Python性能优化秘籍:提升代码效率,应对复杂场景
发布时间: 2024-06-20 21:08:55 阅读量: 66 订阅数: 28 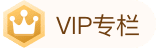
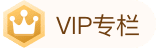
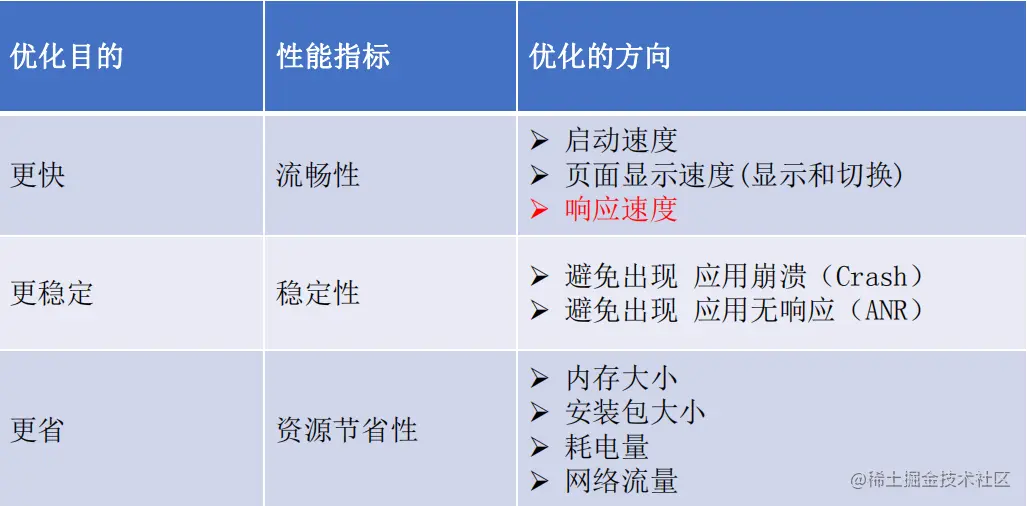
# 1. Python性能优化概述
Python是一种解释型语言,其性能优化至关重要。本章将概述Python性能优化,探讨其重要性、常见挑战和优化策略。
### 1.1 Python性能优化的重要性
* 提升用户体验和应用程序响应速度
* 降低服务器成本和资源消耗
* 提高应用程序的可扩展性和可靠性
### 1.2 Python性能优化面临的挑战
* 解释型语言的固有开销
* 数据结构和算法选择不当
* 代码结构和设计不佳
* 并发和并行编程的复杂性
* 虚拟机和解释器限制
# 2. Python性能分析和度量
### 2.1 性能分析工具和方法
#### 2.1.1 Python内置的性能分析工具
Python提供了内置的性能分析工具,如:
- `timeit`:用于测量代码片段的执行时间。
- `cProfile`:用于分析代码的函数调用和时间消耗。
- `memory_profiler`:用于分析代码的内存使用情况。
**代码块:**
```python
import timeit
def fibonacci(n):
if n < 2:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
# 测量fibonacci函数在输入n=30时的执行时间
time = timeit.timeit("fibonacci(30)", number=1000)
print(f"Fibonacci(30)执行时间:{time}秒")
```
**逻辑分析:**
`timeit`模块的`timeit()`函数用于测量代码片段的执行时间。该函数接收两个参数:要测量的代码片段和重复执行的次数。
在上面的代码中,`fibonacci(30)`代码片段被执行了1000次,并记录了总执行时间。
#### 2.1.2 第三方性能分析工具
除了内置工具外,还有许多第三方性能分析工具可供使用,如:
- `Pyinstrument`:用于分析代码的执行时间和内存使用情况。
- `Snakeviz`:用于可视化代码的性能数据。
- `Heapy`:用于分析代码的内存使用情况。
### 2.2 性能度量指标
#### 2.2.1 时间复杂度和空间复杂度
时间复杂度和空间复杂度是衡量算法性能的重要指标。
- **时间复杂度**:衡量算法执行所花费的时间。
- **空间复杂度**:衡量算法执行所需的内存空间。
**表格:常见算法的时间复杂度和空间复杂度**
| 算法 | 时间复杂度 | 空间复杂度 |
|---|---|---|
| 顺序搜索 | O(n) | O(1) |
| 二分搜索 | O(log n) | O(1) |
| 冒泡排序 | O(n^2) | O(1) |
| 快速排序 | O(n log n) | O(log n) |
| 哈希表 | O(1) | O(n) |
#### 2.2.2 内存使用和CPU利用率
内存使用和CPU利用率是衡量系统性能的两个重要指标。
- **内存使用**:衡量系统中正在使用的内存量。
- **CPU利用率**:衡量CPU正在使用的时间百分比。
**代码块:**
```python
import psutil
# 获取当前内存使用情况
memory_usage = psutil.virtual_memory()
print(f"内存使用:{memory_usage.percent}%")
# 获取当前CPU利用率
cpu_usage = psutil.cpu_percent()
print(f"CPU利用率:{cpu_usage}%")
```
**逻辑分析:**
`psutil`模块提供了用于获取系统性能信息的函数。
在上面的代码中,`psutil.virtual_memory()`函数用于获取当前内存使用情况,`psutil.cpu_percent()`函数用于获取当前CPU利用率。
# 3.1 数据结
0
0
相关推荐
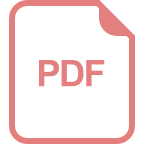
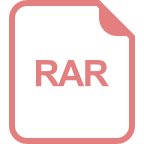
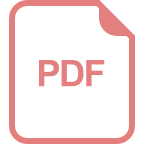
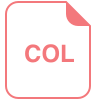
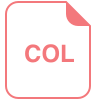
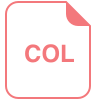
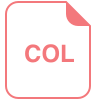
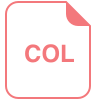
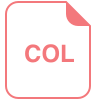