【OpenCV图像显示神器:imshow函数深入解析与实战应用】
发布时间: 2024-08-12 12:31:01 阅读量: 182 订阅数: 25 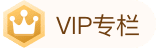
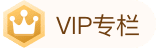
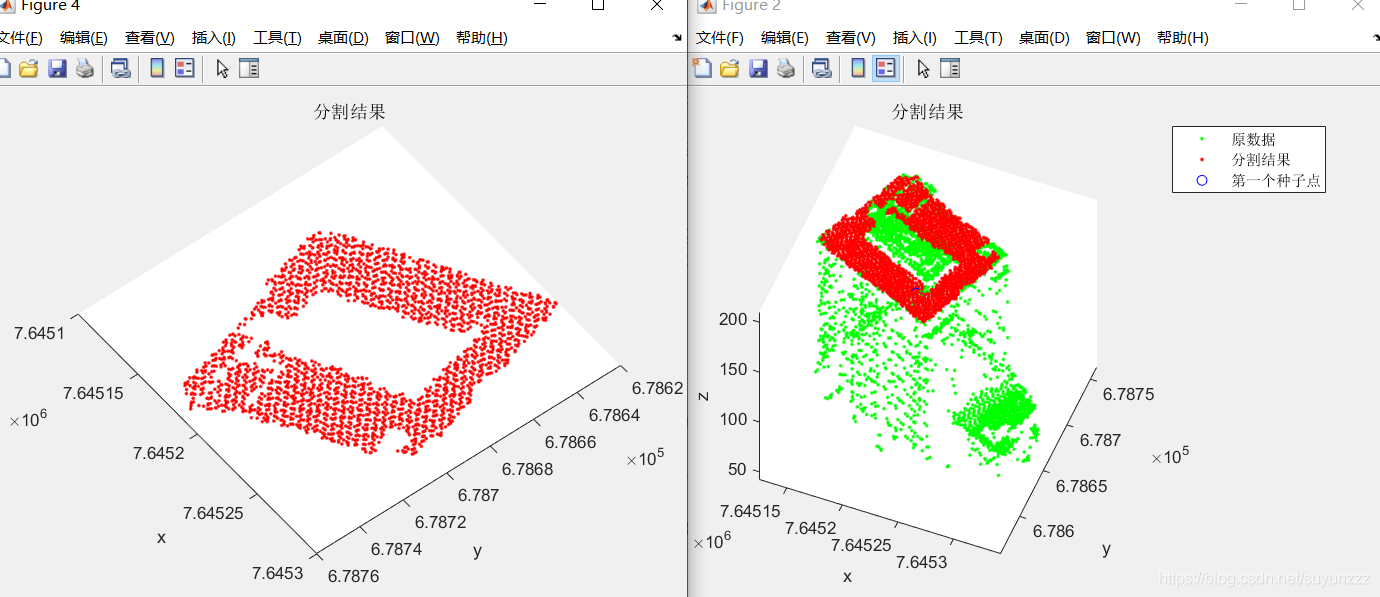
# 1. OpenCV图像显示基础
OpenCV(Open Source Computer Vision Library)是一个开源的计算机视觉库,它提供了广泛的图像处理和计算机视觉算法。图像显示是计算机视觉中一项基本任务,OpenCV提供了`imshow()`函数来轻松显示图像。
`imshow()`函数的语法结构为:`imshow(winname, image)`,其中`winname`是图像窗口的名称,`image`是需要显示的图像。`imshow()`函数会创建一个图像窗口并显示图像。如果图像窗口已经存在,则会更新窗口中的图像。
# 2. imshow函数的原理与实现
### 2.1 imshow函数的语法结构
```cpp
void imshow(const string& winname, const Mat& image);
```
| 参数 | 描述 |
|---|---|
| `winname` | 图像窗口的名称 |
| `image` | 要显示的图像 |
### 2.2 imshow函数的内部机制
imshow函数的内部实现主要分为以下几个步骤:
1. **创建图像窗口:**调用`namedWindow`函数创建指定名称的图像窗口。如果窗口已存在,则激活该窗口。
2. **图像数据复制:**将`image`中的图像数据复制到窗口的图像缓冲区中。
3. **图像显示:**调用`imshow`函数将图像缓冲区中的图像显示在窗口中。
**代码块:**
```cpp
void imshow(const string& winname, const Mat& image) {
// 创建图像窗口
namedWindow(winname);
// 图像数据复制
Mat dstImage;
image.copyTo(dstImage);
// 图像显示
imshow(winname, dstImage);
}
```
**代码逻辑分析:**
* `namedWindow`函数创建图像窗口,如果窗口已存在,则激活该窗口。
* `image.copyTo(dstImage)`将`image`中的图像数据复制到`dstImage`中,避免直接修改原始图像。
* `imshow`函数将`dstImage`中的图像显示在窗口中。
### 2.3 imshow函数的性能优化
imshow函数的性能优化主要集中在以下几个方面:
1. **图像数据复制优化:**使用`Mat::clone()`函数克隆图像数据,避免不必要的内存复制。
2. **图像显示优化:**使用`OpenGL`或`DirectX`等图形库加速图像显示。
3. **窗口管理优化:**使用`setWindowProperty`函数调整窗口大小和位置,减少窗口创建和销毁的开销。
**代码块:**
```cpp
void imshow(const string& winname, const Mat& image) {
// 图像数据复制优化
Mat dstImage = image.clone();
// 图像显示优化
setWindowProperty(winname, CV_WINDOW_OPENGL, true);
// 窗口管理优化
setWindowProperty(winname, CV_WINDOW_NORMAL, true);
// 图像显示
imshow(winname, dstImage);
}
```
**代码逻辑分析:**
* `image.clone()`函数克隆图像数据,避免不必要的内存复制。
* `setWindowProperty`函数设置窗口属性,启用`OpenGL`加速。
* `setWindowProperty`函数设置窗口属性,调整窗口大小和位置。
# 3. imshow函数的应用技巧
### 3.1 图像窗口的创建和销毁
imshow函数创建了一个图像窗口,用于显示图像。窗口的标题默认为图像的文件名,可以通过`cv2.namedWindow()`函数自定义窗口标题。
```python
import cv2
# 创建一个图像窗口
cv2.imshow("My Image", image)
# 等待用户按下任意键关闭窗口
cv2.waitKey(0)
cv2.destroyAllWindows()
```
`cv2.destroyAllWindows()`函数销毁所有创建的图像窗口。
### 3.2 图像的缩放和裁剪
#### 缩放
imshow函数可以通过`cv2.resize()`函数缩放图像。缩放比例可以通过`fx`和`fy`参数指定,分别表示x轴和y轴的缩放因子。
```python
# 将图像缩小一半
scaled_image = cv2.resize(image, (0, 0), fx=0.5, fy=0.5)
```
