使用ThinkPHP5.1构建RESTful API
发布时间: 2023-12-16 06:57:03 阅读量: 12 订阅数: 14 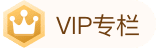
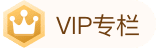
## 1. Introduction
### 1.1 What is RESTful API?
RESTful API, also known as Representational State Transfer, is an architectural style for designing networked applications. It is based on a set of principles and constraints that allow for the scalability, simplicity, and flexibility of web services. RESTful APIs use standard HTTP methods such as GET, POST, PUT, and DELETE to perform operations on resources.
### 1.2 Why use ThinkPHP5.1 for building RESTful API?
ThinkPHP5.1 is a PHP-based framework that provides a solid foundation for building RESTful APIs. It follows the MVC (Model-View-Controller) architectural pattern and offers a wide range of features and utilities for rapid development. Some key reasons to use ThinkPHP5.1 for building RESTful APIs include:
- Robust routing system: ThinkPHP5.1 provides a flexible routing mechanism for defining API endpoints and mapping them to controller actions.
- Database support: It offers seamless integration with various database systems, making it easy to perform CRUD operations on data.
- Security features: ThinkPHP5.1 provides built-in protection against SQL injection and XSS (Cross-Site Scripting) attacks, ensuring the security of your API.
- Extensibility: The framework supports the use of plugins and extensions, allowing developers to enhance the functionality of their APIs.
- Active community: ThinkPHP5.1 has a large and active community of developers who contribute to its documentation, plugins, and provide support.
### 1.3 Brief overview of ThinkPHP5.1 framework
ThinkPHP5.1 is a lightweight, high-performance PHP framework that adheres to modern design patterns and coding standards. It follows the principles of convention over configuration, allowing developers to focus on writing business logic rather than dealing with tedious configurations.
Some key features of ThinkPHP5.1 include:
- MVC architecture: ThinkPHP5.1 follows the Model-View-Controller pattern, separating business logic from presentation and data handling.
- Powerful ORM: It includes an Object-Relational Mapping (ORM) library that simplifies database operations and provides support for multiple database systems.
- Template engine: ThinkPHP5.1 comes with a template engine that enables the separation of HTML/CSS/JavaScript from PHP code, improving code maintainability.
- Caching: The framework offers caching mechanisms to enhance the performance of database queries and application data.
- Error handling: ThinkPHP5.1 provides robust error handling and logging mechanisms to help debug and resolve issues.
In the following sections, we will learn how to set up the environment, create APIs using ThinkPHP5.1, implement authentication and authorization, handle errors, and deploy the API to a production environment.
## 2. Setting Up the Environment
### Installing and Configuring ThinkPHP5.1
To begin developing RESTful APIs with ThinkPHP5.1, you first need to install and configure the framework. Here are the steps to get started:
1. **Install Composer:**
Download and install Composer, a dependency manager for PHP, from [getcomposer.org](https://getcomposer.org/download/).
2. **Create ThinkPHP5.1 Project:**
Use Composer to create a new ThinkPHP5.1 project by running the following command in your terminal or command prompt:
```bash
composer create-project topthink/think=5.1.* myproject
```
Replace `myproject` with your desired project name.
3. **Configure Database Connection:**
Update the database configuration in the `config/database.php` file to connect ThinkPHP to your database.
### Setting Up Database Connection
In the `config/database.php` file, configure the database connection settings according to your environment. For example:
```php
return [
// ...
'connection' => [
// Database type
'type' => 'mysql',
// Host address
'hostname' => 'localhost',
// Database name
'database' => 'my_database',
// Username
'username' => 'root',
// Password
'password' => 'password',
// ...
],
];
```
### Creating Project Structure
Once the project is created and the database connection is configured, you can start setting up the project structure for your RESTful API development. Consider organizing your project into directories such as `controller`, `model`, `view`, and `route` to keep your codebase clean and structured.
By following these steps, you can set up the environment for developing RESTful APIs using ThinkPHP5.1.
### 3. Creating APIs
As we delve into the process of creating APIs using ThinkPHP5.1, it's crucial to understand the principles of RESTful API design. REST (Representational State Transfer) is an architectural style that defines a set of constraints to be used for creating web services. These constraints include using a client-server architecture, statelessness, cacheability, and a uniform interface, among others.
#### Understanding RESTful API design principles
RESTful APIs are designed to be resource-based, meaning that they operate on resources such as users, products, or orders. Each resource is identified by a unique URI, and interactions with the resource are performed using standard HTTP methods such as GET, POST, PUT, and DELETE.
In ThinkPHP5.1, we follow these principles by creating controllers and defining resourceful routes for each API endpoint. For example, a user resource can have endpoints for accessing user details, creating new users, updating user information, and deleting users.
#### Creating routes for API endpoints
In ThinkPHP5.1, routes are defined in the `route/route.php` file. We can create routes for our API endpoints using the `Route::resource()` method, which maps HTTP methods to controller actions for CRUD operations. For instance, the following code defines the routes for a `User` resource:
```php
Route::resource('user', 'user');
```
This single line of code automatically maps HTTP requests to the appropriate controller methods for handling API actions.
#### Implementing CRUD operations
With the routes in place, we can now create controller methods to handle CRUD operations for our API endpoints. For example, the `user` controller would contain
0
0
相关推荐
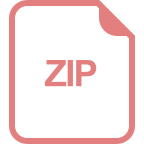
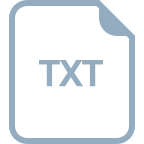
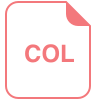
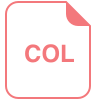
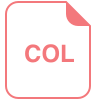
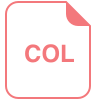

