Angular2.0中组件的使用
发布时间: 2023-12-16 16:55:41 阅读量: 38 订阅数: 38 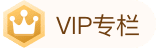
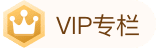
# 1. 简介
## 1.1 什么是Angular2.0
Angular2.0是一种用于构建Web应用程序的开发框架。它是AngularJS框架的升级版本,由Google开发和维护。与AngularJS相比,Angular2.0具有更高的性能、更好的可维护性和更强大的功能。
Angular2.0采用了组件化的架构,将应用程序拆分为多个独立的组件,每个组件都有自己的模板、样式和逻辑。组件可以嵌套使用,从而构建出复杂的应用程序。
## 1.2 什么是组件
在Angular2.0中,组件是构成应用程序的基本单元。一个组件由三部分组成:
- 模板(Template):定义了组件的外观和布局,使用HTML语法来描述。
- 类(Class):包含了组件的逻辑和数据,使用TypeScript或JavaScript来实现。
- 样式(Style):定义了组件的样式和外观,使用CSS语法来描述。
### 2. 组件的创建
在Angular中,组件是构成应用程序界面的基本构建块。一个组件由组件类、模板和装饰器元数据组成。在本章节中,我们将讨论如何使用Angular CLI创建组件以及组件的结构和文件组织。
#### 2.1 使用Angular CLI创建组件
Angular CLI是一个命令行工具,可以帮助我们快速搭建Angular应用程序的骨架,并且可以轻松地创建新的组件。假设我们已经安装了Angular CLI,下面是创建一个新组件的步骤:
首先,打开命令行工具,然后进入到你的项目目录中。
然后运行以下命令来创建一个新的组件:
```bash
ng generate component my-component
```
这条命令会在项目中的`src/app`目录下创建一个名为`my-component`的新组件,同时也会生成该组件所需的文件,包括组件类文件(`my-component.component.ts`)、模板文件(`my-component.component.html`)、样式文件(`my-component.component.css`)以及测试文件。
#### 2.2 组件的结构和文件组织
当我们使用Angular CLI创建组件后,会得到如下所示的文件结构:
```
src/
|-- app/
|-- my-component/
|-- my-component.component.ts
|-- my-component.component.html
|-- my-component.component.css
|-- my-component.component.spec.ts
```
- `my-component.component.ts`:该文件包含了组件的类定义,包括组件的属性和方法。
- `my-component.component.html`:组件的模板文件,用于定义组件的视图结构。
- `my-component.component.css`:组件的样式文件,用于定义组件的样式。
- `my-component.component.spec.ts`:组件的单元测试文件,用于测试组件的行为是否符合预期。
在项目中合理组织和命名这些文件是非常重要的,这样可以方便团队成员协作和维护代码。
### 3. 组件的属性与数据绑定
在Angular中,组件之间的数据传递和交互是非常重要的。组件的属性和数据绑定机制可以帮助我们实现这一目标。本节将介绍组件的属性绑定、事件绑定以及双向数据绑定的用法和示例。
#### 3.1 属性绑定
属性绑定是指将组件中的属性绑定到模板中的表达式上,实现动态数据的展示。在模板中使用方括号`[]`将属性绑定到组件中的属性。例如:
```javascript
// 组件中的属性
export class ProductComponent {
productName: string = '手机';
}
// 模板中的属性绑定
<p>产品名称:{{productName}}</p>
```
#### 3.2 事件绑定
事件绑定用于捕获模板中的事件,并执行组件中的方法。在模板中使用小括号`()`将事件绑定到组件中的方法。例如:
```javascript
// 组件中的方法
export class ProductComponent {
onBuyButtonClick() {
console.log('购买按钮被点击');
}
}
// 模板中的事件绑定
<button (click)="onBuyButtonClick()">购买</button>
```
#### 3.3 双向数据绑定
双向数据绑定允许数据在模型和视图之间进行双向传播。在Angular中,可以使用`[(ngModel)]`指令来实现双向数据绑定。首先需要导入`FormsModule`模块,然后在模板中使用`[(ngModel)]`指令。例如:
```javascript
// 导入FormsModule模块
import { FormsModule } from '@angular/forms';
// 组件中的属性
export class ProductComponent {
productName: string = '手机';
}
// 模板中的双向数据绑定
<input type="text" [(ngModel)]="productName">
<p>产品名称:{{productName}}</p>
```
通过属性绑定、事件绑定和双向数据绑定,我们可以实现组件内部属性和模板之间的数据交互,从而实现动态的用户界面交互效果。
### 4. 组件的生命周期
在Angular中,每个组件都有自己的生命周期,通过生命周期钩子函数可以控制组件在不同阶段的行为。了解组件的生命周期对于理解组件的工作方式和优化性能非常重要。
#### 4.1 组件的生命周期钩子函数
Angular组件的生命周期由一系列钩子函数来管理,它们可以让我们在组件生命周期的不同阶段执行逻辑。下面是一些常用的生命周期钩子函数:
- `ngOnChanges()`: 当组件的输入属性发生变化时调用,首次调用发生在`ngOnInit()`之前。
- `ngOnInit()`: 当组件初始化时调用,通常用来进行组件的初始化操作。
- `ngDoCheck()`: 在Angular自行运行的变更检测之后调用,可以手动检测和处理变更。
- `ngAfterContentInit()`: 当组件内容投影(ng-content)完成后调用。
- `ngAfterContentChecked()`: 在Angular检查投影内容时调用。
- `ngAfterViewInit()`: 当组件的视图初始化完成后调用。
- `ngAfterViewChecked()`: 在Angular检查视图变更时调用。
- `ngOnDestroy()`: 在组件销毁之前调用,用来进行一些清理操作。
#### 4.2 生命周期示例
以下是一个简单的示例,演示了组件的生命周期钩子函数的调用顺序:
```javascript
import { Component, OnInit, OnChanges, OnDestroy } from '@angular/core';
@Component({
selector: 'lifecycle-example',
template: `
<div>组件的生命周期示例</div>
`
})
