Selenium-web自动化高级应用:使用Selenium进行基于行为驱动开发(BDD)的测试
发布时间: 2024-01-20 08:31:49 阅读量: 37 订阅数: 44 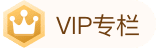
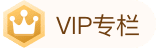
# 1. Selenium-web自动化测试基础
## 1.1 Selenium的概念与原理
Selenium是一个自动化Web应用程序测试工具,主要用于自动化测试的目的。它可以直接在浏览器中模拟用户的操作,支持多种浏览器,并可以运行在多个平台上。
### Selenium的原理
Selenium包括三个主要组件:
- Selenium IDE: 一个Firefox插件,用于用户录制和回放测试用例。
- Selenium WebDriver: 一个用于编写自动化测试脚本的工具,支持多种编程语言。
- Selenium Grid: 用于并行执行测试。
## 1.2 Selenium WebDriver的基本用法
Selenium WebDriver是Selenium的一个关键组件,它会直接控制浏览器,模拟用户操作。下面是一个简单的Python示例:
```python
from selenium import webdriver
# 创建一个新的Chrome浏览器实例
driver = webdriver.Chrome()
# 打开网页
driver.get("http://www.example.com")
# 在搜索框中输入内容
search_box = driver.find_element_by_name("q")
search_box.send_keys("Selenium")
# 提交搜索
search_box.submit()
# 关闭浏览器
driver.quit()
```
## 1.3 编写简单的Selenium自动化测试案例
下面是一个简单的Selenium测试案例,使用Python和unittest框架:
```python
import unittest
from selenium import webdriver
class SimpleSeleniumTest(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
def test_search(self):
self.driver.get("http://www.example.com")
search_box = self.driver.find_element_by_name("q")
search_box.send_keys("Selenium")
search_box.submit()
self.assertIn("Selenium", self.driver.title)
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()
```
这段代码会打开Chrome浏览器,导航至指定网页,搜索关键词"Selenium",并断言页面标题中包含"Selenium"。
以上就是关于Selenium-web自动化测试基础的内容。
# 2. 行为驱动开发(BDD)和Selenium
### 2.1 什么是行为驱动开发(BDD)
行为驱动开发(BDD)是一种软件开发方法论,旨在通过通用的业务语言(如Gherkin)描述应用程序的行为,从而促进开发团队、测试团队和业务领域专家之间的沟通和理解。BDD强调需求和行为的可执行规范,并通过自动化测试来验证软件是否按照预期行为运行。
### 2.2 BDD与Selenium的结合优势
Selenium是一款功能强大的Web自动化测试工具,能够模拟用户的操作行为,例如点击、输入、提交表单等。将BDD与Selenium结合使用可带来以下优势:
- **可读性强**: BDD使用自然语言描述业务需求和行为,使得非技术人员也能理解测试场景。这样,开发人员、测试人员和业务人员可以直接参与测试用例的编写和维护,减少沟通成本。
- **自动化测试**: 结合Selenium,可以将BDD中描述的测试场景转化为具体的自动化测试脚本。这样可以提高测试效率,减少测试人员的工作量,并且能够快速有效地进行回归测试。
- **高可靠性**: 使用BDD框架编写的测试脚本能够更好地关注业务需求和行为,以及应用程序的真实功能。通过Selenium的自动化测试,可以确保测试的准确性和可靠性。
- **持续集成**: BDD和Selenium的结合可以使自动化测试与持续集成流程紧密结合,形成对代码变更的快速反馈机制,帮助开发团队及时发现潜在的问题,并加快软件交付速度。
### 2.3 使用BDD框架编写Selenium自动化测试
在结合BDD和Selenium进行自动化测试时,我们可以使用一些流行的BDD框架,如Cucumber、Behave、SpecFlow等。这些框架提供了框架特定的语法和结构,可用于描述测试场景、步骤和断言。
以下是一个使用Cucumber框架和Selenium WebDriver编写的示例代码:
```java
Feature: User Login
As a registered user
I want to login to the application
So that I can access my account
Scenario: Successful login
Given I am on the login page
When I enter username "john" and password "password"
And I click on the login button
Then I should be redirected to the dashboard page
And I should see the message "Welcome, John!"
Scenario: Invalid login
Given I am on the login page
When I enter invalid username "johndoe" and password "123456"
And I click on the login button
Then I should see the error message "Invalid username or password"
// Step Definitions
public class StepDefinitions {
WebDriver driver;
@Given("^I am on the login page$")
public void navigateToLoginPage() {
driver = new ChromeDriver();
driver.get("https://example.com/login");
}
@When("^I enter username \"([^\"]*)\" and password \"([^\"]*)\"$")
public void enterCredentials(String username, String password) {
WebElement usernameInput = driver.findElement(By.id("username"));
WebElement passwordInput = driver.findElement(By.id("password"));
WebElement loginButton = driver.findElement(By.id("loginButton"));
usernameInput.sendKeys(username);
passwordInput.sendKeys(password);
loginButton.click();
}
@Then("^I should be redirected to the dashboard page$")
public void verifyDashboardPage() {
String currentUrl = driver.getCurrentUrl();
Assert.assertEquals("https://example.com/dashboard", currentUrl);
}
@Then("^I should see the message \"([^\"]*)\"$")
public void verifyWelcomeMessage(String message) {
WebElement welcomeMessage = driver.findElement(By.className("welcome-message"));
Assert.assertEquals(message, welcomeMessage.getText());
}
@Then("^I should see the error message \"([^\"]*)\"$")
public void verifyErrorMessage(String message) {
WebElement errorMessage = driver.findElement(By.className("error-message"));
Assert.assertEquals(message, errorMessage.getText());
}
@After
public void closeBrowser() {
driver.quit();
}
}
```
上述示例中,我们通过Cucumber框架定义了两个测试场景:成功登录和无效登录。每个场景都由多个Given、When、Then步骤组成。通过Step Definitions类中的方法,我们将这些步骤与Selenium WebDriver的操作进行关联,如浏览器导航、元素定位和操作、断言验证等。
通过运行上述代码,Cucumber框架会逐步执行测试场景,并生成相应的报告和日志,以帮助开发团队定位和修复问题。
这就是使用BDD框架编写Selenium自动化测试的基本流程。通过合理使用BDD和Sele
0
0
相关推荐
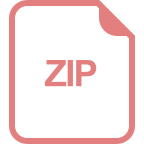
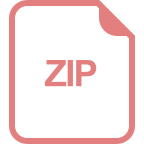
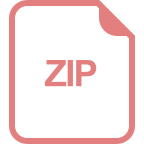
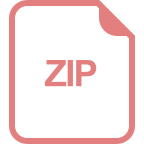
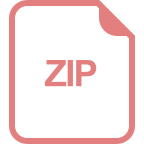
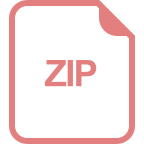
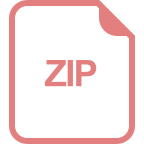
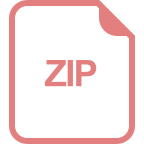
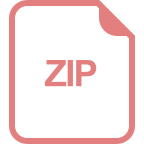