【Eclipse连接MySQL数据库实战指南】:从小白到高手,一文搞定
发布时间: 2024-07-17 04:52:25 阅读量: 30 订阅数: 28 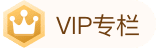
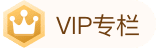

# 1. Eclipse连接MySQL数据库基础
### 1.1 Eclipse简介
Eclipse是一个流行的集成开发环境(IDE),广泛用于Java开发。它提供了丰富的功能,包括代码编辑、调试和数据库连接。
### 1.2 MySQL简介
MySQL是一个开源的关系型数据库管理系统(RDBMS),以其高性能、可靠性和可扩展性而闻名。它广泛用于Web应用程序和数据分析。
# 2. Eclipse连接MySQL数据库的实践步骤
### 2.1 创建Eclipse项目和配置MySQL驱动
**创建Eclipse项目**
1. 打开Eclipse IDE,点击“File”>“New”>“Java Project”。
2. 在“Project Name”中输入项目名称,例如“EclipseMySQLDemo”。
3. 选择“JavaSE-1.8”或更高版本的执行环境。
4. 点击“Finish”创建项目。
**配置MySQL驱动**
1. 右键单击项目,选择“Build Path”>“Configure Build Path”。
2. 在“Libraries”选项卡中,点击“Add External JARs”。
3. 浏览并选择MySQL JDBC驱动程序的JAR文件(例如:mysql-connector-java-8.0.30.jar)。
4. 点击“OK”保存更改。
### 2.2 建立数据库连接和执行SQL语句
**建立数据库连接**
1. 在项目中创建一个新的Java类,例如“MySQLConnection.java”。
2. 导入必要的MySQL JDBC库:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
```
3. 定义连接数据库的常量:
```java
private static final String URL = "jdbc:mysql://localhost:3306/test";
private static final String USER = "root";
private static final String PASSWORD = "password";
```
4. 在`main`方法中建立数据库连接:
```java
public static void main(String[] args) {
try {
Connection conn = DriverManager.getConnection(URL, USER, PASSWORD);
System.out.println("Connected to MySQL database successfully!");
} catch (SQLException e) {
e.printStackTrace();
}
}
```
**执行SQL语句**
1. 使用`Statement`对象执行SQL语句:
```java
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM users");
```
2. 遍历查询结果:
```java
while (rs.next()) {
System.out.println(rs.getInt("id") + " " + rs.getString("name") + " " + rs.getDate("created_at"));
}
```
### 2.3 处理查询结果和异常
**处理查询结果**
* 使用`ResultSet`对象获取查询结果。
* 使用`getXXX()`方法获取特定列的值(例如:`getInt()`、`getString()`)。
* 遍历`ResultSet`对象以获取所有结果行。
**处理异常**
* 使用`try-catch`块捕获`SQLException`。
* 打印异常堆栈跟踪以进行调试。
* 根据异常类型采取适当的措施,例如重新连接数据库或回滚事务。
**代码示例**
```java
try {
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM users");
while (rs.next()) {
System.out.println(rs.getInt("id") + " " + rs.getString("name") + " " + rs.getDate("created_at"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
}
```
# 3. Eclipse连接MySQL数据库的常见问题及解决
### 3.1 连接失败或超时
**问题描述:**
在尝试连接MySQL数据库时,出现连接失败或超时错误。
**可能原因:**
* MySQL服务未启动或未监听端口。
* Eclipse中配置的数据库连接参数不正确。
* 网络连接问题,如防火墙或代理服务器阻止连接。
**解决方案:**
* 检查MySQL服务是否正在运行,并确保其监听指定的端口。
* 仔细检查Eclipse中的数据库连接配置,包括主机、端口、用户名、密码和数据库名称。
* 检查网络连接,确保没有防火墙或代理服务器阻止连接。
### 3.2 SQL语句执行错误
**问题描述:**
执行SQL语句时,出现语法错误、表不存在或其他执行错误。
**可能原因:**
* SQL语句语法不正确。
* 表或列不存在。
* 数据类型不匹配。
* 权限不足。
**解决方案:**
* 仔细检查SQL语句的语法,确保其符合MySQL语法。
* 确认表和列是否存在,并具有正确的名称和类型。
* 检查数据类型是否匹配,并根据需要进行转换。
* 确保用户具有执行SQL语句所需的权限。
### 3.3 数据类型转换异常
**问题描述:**
在处理查询结果时,出现数据类型转换异常。
**可能原因:**
* 查询结果中的数据类型与Java代码中声明的变量类型不匹配。
* MySQL和Java之间的数据类型转换存在差异。
**解决方案:**
* 确保Java代码中声明的变量类型与查询结果中的数据类型匹配。
* 使用适当的转换方法将MySQL数据类型转换为Java数据类型。
* 例如,使用`ResultSet.getInt()`获取整数,`ResultSet.getString()`获取字符串。
# 4. Eclipse连接MySQL数据库的进阶应用
### 4.1 使用PreparedStatement防止SQL注入
**背景:**
SQL注入是一种常见的安全漏洞,攻击者可以利用恶意SQL语句来获取或修改数据库中的数据。PreparedStatement是一种预编译的语句,可以有效防止SQL注入。
**原理:**
PreparedStatement在执行前会先进行预编译,将SQL语句中的参数替换为占位符(?)。当执行语句时,再将参数值逐个绑定到占位符上。这样,SQL语句中的参数值就不会被当作SQL语句的一部分来执行,从而避免了SQL注入。
**代码示例:**
```java
// 使用PreparedStatement防止SQL注入
try {
// 创建PreparedStatement
String sql = "SELECT * FROM users WHERE username = ?";