export class LifecycleExampleComponent implements OnInit, OnChanges, OnDestroy {
constructor() {
console.log('constructor');
}
ngOnChanges() {
console.log('ngOnChanges');
}
ngOnInit() {
console.log('ngOnInit');
}
ngDoCheck() {
console.log('ngDoCheck');
}
ngAfterContentInit() {
console.log('ngAfterContentInit');
}
ngAfterContentChecked() {
console.log('ngAfterContentChecked');
}
ngAfterViewInit() {
console.log('ngAfterViewInit');
}
ngAfterViewChecked() {
console.log('ngAfterViewChecked');
}
ngOnDestroy() {
console.log('ngOnDestroy');
}
}
```
在控制台中,你将看到输出顺序为:
```
constructor
ngOnChanges
ngOnInit
ngAfterContentInit
ngAfterContentChecked
ngAfterViewInit
ngAfterViewChecked
ngDoCheck
```
这个示例展示了组件生命周期钩子函数的调用顺序,帮助你更好地理解各个生命周期阶段的作用。
### 5. 组件之间的通信
在Angular中,组件之间的通信是非常常见且重要的。组件之间的通信可以通过多种方式实现,比如父子组件之间的通信、兄弟组件之间的通信,以及通过服务实现组件通信。下面我们将分别介绍这些内容。
#### 5.1 父子组件之间的通信
在Angular中,父子组件之间的通信通常是通过输入属性和输出属性来实现的。父组件可以通过输入属性向子组件传递数据,而子组件可以通过输出属性向父组件发送事件。让我们通过一个简单的例子来演示父子组件之间的通信:
父组件 `parent-component`:
```typescript
import { Component } from '@angular/core';
@Component({
selector: 'app-parent-component',
template: `
<h2>Parent Component</h2>
<app-child-component [message]="parentMessage" (messageEvent)="receiveMessage($event)"></app-child-component>
`
})
export class ParentComponent {
parentMessage = "Message from parent";
receiveMessage($event: any) {
console.log($event);
}
}
```
子组件 `child-component`:
```typescript
import { Component, Input, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-child-component',
template: `
<h2>Child Component</h2>
<p>{{ message }}</p>
<button (click)="sendMessage()">Send Message to Parent</button>
`
})
export class ChildComponent {
@Input() message: string;
@Output() messageEvent = new EventEmitter<string>();
sendMessage() {
this.messageEvent.emit("Message from child");
}
}
```
在上面的例子中,父组件通过输入属性 `message` 向子组件传递数据,子组件通过输出属性 `messageEvent` 向父组件发送事件。
#### 5.2 兄弟组件之间的通信
在Angular中,兄弟组件之间的通信可以通过共享一个服务来实现。兄弟组件分别注入这个服务,在需要通信时,通过这个服务进行数据交换。让我们通过一个简单的例子来演示兄弟组件之间的通信:
定义一个共享的服务 `shared-service`:
```typescript
import { Injectable } from '@angular/core';
import { Subject } from 'rxjs';
@Injectable()
export class SharedService {
private messageSource = new Subject<string>();
message$ = this.messageSource.asObservable();
sendMessage(message: string) {
this.messageSource.next(message);
}
}
```
兄弟组件 `sibling-one`:
```typescript
import { Component } from '@angular/core';
import { SharedService } from './shared-service';
@Component({
selector: 'app-sibling-one',
template: `
<h2>Sibling One Component</h2>
<button (click)="sendMessageToSiblingTwo()">Send Message to Sibling Two</button>
`
})
export class SiblingOneComponent {
constructor(private sharedService: SharedService) {}
sendMessageToSiblingTwo() {
this.sharedService.sendMessage("Message from sibling one");
}
}
```
兄弟组件 `sibling-two`:
```typescript
import { Component, OnDestroy } from '@angular/core';
import { Subscription } from 'rxjs';
import { SharedService } from './shared-service';
@Component({
selector: 'app-sibling-two',
template: `
<h2>Sibling Two Component</h2>
<p>{{ message }}</p>
`
})
export class SiblingTwoComponent implements OnDestroy {
message: string;
subscription: Subscription;
constructor(private sharedService: SharedService) {
this.subscription = this.sharedService.message$.subscribe(message => {
