利用EF6进行复杂数据关联与操作
发布时间: 2024-02-22 19:51:29 阅读量: 30 订阅数: 24 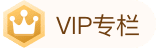
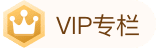
# 1. 理解EF6数据关联的基础知识
## 1.1 EF6简介
Entity Framework(EF)是微软公司推出的一种面向.NET应用程序的对象关系映射(ORM)框架。它提供了将数据库中的数据映射到.NET对象的功能,并支持LINQ查询。EF6是Entity Framework的一个版本,它提供了许多便捷的特性来简化数据访问层的开发。
## 1.2 数据关联的基本概念
在使用EF6进行数据操作时,理解数据关联的基本概念是非常重要的。数据关联是指实体之间的关系,如一对一、一对多、多对多等。
## 1.3 EF6中的数据关联实现方式
EF6通过导航属性和外键关联来实现数据关联,开发者可以通过这些方式来定义实体之间的关系,并实现对应的操作。
以上是第一章的内容,接下来进入第二章内容的编写。
# 2. 实现EF6复杂数据关联
在本章中,我们将深入探讨如何使用EF6进行复杂数据关联操作。我们将介绍单一关联与多重关联的概念,并探讨一对一、一对多和多对多关联的实现方法。最后,我们会讨论如何进行复杂关系的数据建模和关联实现。让我们开始吧!
### 2.1 单一关联与多重关联
#### 单一关联
单一关联指的是两个实体之间只有一种关联关系,通常使用外键关联(ForeignKey)来实现。在EF6中,可以通过配置实体的导航属性和外键属性来表示单一关联关系。
```python
# 示例代码
class Order:
public int OrderId { get; set; }
public string OrderName { get; set; }
public int CustomerId { get; set; }
[ForeignKey("CustomerId")]
public Customer Customer { get; set; }
class Customer:
public int CustomerId { get; set; }
public string CustomerName { get; set; }
```
#### 多重关联
多重关联指的是两个实体之间存在多种不同的关联关系,通常使用多个外键来实现。在EF6中,可以通过配置多个导航属性和外键属性来表示多重关联关系。
```python
# 示例代码
class Order:
public int OrderId { get; set; }
public string OrderName { get; set; }
public int CustomerId { get; set; }
public int ProductId { get; set; }
[ForeignKey("CustomerId")]
public Customer Customer { get; set; }
[ForeignKey("ProductId")]
public Product Product { get; set; }
class Product:
public int ProductId { get; set; }
public string ProductName { get; set; }
class Customer:
public int CustomerId { get; set; }
public string CustomerName { get; set; }
```
### 2.2 一对一、一对多、多对多关联的实现
#### 一对一关联
一对一关联指的是每个实体对象在关联关系中都只能对应另一个实体对象。在EF6中,可以通过配置实体的导航属性和外键属性来表示一对一关联关系。
```python
# 示例代码
class Address:
public int AddressId { get; set; }
public string Street { get; set; }
class Employee:
public int EmployeeId { get; set; }
public string EmployeeName { get; set; }
public int AddressId { get; set; }
public Address Address { get; set; }
```
#### 一对多关联
一对多关联指的是一个实体对象可以对应多个另一个实体对象。在EF6中,可以通过配置实体的导航属性和外键属性来表示一对多关联关系。
```python
# 示例代码
class Department:
public int DepartmentId { get; set; }
public string DepartmentName { get; set; }
public List<Employee> Employees { get; set; }
class Employee:
public int EmployeeId { get; set; }
public string EmployeeName { get; set; }
public int DepartmentId { get; set; }
public Department Department { get; set; }
```
#### 多对多关联
多对多关联指的是两个实体对象之间存在多对多的关联关系。在EF6中,可以通过配置中间表来表示多对多关联关系。
```python
# 示例代码
class Student:
public int StudentId { get; set; }
public string StudentName { get; set; }
public List<Course> Courses { get; set; }
class Course:
public int CourseId { get; set; }
```
0
0
相关推荐








