PyQt5中的绘图和图形效果
发布时间: 2024-02-24 06:50:58 阅读量: 15 订阅数: 17 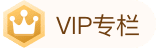
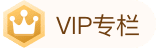
# 1. PyQt5中的绘图基础
## 1.1 PyQt5绘图工具简介
PyQt5是一个功能强大的Python库,它包含了丰富的绘图工具,可以用于创建各种图形和图像效果。在本节中,我们将简要介绍PyQt5中的绘图工具,以及如何利用这些工具进行基本的绘图操作。
在PyQt5中,可以使用QPainter对象进行绘图操作。QPainter提供了丰富的绘图方法,可以绘制直线、矩形、椭圆、文本等各种图形元素。此外,还可以利用QPainterPath来绘制复杂的自定义图形,实现更加灵活多样的绘图效果。
绘图过程通常发生在QWidget或QGraphicsView等继承自QPaintDevice的窗口部件上,通过重写其paintEvent方法并在其中创建QPainter对象来实现绘图操作。下面我们将通过示例代码演示如何在PyQt5中进行简单的绘图操作。
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtGui import QPainter, QPen, QColor
from PyQt5.QtCore import Qt
class DrawingExample(QWidget):
def __init__(self):
super().__init__()
def paintEvent(self, event):
painter = QPainter(self)
painter.setPen(QPen(QColor(Qt.black), 2, Qt.SolidLine))
painter.drawLine(20, 40, 250, 40)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = DrawingExample()
window.setGeometry(100, 100, 300, 200)
window.setWindowTitle('Drawing Example')
window.show()
sys.exit(app.exec_())
```
在上面的示例中,我们创建了一个继承自QWidget的窗口部件类DrawingExample,并重写了其paintEvent方法。在paintEvent方法中,我们创建了一个QPainter对象,并利用setPen方法设置了画笔的颜色、线宽和线型,然后调用drawLine方法绘制了一条直线。最后,我们创建了应用实例并显示了窗口部件,从而实现了简单的绘图操作。
通过以上示例,我们可以看到PyQt5中进行简单绘图操作的基本流程和方法调用,为后续的绘图应用打下了基础。接下来,我们将继续介绍如何在PyQt5中绘制更加丰富多彩的图形元素。
# 2. PyQt5中的图形效果
### 2.1 添加阴影效果
在PyQt5中,我们可以通过设置阴影效果为图形添加更加立体的视觉效果。下面是一个简单的例子,演示如何在PyQt5中为图形添加阴影效果。
```python
import sys
from PyQt5.QtWidgets import QApplication, QGraphicsDropShadowEffect, QGraphicsView, QGraphicsScene, QGraphicsRectItem, QGraphicsEllipseItem
from PyQt5.QtGui import QColor
from PyQt5.QtCore import Qt
if __name__ == '__main__':
app = QApplication(sys.argv)
view = QGraphicsView()
scene = QGraphicsScene()
view.setScene(scene)
# 添加矩形
rect = QGraphicsRectItem(50, 50, 100, 100)
rect.setBrush(QColor(255, 0, 0))
scene.addItem(rect)
# 设置阴影效果
shadow = QGraphicsDropShadowEffect()
shadow.setBlurRadius(8)
shadow.setColor(QColor(0, 0, 0, 160))
shadow.setOffset(4, 4)
rect.setGraphicsEffect(shadow)
# 添加椭圆
ellipse = QGraphicsEllipseItem(200, 50, 100, 100)
ellipse.setBrush(QColor(0, 0, 255))
scene.addItem(ellipse)
# 设置阴影效果
shadow = QGraphicsDropShadowEffect()
shadow.setBlurRadius(8)
shadow.setColor(QColor(0, 0, 0, 160))
shadow.setOffset(4, 4)
ellipse.setGraphicsEffect(shadow)
view.show()
sys.exit(app.exec_())
```
在这个例子中,我们使用了`QGraphicsDropShadowEffect`类为矩形和椭圆添加了阴影效果。可以通过设置`blurRadius`、`color`和`offset`等属性来调整阴影的效果。
运行代码后,可以看到矩形和椭圆都具有立体感的阴影效果。
### 2.2 使用渐变填充图形
PyQt5提供了丰富的渐变填充效果,我们可以通过设置不同类型的渐变来为图形添加丰富多彩的效果。下面是一个简单的例子,演示如何在PyQt5中为图形使用渐变填充。
```python
import sys
from PyQt5.QtWidgets import QApplication, QGraphicsView, QGraphicsScene, QGraphicsRectItem, QGraphicsEllipseItem
from PyQt5.QtGui import QLinearGradient, QBrush, QColor
from PyQt5.QtCore import Qt
if __name__ == '__main__':
app = QApplication(sys.argv)
view = QGraphicsView()
scene = QGraphicsScene()
view.setScene(scene)
# 添加矩形
rect = QGraphicsRectItem(50, 50, 100, 100)
gradient = QLinearGradient(0, 0, 100, 100)
gradient.setColorAt(0, QColor(255, 0, 0))
gradient.setColorAt(0.5, QColor(255, 255, 0))
gradient.setColorAt(1, QColor(255, 0, 255))
rect.setBrush(QBrush(gradient))
scene.addItem(rect)
# 添加椭圆
ellipse = QGraphicsEllipseItem(200, 50, 100, 100)
gradient = QLinearGradient(0, 0, 100, 100)
gradient.setColorAt(0, QColor(0, 0, 255))
gradient.setColorAt(0.5, QColor(0, 255, 255))
gradient.setColorAt(1, QColor(0, 255, 0))
ellipse.setBrush(QBrush(gradient))
scene.addItem(ellipse)
view.show()
sys.exit(app.exec_())
```
在这个例子中,我们使用了`QLinearGradient`类创建了线性渐变,并通过设置不同位置的颜色来实现丰富多彩的渐变填充效果。运行代码后,可以看到矩形和椭圆都具有渐变填充效果。
### 2.3 图形旋转和缩放
在PyQt5中,我们可以通过设置`setRotation`和`setScale`等方法来对图形进行旋转和缩放。下面是一个简单的例子,演示如何在PyQt5中对图形进行旋转和缩放操作。
```python
import sys
fro
```
0
0
相关推荐
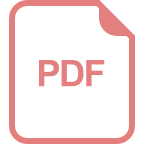
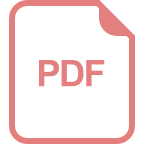
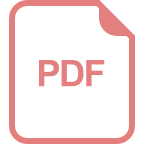
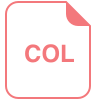
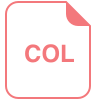
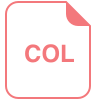
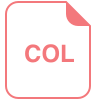
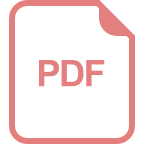