初步了解Angular 4中的组件和模块
发布时间: 2023-12-16 06:44:59 阅读量: 33 订阅数: 32 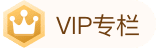
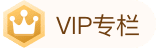
# 1. 介绍Angular 4及其主要特点
## 1.1 Angular 4是什么?
## 1.2 Angular 4的主要特点有哪些?
## 2. 理解Angular 4中的组件概念
组件是Angular中最基本的构建块之一,它负责处理应用程序的不同部分,并将其封装在一个可复用的单元中。在此章节中,我们将详细介绍什么是组件,并探讨Angular 4中的组件如何定义和使用,以及组件的生命周期钩子函数的作用。
### 2.1 什么是组件?
组件是由模板、样式和逻辑代码构成的整体单位。它们通常代表一个特定的视图,用于显示数据并处理用户交互。组件可以嵌套在彼此之中,形成层次结构,从而构建出复杂的用户界面。
### 2.2 Angular 4中的组件如何定义和使用?
在Angular 4中,我们可以使用`@Component`装饰器来定义一个组件。这个装饰器告诉Angular该如何处理组件,包括它的模板、样式以及其它配置项。
以下是一个简单的组件定义示例:
```typescript
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent {
// 组件逻辑代码
}
```
在定义组件时,我们需要指定一个选择器(selector),它用于将组件嵌入到HTML中的指定位置。模板(templateUrl)用于定义组件的视图,而样式(styleUrls)则用于指定组件的样式表。
要在应用程序中使用组件,只需在需要的位置使用选择器即可,比如:
```html
<app-example></app-example>
```
### 2.3 组件的生命周期钩子函数是什么?
组件生命周期钩子函数是由Angular提供的一些回调函数,它们在组件不同的生命周期阶段被调用,以便我们可以在特定的时间点执行一些逻辑操作。
以下是一些常用的生命周期钩子函数:
- `ngOnInit`:在组件初始化完成后调用,用于初始化数据或进行一些逻辑操作。
- `ngOnChanges`:在组件的输入属性发生变化时调用,用于响应属性的变化。
- `ngDoCheck`:检测组件的变化,它会在每个变化检测周期中调用。
- `ngAfterViewInit`:在组件的视图初始化完成后调用,用于操作DOM或调用第三方库。
- `ngOnDestroy`:在组件销毁之前调用,用于清理资源或取消订阅。
通过正确使用这些生命周期钩子函数,我们可以更好地控制组件的行为,并在不同的阶段执行必要的逻辑操作。
### 3. 深入研究Angular 4中的模块
在Angular 4中,模块是一个可复用的代码块,用于将一组相关的组件、指令、管道和服务组织在一起。它帮助我们将应用程序划分为功能模块,使代码更加可维护和可扩展。
#### 3.1 什么是模块?
模块是Angular中的一个关键概念,它用来封装一系列相关的功能并提供这些功能的导出接口。一个模块通常包含组件、指令、管道和服务等。
#### 3.2 Angular 4中的模块有哪些作用?
模块在Angular中的作用主要有以下几个方面:
- 将相关的功能组织在一起,提高代码的可维护性;
- 实现代码的复用和模块化,提高开发效率;
- 提供了模块间的边界,可以避免命名冲突和全局变量污染;