this.message = message;
});
}
ngOnDestroy() {
this.subscription.unsubscribe();
}
}
```
在上面的例子中,`shared-service` 是一个共享的服务,`sibling-one` 和 `sibling-two` 分别注入这个服务,在需要通信时,通过 `shared-service` 来发送和接收消息。
#### 5.3 通过服务实现组件通信
除了兄弟组件之间通过共享服务进行通信外,其他组件之间的通信也可以通过共享服务来实现。通过共享服务,可以将数据共享给整个应用中的任何组件。
以上是组件之间通信的一些常见方式,根据实际情况选择合适的方式来实现组件之间的通信,这对于组件化开发和代码复用非常重要。
### 6. 组件的动态加载与路由
在Angular中,动态加载组件和路由是非常常见的需求。通过动态加载组件和路由,我们可以实现按需加载、延迟加载部分功能模块,从而提高应用的性能和用户体验。在本章节中,我们将学习如何在Angular中实现组件的动态加载和路由配置。
#### 6.1 懒加载组件
懒加载是指在用户访问特定路由时才加载相关的组件和模块,而不是在应用初始化时一次性加载所有组件和模块。这样可以减小应用的初始加载体积,提高应用的加载速度。要实现懒加载,我们可以使用Angular提供的路由配置。
```typescript
// app-routing.module.ts
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
const routes: Routes = [
{ path: 'home', loadChildren: () => import('./home/home.module').then(m => m.HomeModule) },
{ path: 'settings', loadChildren: () => import('./settings/settings.module').then(m => m.SettingsModule) },
// ... other routes
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
```
在以上代码中,通过`loadChildren`属性配合`import()`函数实现了懒加载。当用户访问`home`路由时,才会动态加载`HomeModule`模块;当用户访问`settings`路由时,才会动态加载`SettingsModule`模块。
#### 6.2 动态路由配置
除了懒加载组件外,有时候我们还需要根据特定条件动态配置路由。比如根据用户角色不同,显示不同的功能模块。在Angular中,我们可以通过编程方式动态配置路由。
```typescript
// app.component.ts
import { Component, OnInit } from '@angular/core';
import { Router, ActivatedRoute, Routes, RouterModule } from '@angular/router';
@Component({
selector: 'app-root',
template: '<router-outlet></router-outlet>'
})
export class AppComponent implements OnInit {
constructor(private router: Router, private route: ActivatedRoute) {}
ngOnInit() {
// 根据用户权限动态配置路由
if (user.isAdmin) {
this.router.resetConfig([
{ path: 'admin', loadChildren: () => import('./admin/admin.module').then(m => m.AdminModule) },
// ... other admin routes
]);
} else {
this.router.resetConfig([
{ path: 'user', loadChildren: () => import('./user/user.module').then(m => m.UserModule) },
// ... other user routes
]);
}
}
}
```
在以上代码中,通过调用`router.resetConfig()`方法,根据不同的条件动态配置了路由。当`user.isAdmin`为真时,加载了管理员相关的路由模块;当`user.isAdmin`为假时,加载了普通用户相关的路由模块。
#### 6.3 嵌套路由
在Angular中,我们还可以实现嵌套路由,即在一个组件中嵌套另一个组件的路由。这样可以更好地组织和管理页面结构,提高代码的可维护性。
```typescript
// main.component.html
<router-outlet></router-outlet>
// main-routing.module.ts
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { MainComponent } from './main.component';
import { DashboardComponent } from './dashboard/dashboard.component';
import { ProfileComponent } from './profile/profile.component';
const routes: Routes = [
{
path: 'main',
component: MainComponent,
children: [
{ path: 'dashboard', component: DashboardComponent },
{ path: 'profile', component: ProfileComponent }
]
}
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class MainRoutingModule { }
```
在以上代码中,`MainComponent`是一个包含子路由的父组件,通过`children`属性配置了嵌套路由。当用户访问`/main/dashboard`时,会加载`DashboardComponent`组件;当用户访问`/main/profile`时,会加载`ProfileComponent`组件。
0
0
相关推荐
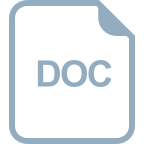
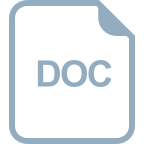
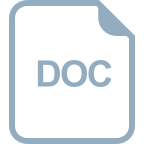
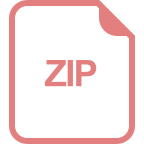
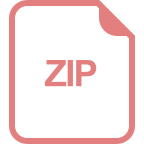
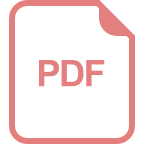
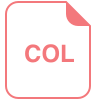
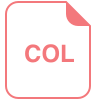
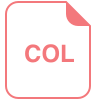