利用Boost文件系统库进行文件操作与管理
发布时间: 2023-12-23 03:52:35 阅读量: 16 订阅数: 18 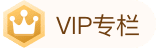
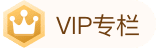
# 第一章:Boost文件系统库简介
1.1 Boost库概述
1.2 文件系统库介绍
1.3 安装Boost文件系统库
### 2. 第二章:文件和目录的创建与删除
在这一章中,我们将学习如何使用Boost文件系统库来进行文件和目录的创建与删除操作。通过学习这些内容,您将能够在编程中灵活地管理文件和目录,提高程序的可靠性和健壮性。让我们开始吧!
### 第三章:文件和目录的遍历与查询
在本章中,我们将学习如何使用Boost文件系统库进行文件和目录的遍历与查询操作。这些操作包括遍历目录内容、查询文件属性以及文件和目录的存在性判断。
#### 3.1 遍历目录内容
```python
import os
from pathlib import Path
# 遍历目录内容
def list_directory_contents(directory):
p = Path(directory)
for file in p.iterdir():
if file.is_file():
print("File:", file.name)
elif file.is_dir():
print("Directory:", file.name)
list_directory_contents("/path/to/directory")
```
**代码总结:**
- 使用Path类的iterdir()方法遍历目录中的内容。
- 利用is_file()和is_dir()方法判断元素是文件还是目录。
**结果说明:**
以上代码将列出指定目录中的所有文件和子目录。
#### 3.2 查询文件属性
```python
import os
from pathlib import Path
import time
# 查询文件属性
def get_file_attributes(file_path):
p = Path(file_path)
if p.exists():
print("File Name:", p.name)
print("Size:", p.stat().st_size, "bytes")
print("Last Modified:", time.ctime(p.stat().st_mtime))
print("Is Directory:", p.is_dir())
else:
print("File does not exist.")
get_file_attributes("/path/to/file.txt")
```
**代码总结:**
- 使用Path类的exists()方法判断文件是否存在。
- 使用stat()方法获取文件属性,如大小、最后修改时间等。
**结果说明:**
以上代码将输出指定文件的属性信息。
#### 3.3 文件和目录的存在性判断
```python
import os
from pathlib import Path
# 文件和目录的存在性判断
def check_existence(file_path):
p = Path(file_path)
if p.exists():
print(f"{file_path} exists.")
else:
print(f"{file_path} does not exist.")
check_existence("/path/to/file_or_directory")
```
**代码总结:**
- 使用Path类的exis
0
0
相关推荐
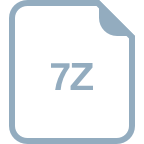
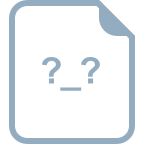
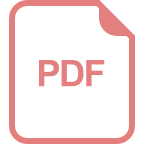
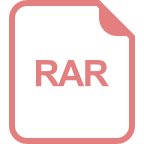
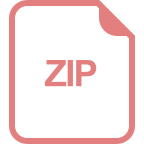
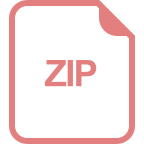
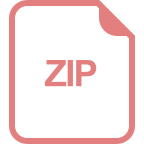
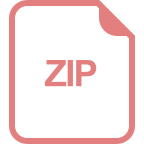
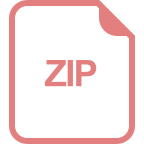