浮点数比较的雷区:避免意外结果的实用指南
发布时间: 2024-07-13 18:07:09 阅读量: 34 订阅数: 25 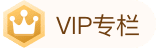
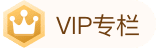
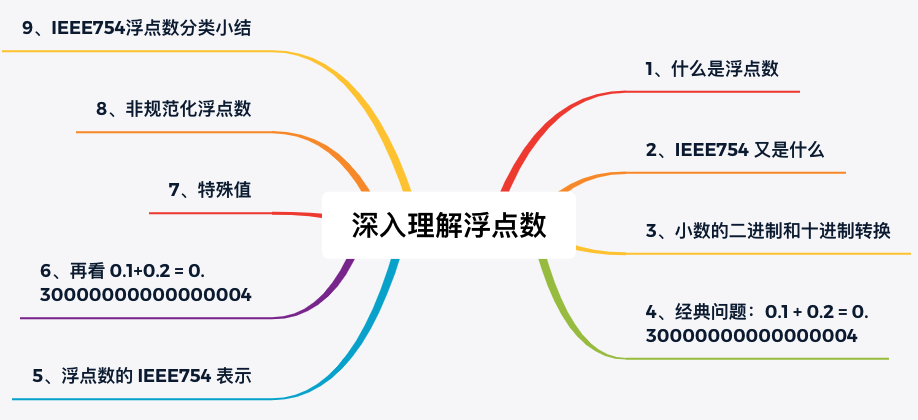
# 1. 浮点数比较的基础知识
浮点数是计算机中表示实数的一种数据类型,它使用科学记数法将数字表示为底数和指数的组合。由于其有限的精度,浮点数比较存在一些固有的挑战。
浮点数的比较通常使用比较操作符(如 `==`、`!=`、`<`、`>`)进行。然而,由于精度限制,这些操作符可能会产生意外结果。例如,两个浮点数可能在数学上相等,但在计算机中却可能被视为不相等。
# 2. 浮点数比较的常见误区
### 2.1 精度损失和舍入误差
浮点数的精度有限,在进行算术运算时会产生精度损失。例如,将两个浮点数相加或相减时,结果的精度可能低于操作数的精度。这是因为浮点数的尾数是有限的,而算术运算可能产生超出尾数范围的结果。
```python
a = 0.1
b = 0.2
c = a + b
print(c) # 输出:0.30000000000000004
```
在这个例子中,`a` 和 `b` 的尾数都是小数点后一位,但它们的和 `c` 的尾数却有 16 位。这是因为 `a + b` 的结果是一个无限循环小数,计算机将其舍入为 16 位浮点数。
### 2.2 NaN和无穷大的处理
NaN(Not a Number)和无穷大是浮点数系统中表示特殊值的特殊值。NaN 表示一个无效或未定义的值,而无穷大表示一个非常大的值。
比较浮点数时,需要特别注意 NaN 和无穷大。NaN 与任何其他值(包括自身)都不相等,而无穷大比任何其他值都大。因此,比较浮点数时,应首先检查它们是否为 NaN 或无穷大。
```python
import math
a = float('nan')
b = float('inf')
c = 1.0
print(a == a) # 输出:False
print(b > c) # 输出:True
```
### 2.3 比较操作符的局限性
浮点数比较操作符(==、!=、<、>、<=、>=)的语义与整数比较操作符不同。整数比较操作符只检查操作数的位模式,而浮点数比较操作符还考虑精度损失和舍入误差。
例如,以下代码会输出 `False`,即使 `a` 和 `b` 在数学上相等。
```python
a = 0.1
b = 0.10000000000000001
print(a == b) # 输出:False
```
这是因为 `a` 和 `b` 在二进制表示中不同,并且浮点数比较操作符会考虑这种差异。
为了避免浮点数比较的误差,应使用相对误差进行比较,而不是相等性比较。
# 3.1 使用相对误差进行比较
相对误差是一种衡量两个浮点数之间差异的度量,它通过将两个浮点数的差值除以它们的平均值来计算。相对误差的公式如下:
```
相对误差 = |(a - b) / ((a + b) / 2)|
```
其中:
* a 和 b 是要比较的两个浮点数
相对误差的一个优点是它不受浮点数精度的影响。这意味着即使两个浮点数的精度不同,也可以使用相对误差来比较它们。
使用相对误差进行比较的步骤如下:
1. 计算两个浮点数的差值。
2. 计算两个浮点数的平均值。
3. 将差值除以平均值。
4. 计算出的结果就是相对误差。
例如,要比较浮点数 1.0000000000000002 和 1.0000000000000001,可以使用以下步骤:
1. 计算差值:1.0000000000000002 - 1.0000000000000001 = 0.0000000000000001
2. 计算平均值:(1.0000000000000002 + 1.0000000000000001) / 2 = 1.00000000000000015
3. 计算相对误差:|0.0000000000000001 / 1.00000000000000015| = 0.0000000000000001
相对误差为 0.0000000000000001,表明这两个浮点数非常接近。
### 3.2 考虑舍入模式和精度
舍入模式和精度是影响浮点数比较的两个重要因素。舍入模式决定了当浮点数无法精确表示为二进制时如何舍入。精度决定了浮点数中可以表示的小数位数。
常见的舍入模式包括:
* **向最接近的偶数舍入(round-to-nearest-even):**这是默认的舍入模式,它将浮点数舍入到最接近的偶数。
* **向零舍入(round-to-zero):**它将浮点数舍入到最接近的整数。
* **向正无穷大舍入(round-to-positive-infinity):**它将浮点数舍入到最接近的正无穷大。
* **向负无穷大舍入(round-to-negative-infinity):**它将浮点数舍入到最接近的负无穷大。