#### 裁剪
imshow函数可以通过`cv2.getRectSubPix()`函数裁剪图像。裁剪区域可以通过`rect`参数指定,是一个元组`(x, y, w, h)`,其中`(x, y)`是裁剪区域的左上角坐标,`w`和`h`是裁剪区域的宽度和高度。
```python
# 裁剪图像的中心部分
cropped_image = cv2.getRectSubPix(image, (100, 100), (50, 50))
```
### 3.3 图像的色彩空间转换
imshow函数可以通过`cv2.cvtColor()`函数转换图像的色彩空间。色彩空间可以通过`cv2.COLOR_BGR2GRAY`等常量指定。
```python
# 将图像转换为灰度图像
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
```
# 4. imshow函数的进阶应用
### 4.1 图像叠加和融合
imshow函数不仅可以显示单张图像,还可以叠加和融合多张图像。这在图像处理和计算机视觉中非常有用,例如:
- 图像配准:将两张图像对齐并叠加,以找出它们之间的差异。
- 图像融合:将多张图像融合成一张更具信息量的图像。
- 背景去除:将前景图像叠加到背景图像上,以去除背景。
#### 图像叠加
```cpp
import cv2
# 加载两张图像
image1 = cv2.imread("image1.jpg")
image2 = cv2.imread("image2.jpg")
# 创建一个与两张图像大小相同的黑色图像
blended = np.zeros((image1.shape[0], image1.shape[1], 3), np.uint8)
# 逐像素叠加两张图像
for i in range(image1.shape[0]):
for j in range(image1.shape[1]):
blended[i, j] = image1[i, j] + image2[i, j]
# 显示叠加后的图像
cv2.imshow("Blended Image", blended)
cv2.waitKey(0)
```
**代码逻辑分析:**
1. 加载两张图像并创建一个黑色图像作为叠加目标。
2. 遍历两张图像的像素,将对应的像素值相加并存储到叠加目标中。
3. 显示叠加后的图像。
#### 图像融合
```cpp
import cv2
# 加载两张图像
image1 = cv2.imread("image1.jpg")
image2 = cv2.imread("image2.jpg")
# 设置融合系数(0-1)
alpha = 0.5
# 创建一个与两张图像大小相同的黑色图像
fused = np.zeros((image1.shape[0], image1.shape[1], 3), np.uint8)
# 逐像素融合两张图像
for i in range(image1.shape[0]):
for j in range(image1.shape[1]):
fused[i, j] = (1 - alpha) * image1[i, j] + alpha * image2[i, j]
# 显示融合后的图像
cv2.imshow("Fused Image", fused)
cv2.waitKey(0)
```
**代码逻辑分析:**
1. 加载两张图像并创建一个黑色图像作为融合目标。
2. 设置融合系数,表示两张图像在融合结果中的权重。
3. 遍历两张图像的像素,将对应的像素值按比例融合并存储到融合目标中。
4. 显示融合后的图像。
### 4.2 图像标注和注释
imshow函数还可以用于图像标注和注释,这在图像分析和可视化中非常有用。
#### 图像标注
```cpp
import cv2
# 加载图像
image = cv2.imread("image.jpg")
# 创建一个矩形框的坐标
x, y, w, h = 100, 100, 200, 200
# 在图像上绘制矩形框
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 显示标注后的图像
cv2.imshow("Annotated Image", image)
cv2.waitKey(0)
```
**代码逻辑分析:**
1. 加载图像并定义矩形框的坐标。
2. 使用`cv2.rectangle()`函数在图像上绘制矩形框,指定颜色和线宽。
3. 显示标注后的图像。
#### 图像注释
```cpp
import cv2
# 加载图像
image = cv2.imread("image.jpg")
# 创建一个文本注释
text = "This is an image"
# 在图像上绘制文本注释
cv2.putText(image, text, (100, 100), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
# 显示注释后的图像
cv2.imshow("Annotated Image", image)
cv2.waitKey(0)
```
**代码逻辑分析:**
1. 加载图像并定义文本注释。
2. 使用`cv2.putText()`函数在图像上绘制文本注释,指定字体、大小、颜色和线宽。
3. 显示注释后的图像。
