排序算法全面剖析:从冒泡到快速排序的演进
发布时间: 2024-09-09 19:35:38 阅读量: 44 订阅数: 46 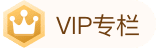
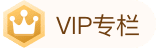
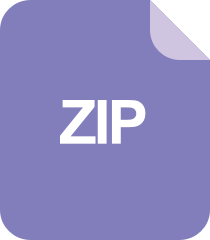
果壳处理器研究小组(Topic基于RISCV64果核处理器的卷积神经网络加速器研究)详细文档+全部资料+优秀项目+源码.zip
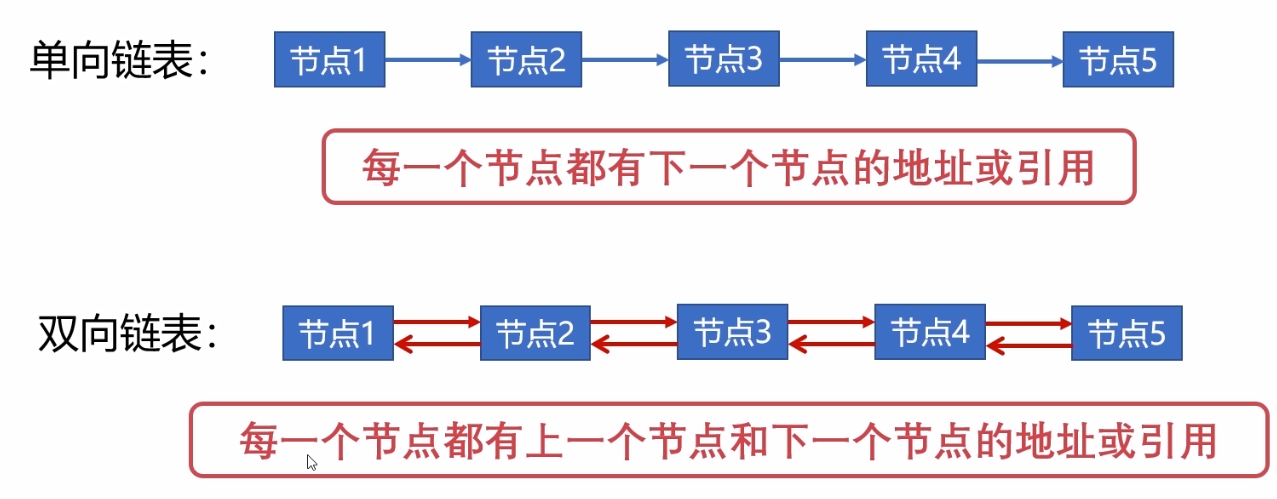
# 1. 排序算法简介与分类
排序算法是计算机科学中不可或缺的一部分,它们在数据处理和分析领域扮演着至关重要的角色。排序算法可以按照不同的标准进行分类,例如稳定性、时间复杂度以及空间复杂度等。了解排序算法的这些分类有助于我们在不同的应用场景中做出合适的选择。
在本章中,我们将简要概述排序算法,并按照它们的工作机制对其进行分类。我们将重点介绍那些常见的排序算法,如冒泡排序、插入排序、选择排序、快速排序等,并探讨它们的优缺点。此外,我们还将引入一些高级排序算法,如归并排序、堆排序和基数排序,这些算法在特定情况下更为高效。
排序算法的分类不仅帮助我们理解各种算法的基本原理,还能够让我们更好地把握算法设计和实现的深层次理念。让我们从排序算法的基础知识开始,逐步深入了解并掌握排序算法的奥秘。接下来的章节中,我们将逐一深入分析每一种排序算法,包括它们的原理、优化技巧以及应用场景。
# 2. 冒泡排序详解
冒泡排序是计算机科学中一个简单直观的排序算法。虽然在算法效率上不是最优,但由于其原理简单,易于实现,在教学和小型数据集的处理中仍然有着广泛的应用。本章将详细解析冒泡排序的原理、实现方法以及常见的优化技巧,并探讨其在实际中的应用场景。
## 2.1 冒泡排序基础
### 2.1.1 算法原理
冒泡排序的基本思想是通过重复遍历待排序的数列,比较每对相邻元素的值,若不符合排序规则(如需从小到大排序,前一个数比后一个数大)就交换它们的位置。遍历数列的工作是重复进行直到没有再需要交换,也就是说该数列已经排序完成。这个算法的名字由来是因为越小(或越大)的元素会经过交换慢慢“浮”到数列的顶端。
### 2.1.2 算法步骤与实现
在实现冒泡排序时,需要注意几个关键点:
1. **外层循环**:控制遍历的次数,对于N个数进行排序,最多需要进行N-1次遍历。
2. **内层循环**:执行实际的比较和交换操作,每一轮遍历都将未排序部分的最大(或最小)值“冒泡”到其应在的位置。
3. **交换操作**:比较相邻元素的大小,若逆序则进行交换。
下面是冒泡排序的伪代码实现:
```pseudocode
function bubbleSort(array):
n = length(array)
repeat
swapped = false
for i = 1 to n-1 inclusive do
if array[i] > array[i+1] then
swap(array[i], array[i+1])
swapped = true
end if
end for
n = n - 1
until not swapped
end function
```
在上述代码中,变量`swapped`用于标记某次遍历中是否发生了交换操作,如果没有交换发生,则说明数组已经完全有序,算法即可结束。
## 2.2 冒泡排序的优化
### 2.2.1 标志位优化法
冒泡排序的一个常见优化是引入标志位,减少不必要的遍历。当一轮遍历中没有发生任何交换时,可以认为数组已经是有序的,因此可以立即结束排序。这种方法可以显著减少排序的时间复杂度,尤其是在待排序数组已经部分有序时。
```python
def optimized_bubble_sort(array):
n = len(array)
while n > 0:
new_n = 0
for i in range(1, n):
if array[i-1] > array[i]:
array[i-1], array[i] = array[i], array[i-1]
new_n = i
n = new_n
```
在上述Python代码实现中,`new_n`记录了最后一次交换发生的位置。由于冒泡排序的特性,我们知道,在`new_n`之后的元素已经是有序的,所以不需要再次进行比较,这样可以大幅减少不必要的比较次数。
### 2.2.2 鸡尾酒排序(双向冒泡排序)
鸡尾酒排序是冒泡排序的一个变种,其基本思想是进行双向的遍历,一轮排序由低到高(传统冒泡排序的方式),再由高到低,这样可以加快排序的速度,尤其是当最小或最大元素远离其最终位置时。