- 支持懒加载,提高应用的加载速度。
#### 3.3 如何创建和管理模块?
在Angular中,可以使用Angular CLI来创建新的模块。以下是创建模块的步骤:
1. 打开命令行工具并导航到项目目录;
2. 运行以下命令来生成一个新的模块:
```
ng generate module module-name
```
其中,module-name是你自定义的模块名称。
3. Angular CLI会自动在项目目录下生成一个新的模块文件,并在app.module.ts文件中将其添加到项目的根模块中。
在模块中,我们可以使用`@NgModule`装饰器来定义模块的元数据,包括模块所依赖的其他模块、声明的组件、指令和管道、服务等。以下是一个模块的示例:
```typescript
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { MyComponent } from './my.component';
import { MyDirective } from './my.directive';
import { MyPipe } from './my.pipe';
import { MyService } from './my.service';
@NgModule({
imports: [
CommonModule
],
declarations: [
MyComponent,
MyDirective,
MyPipe
],
providers: [
MyService
],
exports: [
MyComponent
]
})
export class MyModule { }
```
在这个示例中,我们使用`@NgModule`装饰器定义了一个模块。模块中引入了`CommonModule`,声明了`MyComponent`、`MyDirective`和`MyPipe`,提供了`MyService`,并将`MyComponent`导出以供其他组件使用。
# 4. 组件间的通信方式
在Angular中,组件是构建应用程序的基本单元,组件之间的通信是非常重要的。在这一章节中,我们将介绍几种常见的组件间通信方式。
## 4.1 父子组件之间的通信
在Angular中,父组件可以通过属性绑定向子组件传递数据,而子组件可以通过`@Input()`装饰器接收父组件传递的数据。下面是一个示例:
```typescript
// parent.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-parent',
template: `
<app-child [message]="message"></app-child>
`
})
export class ParentComponent {
message = 'Hello from parent';
}
// child.component.ts
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-child',
template: `
<p>{{ message }}</p>
`
})
export class ChildComponent {
@Input() message: string;
}
```
父组件通过`[message]="message"`将`message`属性绑定到子组件的`message`输入属性上。子组件则可以在模板中使用`message`来显示对应的值。
## 4.2 兄弟组件之间的通信
如果两个组件之间没有直接的父子关系,我们可以使用一个共享的服务来实现兄弟组件之间的通信。下面是一个示例:
```typescript
// data.service.ts
import { Injectable } from '@angular/core';
import { Subject } from 'rxjs';
@Injectable()
export class DataService {
private messageSource = new Subject<string>();
message$ = this.messageSource.asObservable();
sendMessage(message: string) {
this.messageSource.next(message);
}
}
// sibling.component.ts
import { Component } from '@angular/core';
import { DataService } from 'data.service';
@Component({
selector: 'app-sibling-a',
template: `
<button (click)="sendMessage()">Send Message</button>
`
})
export class SiblingAComponent {
constructor(private dataService: DataService) {}
sendMessage() {
this.dataService.sendMessage('Hello from sibling A');
}
}
@Component({
selector: 'app-sibling-b',
template: `
<p>{{ message }}</p>
`
})
export class SiblingBComponent {
message: string;
constructor(private dataService: DataService) {}
ngOnInit() {
this.dataService.message$.subscribe(message => {
this.message = message;
});
}
}
```
在上面的例子中,我们定义了一个名为`DataService`的共享服务,它通过一个`Subject`来保存消息,并将其暴露为`message$`可观察对象。`SiblingAComponent`和`SiblingBComponent`分别是兄弟组件,它们都依赖于`DataService`服务。`SiblingAComponent`通过调用`sendMessage`方法向`DataService`发送消息,而`SiblingBComponent`通过订阅`message$`可观察对象来接收消息并显示。
## 4.3 使用服务进行组件间的通信
除了上面介绍的父子组件和兄弟组件之间的通信方式外,我们还可以使用服务来实现组件间的通信。服务是Angular应用中的可复用代码块,可以在多个组件之间共享数据和逻辑。
