python用字典储存表格
时间: 2023-12-29 13:22:50 浏览: 25
在Python中,可以使用字典来储存表格数据。字典的key可以作为表格的列名,而value则可以作为表格中的数据。例如,可以使用以下代码创建一个包含表格数据的字典:
```
table = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'Gender': ['Female', 'Male', 'Male']
}
```
在这个例子中,字典的key分别为Name、Age和Gender,对应的value则是包含表格数据的列表。可以通过访问字典的key来获取表格中的数据。
相关问题
python爬表格数据_python爬虫,爬取表格数据
要爬取表格数据,你需要使用 Python 爬虫库,如 Beautiful Soup 或 Scrapy。以下是一些基本步骤:
1. 发送 HTTP 请求获取网页 HTML 源代码。
2. 使用 Beautiful Soup 或 Scrapy 解析 HTML 代码,定位到表格数据在哪里。
3. 使用 Python 的列表、字典等数据类型存储表格数据。
4. 可以将数据保存为 CSV 文件或使用数据库存储数据。
以下是一个示例程序,使用 Beautiful Soup 爬取一个网页上的表格数据:
```python
import requests
from bs4 import BeautifulSoup
# 发送 HTTP 请求获取网页 HTML 源代码
url = 'https://www.example.com/table.html'
response = requests.get(url)
html = response.text
# 使用 Beautiful Soup 解析 HTML 代码
soup = BeautifulSoup(html, 'html.parser')
# 定位到表格数据在哪里
table = soup.find('table')
rows = table.find_all('tr')
# 存储表格数据
data = []
for row in rows:
cols = row.find_all('td')
cols = [col.text.strip() for col in cols]
data.append(cols)
# 输出数据
for row in data:
print(row)
```
这个程序会将表格数据存储在一个二维数组中,并逐行输出。你可以根据需要修改程序,如提取特定列的数据、筛选特定条件的行等。
用python往表格添加日期列
### 回答1:
要在Python中向表格添加日期列,您可以使用pandas库。以下是一个简单的示例代码,演示如何创建一个名为“dates”的日期列,并将其附加到名为“df”的现有数据框中:
``` python
import pandas as pd
import datetime as dt
# 创建示例数据框
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# 创建日期列
start_date = dt.date(2023, 1, 1)
end_date = dt.date(2023, 1, 3)
dates = pd.date_range(start_date, end_date)
# 将日期列添加到数据框中
df['dates'] = dates
print(df)
```
在此示例中,我们首先创建一个名为“df”的示例数据框。接下来,我们使用datetime库中的date函数创建一个起始日期和结束日期,并使用pandas库中的date_range函数创建一个包含这些日期的日期范围。最后,我们将这个日期列添加到数据框中,并打印结果。
### 回答2:
在Python中,可以使用pandas库来往表格中添加日期列。首先,需要安装pandas库,通过以下命令进行安装:
```
pip install pandas
```
接下来,导入pandas库并读取表格数据:
```python
import pandas as pd
# 读取表格数据
df = pd.read_excel('data.xlsx')
```
假设表格已经包含了其他列数据,现在要往表格中添加日期列。可以首先创建一个日期的列表,然后使用pandas库的`to_datetime`函数将列表转换为日期类型,最后将日期列插入到表格中。以下是具体的代码示例:
```python
import pandas as pd
# 读取表格数据
df = pd.read_excel('data.xlsx')
# 创建日期列表
dates = ['2021-05-01', '2021-05-02', '2021-05-03']
# 将列表转换为日期类型
date_column = pd.to_datetime(dates)
# 将日期列插入表格中
df['日期'] = date_column
# 打印更新后的表格
print(df)
```
以上代码中的`data.xlsx`是指表格文件的路径,你需要将其替换为你自己的表格文件路径。日期列表`dates`是一个包含日期字符串的Python列表,你可以根据自己的需求修改日期字符串的内容和个数。最后,通过`df['日期'] = date_column`语句将日期列插入到表格中,并使用`print(df)`打印出更新后的表格。
希望以上解答对你有帮助!如有任何疑问,请随时追问。
### 回答3:
使用Python往表格添加日期列可以使用pandas库。首先需要安装pandas库,可以通过pip install pandas命令进行安装。
接下来,我们可以使用pandas库中的DataFrame对象来创建表格,而且pandas库有内置日期处理功能。首先,导入pandas库并创建一个DataFrame对象,可以用一个列表或字典作为输入数据:
```python
import pandas as pd
# 使用列表创建DataFrame
data = [['2022-01-01', 'A', 10],
['2022-01-02', 'B', 20],
['2022-01-03', 'C', 30]]
df = pd.DataFrame(data, columns=['日期', '类别', '数值'])
# 查看原始表格
print(df)
```
以上代码将创建一个包含日期、类别和数值三列的DataFrame对象,并将数据打印出来。注意,这里的日期是以字符串的形式存储的。
接下来,我们可以将日期列转换成日期类型,并将其设置为表格的索引:
```python
# 将日期列转换成日期类型
df['日期'] = pd.to_datetime(df['日期'])
# 设置日期为索引
df.set_index('日期', inplace=True)
# 查看更新后的表格
print(df)
```
以上代码使用pd.to_datetime()方法将日期列的数据转换为日期类型。然后,使用set_index()方法将日期列设置为索引,并使用inplace=True将修改应用于原有的DataFrame对象。最后,打印更新后的表格。
使用以上方法,我们就可以往表格中添加日期列。
相关推荐
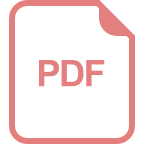
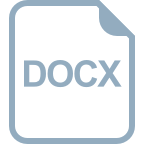












