OpenCV C++图像边缘检测技术:提取图像边缘,勾勒图像轮廓,增强视觉效果
发布时间: 2024-08-05 20:39:58 阅读量: 14 订阅数: 17 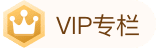
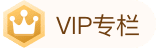
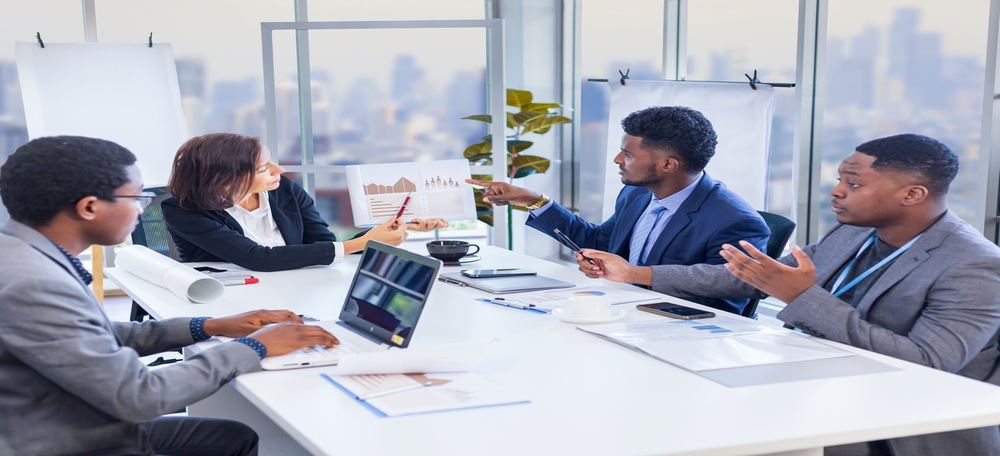
# 1. OpenCV C++图像边缘检测概述
图像边缘检测是计算机视觉中一项重要的技术,它可以从图像中提取有意义的特征,为后续的图像分析和处理提供基础。OpenCV(Open Source Computer Vision Library)是一个功能强大的开源计算机视觉库,它提供了丰富的图像边缘检测函数,使开发者能够轻松实现各种边缘检测算法。
本章将对OpenCV C++图像边缘检测进行概述,包括其基本概念、算法原理和OpenCV中的相关函数介绍。通过理解这些基础知识,开发者可以为图像处理和分析任务选择合适的边缘检测算法,并使用OpenCV高效地实现它们。
# 2. 图像边缘检测理论基础
### 2.1 图像边缘的概念和意义
图像边缘是图像中相邻像素之间灰度值发生显著变化的区域,它代表了图像中不同物体或区域之间的边界。边缘检测是计算机视觉中一项基本任务,其目的是从图像中提取这些边缘,以供进一步处理和分析。
边缘在图像理解中具有重要意义。它可以帮助我们:
- **分割图像:**将图像分割成不同的区域或对象。
- **轮廓提取:**提取图像中对象的轮廓。
- **目标识别:**识别图像中的特定对象。
- **运动检测:**检测图像中运动的物体。
### 2.2 图像边缘检测算法原理
图像边缘检测算法通过分析图像像素之间的灰度值差异来检测边缘。常见的边缘检测算法包括:
#### 2.2.1 梯度法
梯度法利用图像中相邻像素的灰度值差异来检测边缘。它计算每个像素的梯度,即灰度值在水平和垂直方向上的变化率。梯度值较大的像素表示边缘像素。
**Sobel 算子**和 **Prewitt 算子**是梯度法中常用的算子。它们使用 3x3 的卷积核对图像进行卷积,以计算每个像素的梯度。
#### 2.2.2 拉普拉斯算子法
拉普拉斯算子法使用拉普拉斯算子对图像进行卷积,以检测边缘。拉普拉斯算子是一个 3x3 的算子,其中心值为 0,周围值为 1。
拉普拉斯算子法对噪声敏感,因此需要在使用前对图像进行平滑处理。
#### 2.2.3 Canny 边缘检测算法
Canny 边缘检测算法是一种多阶段边缘检测算法,它结合了梯度法和阈值化技术。Canny 算法的步骤如下:
1. 使用高斯滤波器平滑图像。
2. 计算图像的梯度。
3. 对梯度进行非极大值抑制,以消除边缘上的杂散像素。
4. 使用双阈值化技术,将梯度值低于高阈值的像素抑制为背景,将梯度值高于低阈值的像素检测为边缘。
5. 使用滞后阈值化技术,连接低阈值像素和高阈值像素,形成完整的边缘。
Canny 算法是一种鲁棒且有效的边缘检测算法,它广泛应用于计算机视觉领域。
# 3. OpenCV C++图像边缘检测实践
### 3.1 OpenCV图像边缘检测函数介绍
OpenCV C++库提供了丰富的图像边缘检测函数,包括:
- `Canny()`:Canny边缘检测算法
- `Sobel()`:Sobel算子边缘检测
- `Laplacian()`:拉普拉斯算子边缘检测
- `Scharr()`:Scharr算子边缘检测
- `Prewitt()`:Prewitt算子边缘检测
### 3.2 图像边缘检测代码实现
#### 3.2.1 梯度法边缘检测
梯度法边缘检测使用Sobel算子或Scharr算子计算图像像素的梯度,然后根据梯度大小和方向确定边缘点。
```cpp
// Sobel算子边缘检测
cv::Mat sobel_x, sobel_y;
cv::Sobel(input_image, sobel_x, CV_16S, 1, 0, 3);
cv::Sobel(input_image, sobel_y, CV_16S, 0, 1, 3);
cv::convertScaleAbs(sobel_x, sobel_x);
cv::convertScaleAbs(sobel_y, sobel_y);
cv::addWeighted(sobel_x, 0.5, sobel_y, 0.5, 0, edge_image);
// Scharr算子边缘检测
cv::Mat scharr_x, scharr_y;
cv::Scharr(input_image, scharr_x, CV_16S, 1, 0);
cv::Scharr(input_image, scharr_y, CV_16S, 0, 1);
cv::convertScaleAbs(scharr_x, scharr_x);
cv::convertScaleAbs(scharr_y, scharr_y);
cv::addWeighted(scharr_x, 0.5, scharr_y, 0.5, 0, edge_image);
```
**参数说明:**
- `input_image`:输入图像
- `edge_image`:输出边缘图像
- `sobel_x`、`sobel_y`、`scharr_x`、`scharr_y`:梯度图像
- `1`、`0`:梯度计算方向(x方向或y方向)
- `3`:卷积核大小
- `0.5`:权重系数
**代码逻辑分析:**
1. 使用Sobel算子或Scharr算子计算图像的x方向和y方向梯度。
2. 将梯度转换为绝对值并归一化到0-255范围。
3. 将x方向和y方向梯度加权平均得到边缘图像。
#### 3.2.2 拉普拉斯算子法边缘检测
拉普拉斯算子法使用拉普拉
0
0
相关推荐
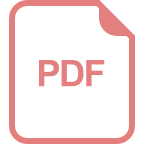
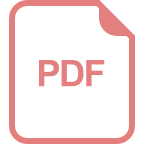
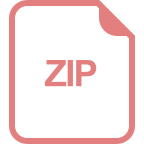