```python
def cocktail_shaker_sort(array):
n = len(array)
swapped = True
start = 0
end = n - 1
while swapped:
swapped = False
for i in range(start, end):
if array[i] > array[i + 1]:
array[i], array[i + 1] = array[i + 1], array[i]
swapped = True
if not swapped: break
swapped = False
end -= 1
for i in range(end - 1, start - 1, -1):
if array[i] > array[i + 1]:
array[i], array[i + 1] = array[i + 1], array[i]
swapped = True
start += 1
```
鸡尾酒排序在每一轮遍历结束时,都会收缩数组的边界。这减少了后续遍历时的比较次数,使得排序更高效。
## 2.3 冒泡排序的变体
### 2.3.1 稳定性分析
冒泡排序是一种稳定的排序算法,因为它不会改变相同元素之间的相对顺序。对于稳定性要求较高的应用场景,冒泡排序即便不是最优的选择,但也是一个考虑的选项。
### 2.3.2 应用场景讨论
尽管冒泡排序在时间效率上不是最优的排序方法,但其简单易懂且易于实现的特性使其在某些特定领域具有一定的优势。例如,在教学中常用于向初学者介绍排序的概念。此外,对于数据量非常小的情况,冒泡排序的性能损耗可以忽略不计,因此在实际应用中也有可能被采用。
在下一章节中,我们将详细探讨插入排序算法,了解其原理、实现和性能,并与冒泡排序进行对比,从而加深对排序算法的理解。
# 3. 插入排序的深入研究
## 3.1 插入排序原理
### 3.1.1 直接插入排序
插入排序是一种简单直观的排序算法。它的工作原理是通过构建有序序列,对于未排序数据,在已排序序列中从后向前扫描,找到相应位置并插入。其基本操作是将一个记录插入到已经排好序的有序表中,从而得到一个新的、记录数增加1的有序表。
在最坏的情况下,插入排序的时间复杂度为O(n^2),它由两个主要部分组成:一个是未排序数据的遍历,另一个是已排序数据的后移。在最好的情况下,即输入数组已经是正序排列的情况下,时间复杂度为O(n)。
以下是直接插入排序的基本步骤:
1. 从第一个元素开始,该元素可以认为已经被排序;
2. 取出下一个元素,在已经排序的元素序列中从后向前扫描;
3. 如果该元素(已排序)大于新元素,将该元素移到下一位置;
4. 重复步骤3,直到找到已排序的元素小于或者等于新元素的位置;
5. 将新元素插入到该位置后;
6. 重复步骤2~5。
代码示例如下:
```python
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
return arr
# 示例
array = [12, 11, 13, 5, 6]
print("Original array is:", array)
sorted_array = insertion_sort(array)
print("Sorted array is:", sorted_array)
```
执行逻辑说明:这段代码实现了一个简单的插入排序算法。它从数组的第二个元素开始,逐个将其与已排序的部分进行比较和插入。如果当前元素比前面的元素小,则将前面的元素向后移动,直到找到适当的位置插入当前元素。
### 3.1.2 希尔排序(缩小增量排序)
希尔排序是插入排序的一种更高效的改进版本,也称为缩小增量排序。希尔排序通过将原来要排序的数据分成若干个子序列,先让每个子序列分别进行直接插入排序,待整个序列中的记录“基本有序”时,再对全体记录进行一次直接插入排
0
0
相关推荐
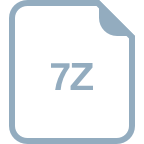
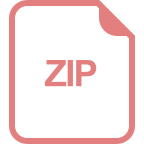
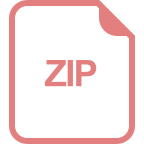
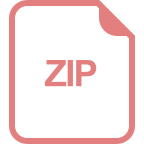
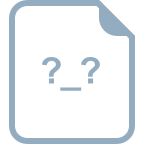
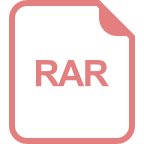
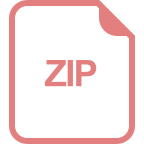