和参数来定制自己的多层环形图。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [Python通过matplotlib画双层饼图及环形图简单示例](https://blog.csdn.net/weixin_39553753/article/details/112050783)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
阅读全文
相关推荐
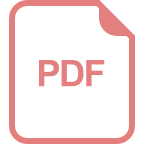
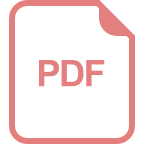
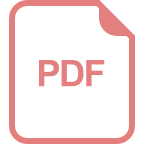