下面是一个使用服务进行组件间通信的示例:
```typescript
// message.service.ts
import { Injectable } from '@angular/core';
@Injectable()
export class MessageService {
private message: string;
setMessage(message: string) {
this.message = message;
}
getMessage(): string {
return this.message;
}
}
// component-a.component.ts
import { Component } from '@angular/core';
import { MessageService } from './message.service';
@Component({
selector: 'app-component-a',
template: `
<p>{{ message }}</p>
<input [(ngModel)]="inputMessage" placeholder="Enter message">
<button (click)="sendMessage()">Send Message</button>
`,
})
export class ComponentAComponent {
message: string;
inputMessage: string;
constructor(private messageService: MessageService) {}
sendMessage() {
this.messageService.setMessage(this.inputMessage);
}
ngOnInit() {
this.message = this.messageService.getMessage();
}
}
// component-b.component.ts
import { Component } from '@angular/core';
import { MessageService } from './message.service';
@Component({
selector: 'app-component-b',
template: `
<p>{{ message }}</p>
`,
})
export class ComponentBComponent {
message: string;
constructor(private messageService: MessageService) {}
ngOnInit() {
this.message = this.messageService.getMessage();
}
}
```
在这个例子中,我们创建了一个名为`MessageService`的服务,它有两个方法:`setMessage`用于设置消息,`getMessage`用于获取消息。`ComponentAComponent`通过调用`setMessage`方法设置消息,而`ComponentBComponent`通过调用`getMessage`方法获取消息并显示。
以上是几种常见的组件间通信方式,在实际应用中,根据具体的业务需求,我们可以选择最适合的方式进行组件间的通信。
### 5. 模块的加载和懒加载
在Angular 4中,模块的加载是非常重要的,它决定了应用程序的性能和用户体验。了解模块的加载方式以及懒加载的优势是开发Angular应用程序的关键。
#### 5.1 如何加载模块?
在Angular中,模块可以通过根模块(AppModule)或特性模块的方式进行加载。根模块负责引导应用程序,而特性模块则用于组织代码、提供服务和管理组件。
下面是一个简单的模块加载示例:
```typescript
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
imports: [BrowserModule],
declarations: [AppComponent],
bootstrap: [AppComponent]
})
export class AppModule { }
```
#### 5.2 什么是懒加载?
懒加载是指在应用程序运行过程中动态加载模块的机制。这意味着当用户访问特定路由时,才会加载相关模块,而不是在应用启动时一次性加载所有模块。
懒加载有助于减少初始加载时间,并且可以根据用户的实际需求按需加载模块,从而提升应用性能和用户体验。
#### 5.3 懒加载的优势和使用场景
懒加载的优势包括:
- 减少初始加载时间,加快应用程序启动速度
- 按需加载模块,减轻了应用的初始化负担
- 优化用户体验,提升页面渲染速度
使用场景包括:
- 对于大型应用程序,可以通过懒加载减小初始加载体积
- 对于部分功能模块较少使用的应用,可以通过懒加载节省资源
模块的加载和懒加载是Angular应用程序优化的重要手段,合理的模块组织和加载策略可以极大地提升应用的性能。
### 6. 总结与展望
在本文中,我们对Angular 4中的组件和模块进行了初步的了解。我们首先介绍了Angular 4以及它的主要特点,包括强大的组件化和模块化架构。
接着,我们深入研究了Angular 4中的组件概念。我们了解了组件的定义和使用方式,还介绍了组件的生命周期钩子函数的作用。
然后,我们着重讨论了Angular 4中的模块。我们解释了模块的概念和作用,并演示了如何创建和管理模块。
接下来,我们详细介绍了组件间的通信方式。我们讨论了父子组件之间、兄弟组件之间以及使用服务进行组件间通信的方法。
此外,我们还学习了模块的加载和懒加载的概念。我们探讨了模块加载的方式,以及懒加载的优势和使用场景。
最后,我们对本文进行了总结和展望。我们强调了组件和模块在Angular中的重要性,并展望了未来Angular版本中组件和模块的发展趋势。
0
0
相关推荐
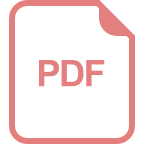
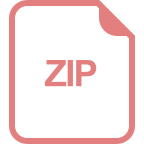
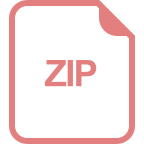
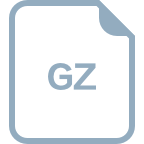
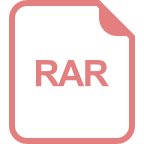
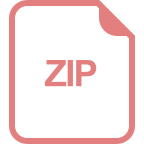