精度决定了浮点数中可以表示的小数位数。精度越高,可以表示的小数位数就越多。
在比较浮点数时,需要考虑舍入模式和精度。例如,如果两个浮点数的精度不同,则它们可能无法精确比较。同样,如果舍入模式不同,则比较结果也可能不同。
### 3.3 避免使用相等性比较
在大多数情况下,避免使用相等性比较(==)来比较浮点数。这是因为浮点数的精度有限,因此两个浮点数可能在数学上相等,但在计算机中却不相等。
例如,以下代码将打印 False,即使两个浮点数在数学上相等:
```python
a = 0.1
b = 0.2
print(a == b) # False
```
这是因为计算机将 0.1 表示为二进制小数,该小数无法精确表示。因此,计算机存储的 a 的值实际上是 0.10000000000000001。当计算机比较 a 和 b 时,它将比较这两个二进制小数,发现它们不相等。
为了避免这个问题,可以使用相对误差或其他比较方法来比较浮点数。
# 4. 浮点数比较的进阶技术
### 4.1 使用浮点数比较库
对于需要处理复杂浮点数比较场景的应用,使用专门的浮点数比较库可以显著简化开发过程。这些库提供了预先构建的函数和算法,可以处理各种浮点数比较情况,包括:
- **相对误差比较:**使用相对误差阈值来确定两个浮点数是否相等。
- **舍入模式和精度控制:**允许指定舍入模式和精度,以确保比较结果符合特定要求。
- **NaN和无穷大处理:**提供专门的函数来处理NaN和无穷大值,确保比较操作的正确性。
一些流行的浮点数比较库包括:
- **Boost.Math:**一个C++库,提供广泛的数学函数,包括浮点数比较函数。
- **Intel Math Kernel Library (MKL):**一个高度优化的数学库,包含用于浮点数比较的特定函数。
- **GNU Scientific Library (GSL):**一个C库,提供各种科学和数学函数,包括浮点数比较函数。
### 4.2 探索硬件特定的比较指令
某些现代处理器提供了特定于硬件的比较指令,可以显著提高浮点数比较的性能。这些指令通常使用专门的硬件电路来执行比较操作,从而减少延迟和提高吞吐量。
例如,英特尔处理器提供了以下比较指令:
- **CMPPS:**比较两个打包的单精度浮点数。
- **CMPPD:**比较两个打包的双精度浮点数。
这些指令可以与汇编语言或内联汇编一起使用,以优化浮点数比较密集型代码的性能。
### 代码示例
以下代码示例演示了如何使用Boost.Math库进行相对误差比较:
```cpp
#include <boost/math/special_functions/fpclassify.hpp>
#include <boost/math/special_functions/ulp.hpp>
bool are_approximately_equal(float a, float b, float tolerance) {
if (boost::math::isnan(a) || boost::math::isnan(b)) {
return false;
}
if (boost::math::isinf(a) || boost::math::isinf(b)) {
return false;
}
float ulp_diff = boost::math::ulp(a) - boost::math::ulp(b);
return std::abs(ulp_diff) <= tolerance;
}
```
在该示例中,`are_approximately_equal`函数使用相对误差阈值`tolerance`来确定两个浮点数`a`和`b`是否相等。它考虑了NaN和无穷大值,并使用`ulp`函数来计算两个浮点数的单位最后一位(ulp)差异。如果ulp差异绝对值小于`tolerance`,则函数返回`true`,表示两个浮点数近似相等。
# 5. 浮点数比较的应用案例
浮点数比较在实际应用中至关重要,以下是一些常见场景:
### 5.1 金融计算中的精度要求
金融计算需要高度的精度,浮点数比较对于确保计算的准确性至关重要。例如,在计算复利时,浮点数比较用于确定是否达到预期的利率。如果比较操作符不准确,可能会导致错误的利率计算,从而影响投资收益。
### 5.2 科学计算中的舍入误差处理
科学计算通常涉及大量浮点数运算,舍入误差可能会累积并影响结果的准确性。浮点数比较用于监控舍入误差,并根据需要采取措施进行补偿。例如,在求解微分方程时,浮点数比较用于确定是否需要增加计算精度以减少舍入误差。
### 5.3 图形学中的无穷大处理
图形学中经常使用无穷大来表示无限远或无限小。浮点数比较用于处理无穷大值,例如,在透视投影中,浮点数比较用于确定物体是否位于视锥体之外,从而决定是否将其渲染。
0
0
相关推荐
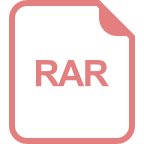
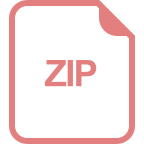
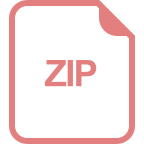





