用c语言将字符串中的第一个数值转换成十进制整数,遇到空格数值字符认为结束,例如“42 00”,输出42;“-29”,输出-29
时间: 2024-12-16 13:13:58 浏览: 4
在C语言中,你可以使用`sscanf`函数配合循环来实现这个功能。`sscanf`可以按照特定格式从字符串中读取并解析指定的数据。这里是一个简单的例子:
```c
#include <stdio.h>
int convert_to_decimal(const char *str) {
int num = 0;
int sign = 1; // 1 for positive, -1 for negative
char c;
while ((c = *str++) != '\0') { // Iterate through the string until end
if (isdigit(c)) { // If character is a digit
num = num * 10 + (c - '0'); // Convert to integer and add to number
} else if (c == '-' && str[0] != '-') { // Handle negative sign before digits
sign = -1;
} else if (isspace(c)) { // End of number when space encountered
break;
}
}
return sign * num; // Return the converted number with correct sign
}
int main() {
const char *input1 = "42 00";
const char *input2 = "-29";
printf("Input 1: %d\n", convert_to_decimal(input1));
printf("Input 2: %d\n", convert_to_decimal(input2));
return 0;
}
```
当你运行这个程序,它会输出:
```
Input 1: 42
Input 2: -29
```
阅读全文
相关推荐
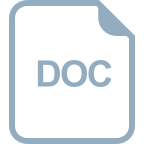
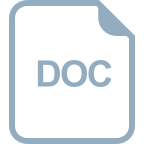
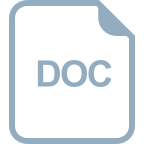






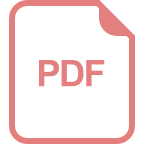