PreparedStatement pstmt = conn.prepareStatement(sql);
// 设置参数
pstmt.setString(1, "admin");
// 执行查询
ResultSet rs = pstmt.executeQuery();
// 处理查询结果
while (rs.next()) {
System.out.println(rs.getString("username"));
}
} catch (SQLException e) {
e.printStackTrace();
}
```
**逻辑分析:**
* 创建PreparedStatement对象,并指定SQL语句。
* 使用`setString()`方法设置参数值。
* 执行查询,获取结果集。
* 遍历结果集,打印用户名。
### 4.2 批量执行SQL语句提高效率
**背景:**
在某些情况下,需要执行大量的SQL语句。逐条执行这些语句会非常耗时。批量执行SQL语句可以显著提高效率。
**原理:**
批量执行SQL语句是指将多个SQL语句合并成一个批处理语句,一次性发送到数据库服务器执行。这样可以减少与数据库服务器的交互次数,从而提高效率。
**代码示例:**
```java
// 批量执行SQL语句提高效率
try {
// 创建Statement对象
Statement stmt = conn.createStatement();
// 添加SQL语句到批处理
stmt.addBatch("INSERT INTO users (username, password) VALUES ('user1', 'pass1')");
stmt.addBatch("INSERT INTO users (username, password) VALUES ('user2', 'pass2')");
stmt.addBatch("INSERT INTO users (username, password) VALUES ('user3', 'pass3')");
// 执行批处理
int[] updateCounts = stmt.executeBatch();
// 处理结果
for (int updateCount : updateCounts) {
System.out.println("更新了 " + updateCount + " 行");
}
} catch (SQLException e) {
e.printStackTrace();
}
```
**逻辑分析:**
* 创建Statement对象。
* 使用`addBatch()`方法将SQL语句添加到批处理。
* 执行批处理,获取更新计数数组。
* 遍历更新计数数组,打印更新的行数。
### 4.3 编写可重用的数据库操作类
**背景:**
在实际开发中,经常需要重复执行一些数据库操作。编写可重用的数据库操作类可以简化代码,提高开发效率。
**原理:**
可重用的数据库操作类封装了常用的数据库操作方法,例如查询、插入、更新和删除。这些方法可以被其他类直接调用,无需重复编写代码。
**代码示例:**
```java
// 编写可重用的数据库操作类
public class DBUtil {
private static Connection conn;
public static void init() {
// 初始化数据库连接
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
}
public static ResultSet query(String sql) {
// 执行查询并返回结果集
try {
Statement stmt = conn.createStatement();
return stmt.executeQuery(sql);
} catch (SQLException e) {
e.printStackTrace();
return null;
}
}
public static int update(String sql) {
// 执行更新并返回受影响的行数
try {
Statement stmt = conn.createStatement();
return stmt.executeUpdate(sql);
} catch (SQLException e) {
e.printStackTrace();
return -1;
}
}
// 其他数据库操作方法...
}
```
**逻辑分析:**
* 初始化数据库连接。
* 提供查询、更新等数据库操作方法。
* 这些方法可以直接被其他类调用,简化代码。
# 5.1 使用连接池优化性能
连接池是一种缓存机制,用于管理和复用数据库连接。它可以显著提高数据库访问的性能,特别是在高并发场景下。
### 连接池的工作原理
连接池本质上是一个连接队列,其中包含已建立的数据库连接。当应用程序需要访问数据库时,它会从连接池中获取一个可用连接。使用完毕后,连接不会被关闭,而是被放回连接池中,供其他线程使用。
### 使用连接池的好处
使用连接池的主要好处包括:
- **减少连接开销:**建立和关闭数据库连接是一个耗时的过程。连接池通过复用连接,减少了连接开销,从而提高了性能。
- **提高并发性:**连接池允许多个线程同时访问数据库,而无需等待新的连接建立。这对于高并发应用程序至关重要。
- **故障隔离:**如果一个连接出现故障,连接池会自动将其从队列中移除,并提供一个新的连接。这有助于防止故障传播到应用程序。
### 在 Eclipse 中使用连接池
Eclipse 提供了 `DataSource` 接口来管理连接池。要使用连接池,需要执行以下步骤:
1. **创建连接池:**使用 `DataSourceFactory` 创建一个 `DataSource` 对象,并配置连接池参数,如最大连接数、空闲时间等。
2. **获取连接:**通过 `DataSource` 对象的 `getConnection()` 方法获取一个数据库连接。
3. **使用连接:**使用连接执行 SQL 语句。
4. **关闭连接:**使用完毕后,调用 `Connection` 对象的 `close()` 方法将连接放回连接池。
### 代码示例
```java
import javax.sql.DataSource;
import javax.sql.DataSourceFactory;
public class ConnectionPoolExample {
public static void main(String[] args) throws Exception {
// 创建连接池
DataSourceFactory factory = DataSourceFactory.newInstance();
DataSource dataSource = factory.createDataSource();
// 获取连接
Connection connection = dataSource.getConnection();
// 使用连接
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM users");
// 关闭连接
connection.close();
}
}
```
0
0
相关推荐
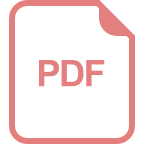
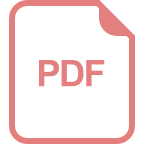





