I_O流操作:Java中文件读写和网络通信
发布时间: 2024-01-13 00:01:00 阅读量: 35 订阅数: 31 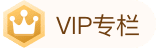
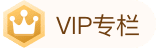
# 1. 简介
## 1.1 什么是I/O流操作
I/O流操作是指输入/输出流操作,是计算机程序与外部世界进行数据交换的一种方式。在Java中,I/O流操作主要用于文件读写和网络通信等功能。
## 1.2 Java中的文件读写和网络通信
在Java中,可以通过文件读写和网络通信来实现数据的输入输出。文件读写操作可以通过File类进行文件操作,使用文件读取流(InputStream)和文件写入流(OutputStream)来读取和写入文件内容。同时,Java也提供了网络编程的功能,可以通过Socket等类来实现网络通信。
接下来,我们将分别介绍文件读写操作、字节流和字符流、缓冲流操作、网络通信以及序列化与反序列化等内容。
# 2. 文件读写操作
在这一节中,我们将介绍如何在Java中进行文件读写操作。文件操作是日常开发中常见的任务之一,了解如何使用Java进行文件读写操作对于开发人员来说十分重要。
#### 2.1 使用File类进行文件操作
首先,我们可以使用File类来进行文件的创建、删除、重命名等操作。下面是一个创建文件和文件夹的示例代码:
```java
import java.io.File;
public class FileOperationExample {
public static void main(String[] args) {
// 创建文件
File file = new File("test.txt");
try {
if (file.createNewFile()) {
System.out.println("File created: " + file.getName());
} else {
System.out.println("File already exists.");
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
// 创建文件夹
File dir = new File("testDir");
if (!dir.exists()) {
if (dir.mkdir()) {
System.out.println("Directory created: " + dir.getName());
} else {
System.out.println("Failed to create directory");
}
}
}
}
```
通过File类,我们可以轻松地进行文件和文件夹的操作。
#### 2.2 文件读取流(InputStream)的使用
文件读取流用于从文件中读取数据。InputStream是所有输入流的超类,我们可以使用FileInputStream来读取文件数据。以下是一个简单的文件读取示例:
```java
import java.io.FileInputStream;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
try (FileInputStream fis = new FileInputStream("test.txt")) {
int content;
while ((content = fis.read()) != -1) {
System.out.print((char) content);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上代码使用FileInputStream来读取文件内容,并且利用while循环和read()方法逐个读取文件中的字符。
#### 2.3 文件写入流(OutputStream)的使用
文件写入流用于向文件中写入数据。OutputStream是所有输出流的超类,我们可以使用FileOutputStream来进行文件写入操作。以下是一个简单的文件写入示例:
```java
import java.io.FileOutputStream;
import java.io.IOException;
public class FileWriteExample {
public static void main(String[] args) {
String content = "Hello, this is a file write example.";
try (FileOutputStream fos = new FileOutputStream("output.txt")) {
byte[] bytes = content.getBytes();
fos.write(bytes);
System.out.println("Content has been written to the file.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
通过以上示例,我们了解了如何使用FileInputStream和FileOutputStream来实现文件的读取和写入操作。在实际开发中,我们经常需要进行文件的复制和移动,下面将介绍相关操作。
#### 2.4 文件的复制和移动
对于文件复制操作,我们可以使用FileInputStream和FileOutputStream来实现,先将源文件内容读取到内存中,再写入到目标文件中。文件移动则可使用File类的renameTo()方法。
```java
import java.io.*;
public class FileCopyMoveExample {
public static void main(String[] args) {
// 文件复制
try (FileInputStream fis = new FileInputStream("source.txt");
FileOutputStream fos = new FileOutputStream("target.txt")) {
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
System.out.println("File has been copied successfully.");
} catch (IOException e) {
e.printStackTrace();
}
// 文件移动
File file = new File("source.txt");
File newFile = new File("movedFile.txt");
if (file.renameTo(newFile)) {
System.out.println("File has been moved successfully.");
} else {
System.out.println("Failed to move the file.");
}
}
}
```
通过以上示例,我们了解了如何使用Java进行文件的复制和移动操作。文件读写是Java中常见的操作之一,熟练掌握文件读
0
0
相关推荐
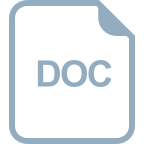
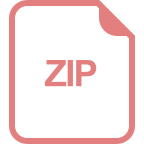
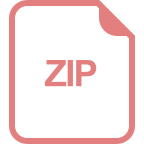
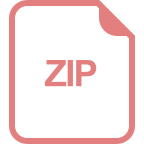
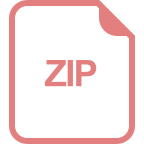
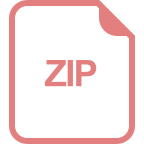
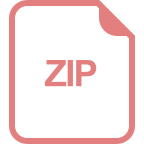