# 5. imshow函数的实战应用
### 5.1 图像处理流水线
在实际应用中,imshow函数通常被集成到图像处理流水线中,作为图像处理结果的可视化工具。一个典型的图像处理流水线可能包含以下步骤:
- **图像获取:**从文件、摄像头或其他来源获取图像。
- **图像预处理:**对图像进行预处理,例如调整大小、转换颜色空间或应用滤波器。
- **图像处理:**对图像进行处理,例如目标检测、图像分割或特征提取。
- **图像显示:**使用imshow函数将处理后的图像可视化。
- **结果分析:**分析可视化的图像,并根据需要进行进一步的处理。
### 5.2 图像识别与分类
imshow函数在图像识别和分类任务中发挥着至关重要的作用。通过将图像可视化,可以方便地识别和分类图像中的对象。
**图像识别:**
```python
import cv2
# 加载图像
image = cv2.imread('image.jpg')
# 显示图像
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析:**
- `cv2.imread('image.jpg')`:从文件中加载图像。
- `cv2.imshow('Image', image)`:显示图像,窗口标题为“Image”。
- `cv2.waitKey(0)`:等待用户按任意键关闭窗口。
- `cv2.destroyAllWindows()`:销毁所有打开的窗口。
**图像分类:**
```python
import cv2
import numpy as np
# 加载图像
image = cv2.imread('image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 二值化图像
thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)[1]
# 查找轮廓
cnts = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cnts = cnts[0] if len(cnts) == 2 else cnts[1]
# 遍历轮廓并显示
for c in cnts:
# 计算轮廓的面积
area = cv2.contourArea(c)
# 如果面积大于某个阈值,则认为是对象
if area > 100:
# 计算轮廓的边界矩形
x, y, w, h = cv2.boundingRect(c)
# 在图像上绘制边界矩形
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 显示图像
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析:**
- `cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)`:将图像转换为灰度图像。
- `cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)[1]`:对灰度图像进行二值化,阈值设为 127。
- `cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)`:查找图像中的轮廓。
- `for c in cnts:`:遍历轮廓。
- `area = cv2.contourArea(c)`:计算轮廓的面积。
- `if area > 100:`:如果面积大于阈值,则认为是对象。
- `x, y, w, h = cv2.boundingRect(c)`:计算轮廓的边界矩形。
- `cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)`:在图像上绘制边界矩形。
# 6. imshow函数的性能优化
### 6.1 图像显示的优化策略
**1. 减少不必要的窗口创建和销毁**
频繁创建和销毁窗口会消耗大量的系统资源。为了优化性能,应尽可能复用现有的窗口,而不是每次显示图像时都创建新的窗口。
**2. 使用适当的图像格式**
不同的图像格式具有不同的处理效率。对于实时显示的图像,建议使用轻量级的格式,如 JPEG 或 PNG。
**3. 优化图像大小**
显示大尺寸图像会占用更多的内存和处理时间。在显示图像之前,应根据需要调整图像大小,以减少资源消耗。
**4. 启用硬件加速**
现代计算机通常配备了图形处理单元 (GPU),可以加速图像处理任务。通过启用硬件加速,可以显著提高图像显示的性能。
### 6.2 内存管理与资源释放
**1. 避免内存泄漏**
图像显示过程中会分配大量的内存,如果处理不当,可能会导致内存泄漏。确保在不再需要图像时及时释放内存,以防止系统资源耗尽。
**2. 使用共享内存**
在多线程环境中,图像数据可能需要在多个线程之间共享。使用共享内存机制可以避免不必要的内存复制,提高性能。
**3. 优化内存分配策略**
选择合适的内存分配策略可以减少内存碎片,提高内存利用率。考虑使用内存池或内存对齐技术来优化内存分配。
**4. 及时释放资源**
当图像显示窗口不再需要时,应及时释放与之关联的所有资源,包括窗口句柄、图像数据和共享内存。这有助于防止资源泄漏和系统不稳定。
0
0
相关推荐








